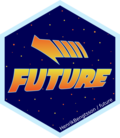
Back trace the expressions evaluated when an error was caught
Source:R/utils_api-backtrace.R
backtrace.Rd
Back trace the expressions evaluated when an error was caught
Usage
backtrace(future, envir = parent.frame(), ...)
Examples
my_log <- function(x) log(x)
foo <- function(...) my_log(...)
f <- future({ foo("a") })
res <- tryCatch({
v <- value(f)
}, error = function(ex) {
t <- backtrace(f)
print(t)
})
#> [[1]]
#> base::tryCatch(base::withCallingHandlers({
#> NULL
#> base::saveRDS(base::do.call(base::do.call, base::c(base::readRDS("/tmp/henrik/RtmpJraagA/callr-fun-101bce40f7699e"),
#> base::list(envir = .GlobalEnv, quote = TRUE)), envir = .GlobalEnv,
#> quote = TRUE), file = "/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> compress = FALSE)
#> base::flush(base::stdout())
#> base::flush(base::stderr())
#> NULL
#> base::invisible()
#> }, error = function(e) {
#> {
#> callr_data <- base::as.environment("tools:callr")$`__callr_data__`
#> err <- callr_data$err
#> if (FALSE) {
#> base::assign(".Traceback", base::.traceback(4), envir = callr_data)
#> utils::dump.frames("__callr_dump__")
#> base::assign(".Last.dump", .GlobalEnv$`__callr_dump__`,
#> envir = callr_data)
#> base::rm("__callr_dump__", envir = .GlobalEnv)
#> }
#> e <- err$process_call(e)
#> e2 <- err$new_error("error in callr subprocess")
#> class <- base::class
#> class(e2) <- base::c("callr_remote_error", class(e2))
#> e2 <- err$add_trace_back(e2)
#> cut <- base::which(e2$trace$scope == "global")[1]
#> if (!base::is.na(cut)) {
#> e2$trace <- e2$trace[-(1:cut), ]
#> }
#> base::saveRDS(base::list("error", e2, e), file = base::paste0("/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> ".error"))
#> }
#> }, interrupt = function(e) {
#> {
#> callr_data <- base::as.environment("tools:callr")$`__callr_data__`
#> err <- callr_data$err
#> if (FALSE) {
#> base::assign(".Traceback", base::.traceback(4), envir = callr_data)
#> utils::dump.frames("__callr_dump__")
#> base::assign(".Last.dump", .GlobalEnv$`__callr_dump__`,
#> envir = callr_data)
#> base::rm("__callr_dump__", envir = .GlobalEnv)
#> }
#> e <- err$process_call(e)
#> e2 <- err$new_error("error in callr subprocess")
#> class <- base::class
#> class(e2) <- base::c("callr_remote_error", class(e2))
#> e2 <- err$add_trace_back(e2)
#> cut <- base::which(e2$trace$scope == "global")[1]
#> if (!base::is.na(cut)) {
#> e2$trace <- e2$trace[-(1:cut), ]
#> }
#> base::saveRDS(base::list("error", e2, e), file = base::paste0("/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> ".error"))
#> }
#> }, callr_message = function(e) {
#> base::try(base::signalCondition(e))
#> }), error = function(e) {
#> NULL
#> if (FALSE) {
#> base::try(base::stop(e))
#> }
#> else {
#> base::invisible()
#> }
#> }, interrupt = function(e) {
#> NULL
#> if (FALSE) {
#> e
#> }
#> else {
#> base::invisible()
#> }
#> })
#>
#> [[2]]
#> tryCatchList(expr, classes, parentenv, handlers)
#>
#> [[3]]
#> tryCatchOne(tryCatchList(expr, names[-nh], parentenv, handlers[-nh]),
#> names[nh], parentenv, handlers[[nh]])
#>
#> [[4]]
#> doTryCatch(return(expr), name, parentenv, handler)
#>
#> [[5]]
#> tryCatchList(expr, names[-nh], parentenv, handlers[-nh])
#>
#> [[6]]
#> tryCatchOne(expr, names, parentenv, handlers[[1L]])
#>
#> [[7]]
#> doTryCatch(return(expr), name, parentenv, handler)
#>
#> [[8]]
#> base::withCallingHandlers({
#> NULL
#> base::saveRDS(base::do.call(base::do.call, base::c(base::readRDS("/tmp/henrik/RtmpJraagA/callr-fun-101bce40f7699e"),
#> base::list(envir = .GlobalEnv, quote = TRUE)), envir = .GlobalEnv,
#> quote = TRUE), file = "/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> compress = FALSE)
#> base::flush(base::stdout())
#> base::flush(base::stderr())
#> NULL
#> base::invisible()
#> }, error = function(e) {
#> {
#> callr_data <- base::as.environment("tools:callr")$`__callr_data__`
#> err <- callr_data$err
#> if (FALSE) {
#> base::assign(".Traceback", base::.traceback(4), envir = callr_data)
#> utils::dump.frames("__callr_dump__")
#> base::assign(".Last.dump", .GlobalEnv$`__callr_dump__`,
#> envir = callr_data)
#> base::rm("__callr_dump__", envir = .GlobalEnv)
#> }
#> e <- err$process_call(e)
#> e2 <- err$new_error("error in callr subprocess")
#> class <- base::class
#> class(e2) <- base::c("callr_remote_error", class(e2))
#> e2 <- err$add_trace_back(e2)
#> cut <- base::which(e2$trace$scope == "global")[1]
#> if (!base::is.na(cut)) {
#> e2$trace <- e2$trace[-(1:cut), ]
#> }
#> base::saveRDS(base::list("error", e2, e), file = base::paste0("/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> ".error"))
#> }
#> }, interrupt = function(e) {
#> {
#> callr_data <- base::as.environment("tools:callr")$`__callr_data__`
#> err <- callr_data$err
#> if (FALSE) {
#> base::assign(".Traceback", base::.traceback(4), envir = callr_data)
#> utils::dump.frames("__callr_dump__")
#> base::assign(".Last.dump", .GlobalEnv$`__callr_dump__`,
#> envir = callr_data)
#> base::rm("__callr_dump__", envir = .GlobalEnv)
#> }
#> e <- err$process_call(e)
#> e2 <- err$new_error("error in callr subprocess")
#> class <- base::class
#> class(e2) <- base::c("callr_remote_error", class(e2))
#> e2 <- err$add_trace_back(e2)
#> cut <- base::which(e2$trace$scope == "global")[1]
#> if (!base::is.na(cut)) {
#> e2$trace <- e2$trace[-(1:cut), ]
#> }
#> base::saveRDS(base::list("error", e2, e), file = base::paste0("/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> ".error"))
#> }
#> }, callr_message = function(e) {
#> base::try(base::signalCondition(e))
#> })
#>
#> [[9]]
#> base::saveRDS(base::do.call(base::do.call, base::c(base::readRDS("/tmp/henrik/RtmpJraagA/callr-fun-101bce40f7699e"),
#> base::list(envir = .GlobalEnv, quote = TRUE)), envir = .GlobalEnv,
#> quote = TRUE), file = "/tmp/henrik/RtmpJraagA/callr-res-101bce52c0bacf",
#> compress = FALSE)
#>
#> [[10]]
#> base::do.call(base::do.call, base::c(base::readRDS("/tmp/henrik/RtmpJraagA/callr-fun-101bce40f7699e"),
#> base::list(envir = .GlobalEnv, quote = TRUE)), envir = .GlobalEnv,
#> quote = TRUE)
#>
#> [[11]]
#> (function (what, args, quote = FALSE, envir = parent.frame())
#> {
#> if (!is.list(args))
#> stop("second argument must be a list")
#> if (quote)
#> args <- lapply(args, enquote)
#> .Internal(do.call(what, args, envir))
#> })(base::quote(function (..., cli_colors, hyperlinks)
#> {
#> options(cli.num_colors = cli_colors, cli.hyperlink = hyperlinks,
#> cli.hyperlink_run = hyperlinks)
#> pkgdown::build_site(...)
#> }), base::quote(list(pkg = list(package = "future", version = "1.58.0",
#> src_path = "/tmp/henrik/RtmpJraagA/future", meta = list(url = "https://future.futureverse.org",
#> home = list(links = list(list(text = "Roadmap/Milestones",
#> href = "https://github.com/futureverse/future/milestones"),
#> list(text = "The Futureverse Project", href = "https://www.futureverse.org/"),
#> list(text = "Futureverse User Forum", href = "https://github.com/futureverse/future/discussions"))),
#> navbar = list(structure = list(right = c("search", "futureverse",
#> "pkgs", "cran", "github", "lightswitch")), components = list(
#> futureverse = list(icon = "fas fa-home", href = "https://www.futureverse.org/"),
#> pkgs = list(text = "Packages", menu = list(list(text = "doFuture (map-reduce)",
#> href = "https://doFuture.futureverse.org"), list(
#> text = "furrr (map-reduce)", href = "https://furrr.futureverse.org"),
#> list(text = "future", href = "https://future.futureverse.org"),
#> list(text = "future.apply (map-reduce)", href = "https://future.apply.futureverse.org"),
#> list(text = "future.batchtools (backend)", href = "https://future.batchtools.futureverse.org"),
#> list(text = "future.callr (backend)", href = "https://future.callr.futureverse.org"),
#> list(text = "future.mirai (backend)", href = "https://future.mirai.futureverse.org"),
#> list(text = "future.tests", href = "https://future.tests.futureverse.org"),
#> list(text = "globals", href = "https://globals.futureverse.org"),
#> list(text = "listenv", href = "https://listenv.futureverse.org"),
#> list(text = "parallelly", href = "https://parallelly.futureverse.org"),
#> list(text = "progressr", href = "https://progressr.futureverse.org"),
#> list(text = "BiocParallel.FutureParam (experimental)",
#> href = "https://BiocParallel.FutureParam.futureverse.org"),
#> list(text = "future.tools (experimental)", href = "https://future.tools.futureverse.org"),
#> list(text = "future.mapreduce (experimental)",
#> href = "https://future.mapreduce.futureverse.org"),
#> list(text = "marshal (experimental)", href = "https://marshal.futureverse.org"))),
#> cran = list(icon = "fab fa-r-project", href = "https://cloud.r-project.org/package=future"))),
#> search = list(exclude = "README_ja.md"), template = list(
#> params = list(docsearch = list(api_key = "aa6e02fc501886fb0f7c91ac4e300456",
#> index_name = "futureverse", algoliaOptions = list(
#> facetFilters = "project:future")), ganalytics = "G-SB3EQSD9FR"),
#> bootstrap = 5L, `light-switch` = TRUE)), desc = <environment>,
#> bs_version = 5L, development = list(destination = "dev",
#> mode = "default", version_label = "muted", in_dev = FALSE,
#> prefix = ""), prefix = "", dst_path = "/tmp/henrik/RtmpJraagA/future/docs",
#> lang = "en-US", install_metadata = FALSE, figures = list(
#> dev = "ragg::agg_png", dpi = 96L, dev.args = list(),
#> fig.ext = "png", fig.width = 7.29166666666667, fig.height = NULL,
#> fig.retina = 2L, fig.asp = 0.618046971569839, bg = NULL,
#> other.parameters = list()), repo = list(url = list(home = "https://github.com/futureverse/future/",
#> source = "https://github.com/futureverse/future/blob/HEAD/",
#> issue = "https://github.com/futureverse/future/issues/",
#> user = "https://github.com/")), topics = list(name = c(`Future-class.Rd` = "Future-class",
#> FutureBackend.Rd = "FutureBackend", FutureCondition.Rd = "FutureCondition",
#> FutureGlobals.Rd = "FutureGlobals", FutureResult.Rd = "FutureResult",
#> `MulticoreFuture-class.Rd` = "MulticoreFuture-class", `MultiprocessFuture-class.Rd` = "MultiprocessFuture-class",
#> `UniprocessFuture-class.Rd` = "UniprocessFuture-class", backtrace.Rd = "backtrace",
#> cancel.Rd = "cancel", cluster.Rd = "cluster", clusterExportSticky.Rd = "clusterExportSticky",
#> find_references.Rd = "find_references", future.Rd = "future",
#> futureAssign.Rd = "futureAssign", futureOf.Rd = "futureOf",
#> futureSessionInfo.Rd = "futureSessionInfo", futures.Rd = "futures",
#> getExpression.Rd = "getExpression", getGlobalsAndPackages.Rd = "getGlobalsAndPackages",
#> makeClusterFuture.Rd = "makeClusterFuture", mandelbrot.Rd = "mandelbrot",
#> multicore.Rd = "multicore", multisession.Rd = "multisession",
#> nbrOfWorkers.Rd = "nbrOfWorkers", nullcon.Rd = "nullcon",
#> plan.Rd = "with.FutureStrategyList", private_length.Rd = ".length",
#> `re-exports.Rd` = "re-exports", readImmediateConditions.Rd = "readImmediateConditions",
#> requestCore.Rd = "requestCore", reset.Rd = "reset", resetWorkers.Rd = "resetWorkers",
#> resolve.Rd = "resolve", resolved.Rd = "resolved", result.Rd = "result.Future",
#> run.Rd = "run.Future", save_rds.Rd = "save_rds", sequential.Rd = "sequential",
#> sessionDetails.Rd = "sessionDetails", signalConditions.Rd = "signalConditions",
#> sticky_globals.Rd = "sticky_globals", usedCores.Rd = "usedCores",
#> value.Rd = "value", `zzz-future.options.Rd` = "zzz-future.options"
#> ), file_in = c("Future-class.Rd", "FutureBackend.Rd", "FutureCondition.Rd",
#> "FutureGlobals.Rd", "FutureResult.Rd", "MulticoreFuture-class.Rd",
#> "MultiprocessFuture-class.Rd", "UniprocessFuture-class.Rd",
#> "backtrace.Rd", "cancel.Rd", "cluster.Rd", "clusterExportSticky.Rd",
#> "find_references.Rd", "future.Rd", "futureAssign.Rd", "futureOf.Rd",
#> "futureSessionInfo.Rd", "futures.Rd", "getExpression.Rd",
#> "getGlobalsAndPackages.Rd", "makeClusterFuture.Rd", "mandelbrot.Rd",
#> "multicore.Rd", "multisession.Rd", "nbrOfWorkers.Rd", "nullcon.Rd",
#> "plan.Rd", "private_length.Rd", "re-exports.Rd", "readImmediateConditions.Rd",
#> "requestCore.Rd", "reset.Rd", "resetWorkers.Rd", "resolve.Rd",
#> "resolved.Rd", "result.Rd", "run.Rd", "save_rds.Rd", "sequential.Rd",
#> "sessionDetails.Rd", "signalConditions.Rd", "sticky_globals.Rd",
#> "usedCores.Rd", "value.Rd", "zzz-future.options.Rd"), file_out = c("Future-class.html",
#> "FutureBackend.html", "FutureCondition.html", "FutureGlobals.html",
#> "FutureResult.html", "MulticoreFuture-class.html", "MultiprocessFuture-class.html",
#> "UniprocessFuture-class.html", "backtrace.html", "cancel.html",
#> "cluster.html", "clusterExportSticky.html", "find_references.html",
#> "future.html", "futureAssign.html", "futureOf.html", "futureSessionInfo.html",
#> "futures.html", "getExpression.html", "getGlobalsAndPackages.html",
#> "makeClusterFuture.html", "mandelbrot.html", "multicore.html",
#> "multisession.html", "nbrOfWorkers.html", "nullcon.html",
#> "plan.html", "private_length.html", "re-exports.html", "readImmediateConditions.html",
#> "requestCore.html", "reset.html", "resetWorkers.html", "resolve.html",
#> "resolved.html", "result.html", "run.html", "save_rds.html",
#> "sequential.html", "sessionDetails.html", "signalConditions.html",
#> "sticky_globals.html", "usedCores.html", "value.html", "zzz-future.options.html"
#> ), alias = list(`Future-class.Rd` = c("Future-class", "Future"
#> ), FutureBackend.Rd = c("FutureBackend", "launchFuture",
#> "listFutures", "interruptFuture", "validateFutureGlobals",
#> "stopWorkers", "MultiprocessFutureBackend", "ClusterFutureBackend",
#> "MulticoreFutureBackend", "SequentialFutureBackend", "MultisessionFutureBackend"
#> ), FutureCondition.Rd = c("FutureCondition", "FutureMessage",
#> "FutureWarning", "FutureError", "RngFutureCondition", "RngFutureWarning",
#> "RngFutureError", "UnexpectedFutureResultError", "GlobalEnvMisuseFutureCondition",
#> "GlobalEnvMisuseFutureWarning", "GlobalEnvMisuseFutureError",
#> "ConnectionMisuseFutureCondition", "ConnectionMisuseFutureWarning",
#> "ConnectionMisuseFutureError", "DeviceMisuseFutureCondition",
#> "DeviceMisuseFutureWarning", "DeviceMisuseFutureError", "DefaultDeviceMisuseFutureCondition",
#> "DefaultDeviceMisuseFutureWarning", "DefaultDeviceMisuseFutureError",
#> "FutureLaunchError", "FutureInterruptError", "FutureCanceledError",
#> "FutureDroppedError", "FutureJournalCondition"), FutureGlobals.Rd = c("FutureGlobals",
#> "as.FutureGlobals", "as.FutureGlobals.FutureGlobals", "as.FutureGlobals.Globals",
#> "as.FutureGlobals.list", "[.FutureGlobals", "c.FutureGlobals",
#> "unique.FutureGlobals"), FutureResult.Rd = c("FutureResult",
#> "as.character.FutureResult", "print.FutureResult"), `MulticoreFuture-class.Rd` = c("MulticoreFuture-class",
#> "resolved.MulticoreFuture"), `MultiprocessFuture-class.Rd` = c("MultiprocessFuture-class",
#> "MultiprocessFuture"), `UniprocessFuture-class.Rd` = c("UniprocessFuture-class",
#> "UniprocessFuture"), backtrace.Rd = "backtrace", cancel.Rd = "cancel",
#> cluster.Rd = "cluster", clusterExportSticky.Rd = "clusterExportSticky",
#> find_references.Rd = c("find_references", "assert_no_references"
#> ), future.Rd = c("future", "futureCall", "minifuture"
#> ), futureAssign.Rd = c("futureAssign", "%<-%", "%->%",
#> "%globals%", "%packages%", "%seed%", "%stdout%", "%conditions%",
#> "%lazy%", "%label%", "%plan%", "%tweak%"), futureOf.Rd = "futureOf",
#> futureSessionInfo.Rd = "futureSessionInfo", futures.Rd = "futures",
#> getExpression.Rd = c("getExpression", "getExpression.Future"
#> ), getGlobalsAndPackages.Rd = "getGlobalsAndPackages",
#> makeClusterFuture.Rd = c("makeClusterFuture", "FUTURE"
#> ), mandelbrot.Rd = c("mandelbrot", "as.raster.Mandelbrot",
#> "plot.Mandelbrot", "mandelbrot_tiles", "mandelbrot.matrix",
#> "mandelbrot.numeric"), multicore.Rd = "multicore", multisession.Rd = "multisession",
#> nbrOfWorkers.Rd = c("nbrOfWorkers", "nbrOfFreeWorkers"
#> ), nullcon.Rd = "nullcon", plan.Rd = c("with.FutureStrategyList",
#> "plan", "tweak"), private_length.Rd = ".length", `re-exports.Rd` = c("re-exports",
#> "as.cluster", "availableCores", "availableWorkers", "makeClusterPSOCK",
#> "supportsMulticore"), readImmediateConditions.Rd = c("readImmediateConditions",
#> "saveImmediateCondition"), requestCore.Rd = "requestCore",
#> reset.Rd = "reset", resetWorkers.Rd = "resetWorkers",
#> resolve.Rd = "resolve", resolved.Rd = "resolved", result.Rd = c("result.Future",
#> "result"), run.Rd = c("run.Future", "run"), save_rds.Rd = "save_rds",
#> sequential.Rd = c("sequential", "uniprocess"), sessionDetails.Rd = "sessionDetails",
#> signalConditions.Rd = "signalConditions", sticky_globals.Rd = "sticky_globals",
#> usedCores.Rd = "usedCores", value.Rd = c("value", "value.Future",
#> "value.list", "value.listenv", "value.environment"),
#> `zzz-future.options.Rd` = c("zzz-future.options", "future.options",
#> "future.startup.script", "future.debug", "future.demo.mandelbrot.region",
#> "future.demo.mandelbrot.nrow", "future.fork.multithreading.enable",
#> "future.globals.maxSize", "future.globals.method", "future.globals.onMissing",
#> "future.globals.resolve", "future.globals.onReference",
#> "future.plan", "future.onFutureCondition.keepFuture",
#> "future.resolve.recursive", "future.connections.onMisuse",
#> "future.defaultDevice.onMisuse", "future.devices.onMisuse",
#> "future.globalenv.onMisuse", "future.rng.onMisuse", "future.wait.alpha",
#> "future.wait.interval", "future.wait.timeout", "future.output.windows.reencode",
#> "future.journal", "future.globals.objectSize.method",
#> "future.ClusterFuture.clusterEvalQ", "R_FUTURE_STARTUP_SCRIPT",
#> "R_FUTURE_DEBUG", "R_FUTURE_DEMO_MANDELBROT_REGION",
#> "R_FUTURE_DEMO_MANDELBROT_NROW", "R_FUTURE_FORK_MULTITHREADING_ENABLE",
#> "R_FUTURE_GLOBALS_MAXSIZE", "R_FUTURE_GLOBALS_METHOD",
#> "R_FUTURE_GLOBALS_ONMISSING", "R_FUTURE_GLOBALS_RESOLVE",
#> "R_FUTURE_GLOBALS_ONREFERENCE", "R_FUTURE_PLAN", "R_FUTURE_ONFUTURECONDITION_KEEPFUTURE",
#> "R_FUTURE_RESOLVE_RECURSIVE", "R_FUTURE_CONNECTIONS_ONMISUSE",
#> "R_FUTURE_DEVICES_ONMISUSE", "R_FUTURE_DEFAULTDEVICE_ONMISUSE",
#> "R_FUTURE_GLOBALENV_ONMISUSE", "R_FUTURE_RNG_ONMISUSE",
#> "R_FUTURE_WAIT_ALPHA", "R_FUTURE_WAIT_INTERVAL", "R_FUTURE_WAIT_TIMEOUT",
#> "R_FUTURE_RESOLVED_TIMEOUT", "R_FUTURE_OUTPUT_WINDOWS_REENCODE",
#> "R_FUTURE_JOURNAL", "R_FUTURE_GLOBALS_OBJECTSIZE_METHOD",
#> "R_FUTURE_CLUSTERFUTURE_CLUSTEREVALQ", "future.cmdargs",
#> ".future.R")), funs = list(`Future-class.Rd` = "Future()",
#> FutureBackend.Rd = c("FutureBackend()", "launchFuture()",
#> "listFutures()", "interruptFuture()", "validateFutureGlobals()",
#> "stopWorkers()", "MultiprocessFutureBackend()", "ClusterFutureBackend()",
#> "MulticoreFutureBackend()", "SequentialFutureBackend()",
#> "MultisessionFutureBackend()"), FutureCondition.Rd = c("FutureCondition()",
#> "FutureMessage()", "FutureWarning()", "FutureError()",
#> "RngFutureCondition()", "RngFutureWarning()", "RngFutureError()",
#> "UnexpectedFutureResultError()", "GlobalEnvMisuseFutureCondition()",
#> "GlobalEnvMisuseFutureWarning()", "GlobalEnvMisuseFutureError()",
#> "ConnectionMisuseFutureCondition()", "ConnectionMisuseFutureWarning()",
#> "ConnectionMisuseFutureError()", "DeviceMisuseFutureCondition()",
#> "DeviceMisuseFutureWarning()", "DeviceMisuseFutureError()",
#> "DefaultDeviceMisuseFutureCondition()", "DefaultDeviceMisuseFutureWarning()",
#> "DefaultDeviceMisuseFutureError()", "FutureLaunchError()",
#> "FutureInterruptError()", "FutureCanceledError()", "FutureDroppedError()",
#> "FutureJournalCondition()"), FutureGlobals.Rd = "FutureGlobals()",
#> FutureResult.Rd = c("FutureResult()", "as.character(<i><FutureResult></i>)",
#> "print(<i><FutureResult></i>)"), `MulticoreFuture-class.Rd` = "resolved(<i><MulticoreFuture></i>)",
#> `MultiprocessFuture-class.Rd` = "MultiprocessFuture()",
#> `UniprocessFuture-class.Rd` = "UniprocessFuture()", backtrace.Rd = "backtrace()",
#> cancel.Rd = "cancel()", cluster.Rd = "cluster()", clusterExportSticky.Rd = "clusterExportSticky()",
#> find_references.Rd = c("find_references()", "assert_no_references()"
#> ), future.Rd = c("future()", "futureCall()", "minifuture()"
#> ), futureAssign.Rd = c("futureAssign()", "`%<-%`",
#> "`%globals%`", "`%packages%`", "`%seed%`", "`%stdout%`",
#> "`%conditions%`", "`%lazy%`", "`%label%`", "`%plan%`",
#> "`%tweak%`"), futureOf.Rd = "futureOf()", futureSessionInfo.Rd = "futureSessionInfo()",
#> futures.Rd = "futures()", getExpression.Rd = "getExpression()",
#> getGlobalsAndPackages.Rd = "getGlobalsAndPackages()",
#> makeClusterFuture.Rd = "makeClusterFuture()", mandelbrot.Rd = "mandelbrot()",
#> multicore.Rd = "multicore()", multisession.Rd = "multisession()",
#> nbrOfWorkers.Rd = c("nbrOfWorkers()", "nbrOfFreeWorkers()"
#> ), nullcon.Rd = "nullcon()", plan.Rd = c("with(<i><FutureStrategyList></i>)",
#> "plan()", "tweak()"), private_length.Rd = ".length()",
#> `re-exports.Rd` = character(0), readImmediateConditions.Rd = c("readImmediateConditions()",
#> "saveImmediateCondition()"), requestCore.Rd = "requestCore()",
#> reset.Rd = "reset()", resetWorkers.Rd = "resetWorkers()",
#> resolve.Rd = "resolve()", resolved.Rd = "resolved()",
#> result.Rd = "result(<i><Future></i>)", run.Rd = "run(<i><Future></i>)",
#> save_rds.Rd = "save_rds()", sequential.Rd = "sequential()",
#> sessionDetails.Rd = "sessionDetails()", signalConditions.Rd = "signalConditions()",
#> sticky_globals.Rd = "sticky_globals()", usedCores.Rd = "usedCores()",
#> value.Rd = "value()", `zzz-future.options.Rd` = character(0)),
#> title = c(`Future-class.Rd` = "A future represents a value that will be available at some point in the future",
#> FutureBackend.Rd = "Configure a backend that controls how and where futures are evaluated",
#> FutureCondition.Rd = "A condition (message, warning, or error) that occurred while orchestrating a future",
#> FutureGlobals.Rd = "A representation of a set of globals used with futures",
#> FutureResult.Rd = "Results from resolving a future",
#> `MulticoreFuture-class.Rd` = "A multicore future is a future whose value will be resolved asynchronously in a parallel process",
#> `MultiprocessFuture-class.Rd` = "A multiprocess future is a future whose value will be resolved asynchronously in a parallel process",
#> `UniprocessFuture-class.Rd` = "An uniprocess future is a future whose value will be resolved synchronously in the current process",
#> backtrace.Rd = "Back trace the expressions evaluated when an error was caught",
#> cancel.Rd = "Cancel a future", cluster.Rd = "Create a cluster future whose value will be resolved asynchronously in a parallel process",
#> clusterExportSticky.Rd = "Export globals to the sticky-globals environment of the cluster nodes",
#> find_references.Rd = "Get the first or all references of an <span style=\"R\">R</span> object",
#> future.Rd = "Create a future", futureAssign.Rd = "Create a future assignment",
#> futureOf.Rd = "Get the future of a future variable",
#> futureSessionInfo.Rd = "Get future-specific session information and validate current backend",
#> futures.Rd = "Get all futures in a container", getExpression.Rd = "Inject code for the next type of future to use for nested futures",
#> getGlobalsAndPackages.Rd = "Retrieves global variables of an expression and their associated packages",
#> makeClusterFuture.Rd = "Create a Future Cluster of Stateless Workers for Parallel Processing",
#> mandelbrot.Rd = "Mandelbrot convergence counts", multicore.Rd = "Create a multicore future whose value will be resolved asynchronously in a forked parallel process",
#> multisession.Rd = "Create a multisession future whose value will be resolved asynchronously in a parallel <span style=\"R\">R</span> session",
#> nbrOfWorkers.Rd = "Get the number of workers available",
#> nullcon.Rd = "Creates a connection to the system null device",
#> plan.Rd = "Evaluate an expression using a temporarily set future plan",
#> private_length.Rd = "Gets the length of an object without dispatching",
#> `re-exports.Rd` = "Functions Moved to 'parallelly'",
#> readImmediateConditions.Rd = "Writes and Reads 'immediateCondition' RDS Files",
#> requestCore.Rd = "Request a core for multicore processing",
#> reset.Rd = "Reset a finished, failed, canceled, or interrupted future to a lazy future",
#> resetWorkers.Rd = "Free up active background workers",
#> resolve.Rd = "Resolve one or more futures synchronously",
#> resolved.Rd = "Check whether a future is resolved or not",
#> result.Rd = "Get the results of a resolved future", run.Rd = "Run a future",
#> save_rds.Rd = "Robustly Saves an Object to RDS File Atomically",
#> sequential.Rd = "Create a sequential future whose value will be in the current <span style=\"R\">R</span> session",
#> sessionDetails.Rd = "Outputs details on the current <span style=\"R\">R</span> session",
#> signalConditions.Rd = "Signals Captured Conditions",
#> sticky_globals.Rd = "Place a sticky-globals environment immediately after the global environment",
#> usedCores.Rd = "Get number of cores currently used",
#> value.Rd = "The value of a future or the values of all elements in a container",
#> `zzz-future.options.Rd` = "Options used for futures"),
#> rd = list(`Future-class.Rd` = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("Future-class"), "\n", list("Future-class"),
#> "\n", list("Future"), "\n", list("A future represents a value that will be available at some point in the future"),
#> "\n", list("\n", "Future(\n", " expr = NULL,\n",
#> " envir = parent.frame(),\n", " substitute = TRUE,\n",
#> " stdout = TRUE,\n", " conditions = \"condition\",\n",
#> " globals = list(),\n", " packages = NULL,\n",
#> " seed = FALSE,\n", " lazy = FALSE,\n", " gc = FALSE,\n",
#> " earlySignal = FALSE,\n", " label = NULL,\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "expr"), list("An ", list(), " ", list("expression"),
#> ".")), "\n", "\n", list(list("envir"), list("The ",
#> list("environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list("substitute"),
#> list("If TRUE, argument ", list("expr"), " is\n",
#> list(list("substitute"), "()"), ":ed, otherwise not.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE (default), then the standard output is captured,\n",
#> "and re-outputted when ", list("value()"),
#> " is called.\n", "If FALSE, any output is silenced (by sinking it to the null device as\n",
#> "it is outputted).\n", "Using ", list("stdout = structure(TRUE, drop = TRUE)"),
#> " causes the captured\n", "standard output to be dropped from the future object as soon as it has\n",
#> "been relayed. This can help decrease the overall memory consumed by\n",
#> "captured output across futures.\n", "Using ",
#> list("stdout = NA"), " fully avoids intercepting the standard output;\n",
#> "behavior of such unhandled standard output depends on the future backend.")),
#> "\n", "\n", list(list("conditions"), list("A character string of conditions classes to be captured\n",
#> "and relayed. The default is to relay all conditions, including messages\n",
#> "and warnings. To drop all conditions, use ",
#> list("conditions = character(0)"), ".\n", "Errors are always relayed.\n",
#> "Attribute ", list("exclude"), " can be used to ignore specific classes, e.g.\n",
#> list("conditions = structure(\"condition\", exclude = \"message\")"),
#> " will capture\n", "all ", list("condition"),
#> " classes except those that inherits from the ",
#> list("message"), " class.\n", "Using ", list(
#> "conditions = structure(..., drop = TRUE)"),
#> " causes any captured\n", "conditions to be dropped from the future object as soon as it has\n",
#> "been relayed, e.g. by ", list("value(f)"),
#> ". This can help decrease the overall\n", "memory consumed by captured conditions across futures.\n",
#> "Using ", list("conditions = NULL"), " (not recommended) avoids intercepting conditions,\n",
#> "except from errors; behavior of such unhandled conditions depends on the\n",
#> "future backend and the environment from which R runs.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, or a named list\n",
#> "to control how globals are handled.\n", "For details, see section 'Globals used by future expressions'\n",
#> "in the help for ", list(list("future()")),
#> ".")), "\n", "\n", list(list("packages"), list(
#> "(optional) a character vector specifying packages\n",
#> "to be attached in the ", list(), " environment evaluating the future.")),
#> "\n", "\n", list(list("seed"), list("(optional) If TRUE, the random seed, that is, the state of the\n",
#> "random number generator (RNG) will be set such that statistically sound\n",
#> "random numbers are produced (also during parallelization).\n",
#> "If FALSE (default), it is assumed that the future expression does neither\n",
#> "need nor use random numbers generation.\n",
#> "To use a fixed random seed, specify a L'Ecuyer-CMRG seed (seven integer)\n",
#> "or a regular RNG seed (a single integer). If the latter, then a\n",
#> "L'Ecuyer-CMRG seed will be automatically created based on the given seed.\n",
#> "Furthermore, if FALSE, then the future will be monitored to make sure it\n",
#> "does not use random numbers. If it does and depending on the value of\n",
#> "option ", list("future.rng.onMisuse"), ", the check is\n",
#> "ignored, an informative warning, or error will be produced.\n",
#> "If ", list("seed"), " is NULL, then the effect is as with ",
#> list("seed = FALSE"), "\n", "but without the RNG check being performed.")),
#> "\n", "\n", list(list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ", list(
#> "earlySignal"), " is TRUE (more\n", "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list("Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(list(
#> "label"), list("A character string label attached to the future.")),
#> "\n", "\n", list(list(list()), list("Additional named elements of the future.")),
#> "\n"), "\n", list("\n", list("Future()"), " returns an object of class ",
#> list("Future"), ".\n"), "\n", list("\n", "A ",
#> list("future"), " is an abstraction for a ",
#> list("value"), " that may\n", "available at some point in the future. A future can either be\n",
#> list("unresolved"), " or ", list("resolved"),
#> ", a state which can be checked\n", "with ",
#> list(list("resolved()")), ". As long as it is ",
#> list("unresolved"), ", the\n", "value is not available. As soon as it is ",
#> list("resolved"), ", the value\n", "is available via ",
#> list(list("value"), "()"), ".\n"), "\n", list(
#> "\n", "A Future object is itself an ", list("environment"),
#> ".\n"), "\n", list("\n", "One function that creates a Future is ",
#> list(list("future()")), ".\n", "It returns a Future that evaluates an ",
#> list(), " expression in the future.\n", "An alternative approach is to use the ",
#> list(list("%<-%")), " infix\n", "assignment operator, which creates a future from the\n",
#> "right-hand-side (RHS) ", list(), " expression and assigns its future value\n",
#> "to a variable as a ", list(list("promise")),
#> ".\n"), "\n", list("internal"), "\n"), FutureBackend.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/backend_api-01-FutureBackend-class.R,",
#> "\n", "% R/backend_api-03.MultiprocessFutureBackend-class.R,",
#> "\n", "% R/backend_api-11.ClusterFutureBackend-class.R,",
#> "\n", "% R/backend_api-11.MulticoreFutureBackend-class.R,",
#> "\n", "% R/backend_api-11.SequentialFutureBackend-class.R,",
#> "\n", "% R/backend_api-13.MultisessionFutureBackend-class.R",
#> "\n", list("FutureBackend"), "\n", list("FutureBackend"),
#> "\n", list("launchFuture"), "\n", list("listFutures"),
#> "\n", list("interruptFuture"), "\n", list("validateFutureGlobals"),
#> "\n", list("stopWorkers"), "\n", list("MultiprocessFutureBackend"),
#> "\n", list("ClusterFutureBackend"), "\n", list("MulticoreFutureBackend"),
#> "\n", list("SequentialFutureBackend"), "\n", list(
#> "MultisessionFutureBackend"), "\n", list("Configure a backend that controls how and where futures are evaluated"),
#> "\n", list("\n", "FutureBackend(\n", " ...,\n",
#> " earlySignal = FALSE,\n", " gc = FALSE,\n",
#> " maxSizeOfObjects = getOption(\"future.globals.maxSize\", +Inf),\n",
#> " interrupts = TRUE,\n", " hooks = FALSE\n",
#> ")\n", "\n", "launchFuture(backend, future, ...)\n",
#> "\n", "listFutures(backend, ...)\n", "\n", "interruptFuture(backend, future, ...)\n",
#> "\n", "validateFutureGlobals(backend, future, ...)\n",
#> "\n", "stopWorkers(backend, ...)\n", "\n", "MultiprocessFutureBackend(\n",
#> " ...,\n", " wait.timeout = getOption(\"future.wait.timeout\", 24 * 60 * 60),\n",
#> " wait.interval = getOption(\"future.wait.interval\", 0.01),\n",
#> " wait.alpha = getOption(\"future.wait.alpha\", 1.01)\n",
#> ")\n", "\n", "ClusterFutureBackend(\n", " workers = availableWorkers(constraints = \"connections\"),\n",
#> " gc = TRUE,\n", " earlySignal = TRUE,\n",
#> " interrupts = FALSE,\n", " persistent = FALSE,\n",
#> " ...\n", ")\n", "\n", "MulticoreFutureBackend(\n",
#> " workers = availableCores(constraints = \"multicore\"),\n",
#> " maxSizeOfObjects = +Inf,\n", " ...\n", ")\n",
#> "\n", "SequentialFutureBackend(..., maxSizeOfObjects = +Inf)\n",
#> "\n", "MultisessionFutureBackend(\n", " workers = availableCores(),\n",
#> " rscript_libs = .libPaths(),\n", " interrupts = TRUE,\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "earlySignal"), list("Overrides the default behavior on whether futures\n",
#> "should resignal (\"relay\") conditions captured as soon as possible, or\n",
#> "delayed, for instance, until ", list(list("value()")),
#> " is called on the future.\n", "(Default: ",
#> list("FALSE"), ")")), "\n", "\n", list(list("gc"),
#> list("Overrides the default behavior of whether futures should trigger\n",
#> "garbage collection via ", list(list("gc()")),
#> " on the parallel worker after the value has\n",
#> "been collected from the worker.\n", "This can help to release memory sooner than letting R itself on the parallel\n",
#> "worker decided when it is needed. Releasing memory sooner can help to fit\n",
#> "more parallel workers on a machine with limited amount of total memory.\n",
#> "(Default: ", list("FALSE"), ")")), "\n", "\n",
#> list(list("maxSizeOfObjects"), list("The maximum allowed total size, in bytes, of all\n",
#> "objects to and from the parallel worker allows.\n",
#> "This can help to protect against unexpectedly large data transfers between\n",
#> "the parent process and the parallel workers - data that is often transferred\n",
#> "over the network, which sometimes also includes the internet. For instance,\n",
#> "if you sit at home and have set up a future backend with workers running\n",
#> "remotely at your university or company, then you might want to use this\n",
#> "protection to avoid transferring giga- or terabytes of data without noticing.\n",
#> "(Default: ", list(list("500 \\cdot 1024^2")),
#> " bytes = 500 MiB, unless overridden by a\n",
#> "FutureBackend subclass, or by R option ",
#> list("future.globals.maxSize"), " (sic!))")),
#> "\n", "\n", list(list("interrupts"), list("If FALSE, attempts to interrupt futures will not take\n",
#> "place on this backend, even if the backend supports it. This is useful\n",
#> "when, for instance, it takes a long time to interrupt a future.")),
#> "\n", "\n", list(list("backend"), list("a ",
#> list("FutureBackend"), ".")), "\n", "\n", list(
#> list("future"), list("a ", list("Future"),
#> " to be started.")), "\n", "\n", list(list(
#> "wait.timeout"), list("Number of seconds before timing out.")),
#> "\n", "\n", list(list("wait.interval"), list(
#> "Baseline number of seconds between retries.")),
#> "\n", "\n", list(list("wait.alpha"), list("Scale factor increasing waiting interval after each\n",
#> "attempt.")), "\n", "\n", list(list("workers"),
#> list("...")), "\n", "\n", list(list("persistent"),
#> list("(deprecated) ...")), "\n", "\n", list(
#> list(list()), list("(optional) not used.")),
#> "\n"), "\n", list("\n", list("FutureBackend()"),
#> " returns a FutureBackend object, which inherits an\n",
#> "environment. Specific future backends are defined by subclasses\n",
#> "implementing the FutureBackend API.\n", "\n",
#> list("launchFuture()"), " returns the launched ",
#> list("Future"), " object.\n", "\n", list("interruptFuture()"),
#> " returns the interrupted ", list("Future"),
#> " object,\n", "if supported, other the unmodified future.\n",
#> "\n", list("stopWorkers()"), " returns TRUE if the workers were shut down,\n",
#> "otherwise FALSE.\n"), "\n", list("\n", list(
#> "This functionality is only for developers who wish to implement their\n",
#> "own future backend. End-users and package developers use futureverse,\n",
#> "does not need to know about these functions."),
#> "\n", "\n", "If you are looking for available future backends to choose from, please\n",
#> "see the 'A Future for R: Available Future Backends' vignette and\n",
#> list("https://www.futureverse.org/backends.html"),
#> ".\n"), "\n", list("\n", "The ", list("ClusterFutureBackend"),
#> " is selected by\n", list("plan(cluster, workers = workers)"),
#> ".\n", "\n", "The ", list("MulticoreFutureBackend"),
#> " backend is selected by\n", list("plan(multicore, workers = workers)"),
#> ".\n", "\n", "The ", list("SequentialFutureBackend"),
#> " is selected by ", list("plan(sequential)"),
#> ".\n", "\n", "The ", list("MultisessionFutureBackend"),
#> " backend is selected by\n", list("plan(multisession, workers = workers)"),
#> ".\n"), "\n", list(list("The FutureBackend API"),
#> list("\n", "\n", "The ", list("FutureBackend"),
#> " class specifies FutureBackend API,\n", "that all backends must implement and comply to. Specifically,\n")),
#> "\n", "\n", list("\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n", "\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n"), "\n", list("internal"), "\n"), FutureCondition.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/protected_api-FutureCondition-class.R,",
#> "\n", "% R/protected_api-journal.R", "\n", list(
#> "FutureCondition"), "\n", list("FutureCondition"),
#> "\n", list("FutureMessage"), "\n", list("FutureWarning"),
#> "\n", list("FutureError"), "\n", list("RngFutureCondition"),
#> "\n", list("RngFutureWarning"), "\n", list("RngFutureError"),
#> "\n", list("UnexpectedFutureResultError"), "\n",
#> list("GlobalEnvMisuseFutureCondition"), "\n", list(
#> "GlobalEnvMisuseFutureWarning"), "\n", list("GlobalEnvMisuseFutureError"),
#> "\n", list("ConnectionMisuseFutureCondition"), "\n",
#> list("ConnectionMisuseFutureWarning"), "\n", list(
#> "ConnectionMisuseFutureError"), "\n", list("DeviceMisuseFutureCondition"),
#> "\n", list("DeviceMisuseFutureWarning"), "\n", list(
#> "DeviceMisuseFutureError"), "\n", list("DefaultDeviceMisuseFutureCondition"),
#> "\n", list("DefaultDeviceMisuseFutureWarning"), "\n",
#> list("DefaultDeviceMisuseFutureError"), "\n", list(
#> "FutureLaunchError"), "\n", list("FutureInterruptError"),
#> "\n", list("FutureCanceledError"), "\n", list("FutureDroppedError"),
#> "\n", list("FutureJournalCondition"), "\n", list(
#> "A condition (message, warning, or error) that occurred while orchestrating a future"),
#> "\n", list("\n", "FutureCondition(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "FutureMessage(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "FutureWarning(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "FutureError(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "RngFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " uuid = future[[\"uuid\"]],\n",
#> " future = NULL\n", ")\n", "\n", "RngFutureWarning(...)\n",
#> "\n", "RngFutureError(...)\n", "\n", "UnexpectedFutureResultError(future, hint = NULL)\n",
#> "\n", "GlobalEnvMisuseFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " differences = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "GlobalEnvMisuseFutureWarning(...)\n",
#> "\n", "GlobalEnvMisuseFutureError(...)\n", "\n",
#> "ConnectionMisuseFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " differences = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "ConnectionMisuseFutureWarning(...)\n",
#> "\n", "ConnectionMisuseFutureError(...)\n", "\n",
#> "DeviceMisuseFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " differences = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "DeviceMisuseFutureWarning(...)\n",
#> "\n", "DeviceMisuseFutureError(...)\n", "\n",
#> "DefaultDeviceMisuseFutureCondition(\n", " message = NULL,\n",
#> " incidents = NULL,\n", " call = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "DefaultDeviceMisuseFutureWarning(...)\n",
#> "\n", "DefaultDeviceMisuseFutureError(...)\n",
#> "\n", "FutureLaunchError(..., future = NULL)\n",
#> "\n", "FutureInterruptError(..., future = NULL)\n",
#> "\n", "FutureCanceledError(..., future = NULL)\n",
#> "\n", "FutureDroppedError(..., future = NULL)\n",
#> "\n", "FutureJournalCondition(\n", " message,\n",
#> " journal,\n", " call = NULL,\n", " uuid = future[[\"uuid\"]],\n",
#> " future = NULL\n", ")\n"), "\n", list("\n",
#> list(list("message"), list("A message condition.")),
#> "\n", "\n", list(list("call"), list("The call stack that led up to the condition.")),
#> "\n", "\n", list(list("uuid"), list("A universally unique identifier for the future associated with\n",
#> "this FutureCondition.")), "\n", "\n", list(
#> list("future"), list("The ", list("Future"),
#> " involved.")), "\n", "\n", list(list("hint"),
#> list("(optional) A string with a suggestion on what might be wrong.")),
#> "\n"), "\n", list("\n", "An object of class FutureCondition which inherits from class\n",
#> list("condition"), " and FutureMessage, FutureWarning,\n",
#> "and FutureError all inherits from FutureCondition.\n",
#> "Moreover, a FutureError inherits from ", list(
#> "error"), ",\n", "a FutureWarning from ", list(
#> "warning"), ", and\n", "a FutureMessage from ",
#> list("message"), ".\n"), "\n", list("\n", "While ",
#> list("orchestrating"), " (creating, launching, querying, collection)\n",
#> "futures, unexpected run-time errors (and other types of conditions) may\n",
#> "occur. Such conditions are coerced to a corresponding FutureCondition\n",
#> "class to help distinguish them from conditions that occur due to the\n",
#> list("evaluation"), " of the future.\n"), "\n",
#> list("internal"), "\n"), FutureGlobals.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/protected_api-FutureGlobals-class.R",
#> "\n", list("FutureGlobals"), "\n", list("FutureGlobals"),
#> "\n", list("as.FutureGlobals"), "\n", list("as.FutureGlobals.FutureGlobals"),
#> "\n", list("as.FutureGlobals.Globals"), "\n", list(
#> "as.FutureGlobals.list"), "\n", list("[.FutureGlobals"),
#> "\n", list("c.FutureGlobals"), "\n", list("unique.FutureGlobals"),
#> "\n", list("A representation of a set of globals used with futures"),
#> "\n", list("\n", "FutureGlobals(object = list(), resolved = FALSE, total_size = NA_real_, ...)\n"),
#> "\n", list("\n", list(list("object"), list("A named list.")),
#> "\n", "\n", list(list("resolved"), list("A logical indicating whether these globals\n",
#> "have been scanned for and resolved futures or not.")),
#> "\n", "\n", list(list("total_size"), list("The total size of all globals, if known.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "An object of class ",
#> list("FutureGlobals"), ".\n"), "\n", list("\n",
#> "A representation of a set of globals used with futures\n"),
#> "\n", list("\n", "This class extends the ", list(
#> "Globals"), " class by adding\n", "attributes ",
#> list("resolved"), " and ", list("total_size"),
#> ".\n"), "\n", list("internal"), "\n"), FutureResult.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/protected_api-FutureResult-class.R",
#> "\n", list("FutureResult"), "\n", list("FutureResult"),
#> "\n", list("as.character.FutureResult"), "\n", list(
#> "print.FutureResult"), "\n", list("Results from resolving a future"),
#> "\n", list("\n", "FutureResult(\n", " value = NULL,\n",
#> " visible = TRUE,\n", " stdout = NULL,\n",
#> " conditions = NULL,\n", " rng = FALSE,\n",
#> " ...,\n", " uuid = NULL,\n", " started = .POSIXct(NA_real_),\n",
#> " finished = Sys.time(),\n", " version = \"1.8\"\n",
#> ")\n", "\n", list(list("as.character"), list(
#> "FutureResult")), "(x, ...)\n", "\n", list(
#> list("print"), list("FutureResult")), "(x, ...)\n"),
#> "\n", list("\n", list(list("value"), list("The value of the future expression.\n",
#> "If the expression was not fully resolved (e.g. an error) occurred,\n",
#> "the the value is ", list("NULL"), ".")), "\n",
#> "\n", list(list("visible"), list("If TRUE, the value was visible, otherwise invisible.")),
#> "\n", "\n", list(list("conditions"), list("A list of zero or more list elements each containing\n",
#> "a captured ", list("condition"), " and possibly more meta data such as the\n",
#> "call stack and a timestamp.")), "\n", "\n",
#> list(list("rng"), list("If TRUE, the ", list(
#> ".Random.seed"), " was updated from resolving the\n",
#> "future, otherwise not.")), "\n", "\n", list(
#> list("started, finished"), list(list("POSIXct"),
#> " timestamps\n", "when the evaluation of the future expression was started and finished.")),
#> "\n", "\n", list(list("version"), list("The version format of the results.")),
#> "\n", "\n", list(list(list()), list("(optional) Additional named results to be returned.")),
#> "\n"), "\n", list("\n", "An object of class FutureResult.\n"),
#> "\n", list("\n", "Results from resolving a future\n"),
#> "\n", list("\n", "This function is only part of the ",
#> list("backend"), " Future API.\n", "This function is ",
#> list("not"), " part of the frontend Future API.\n"),
#> "\n", list(list("Note to developers"), list("\n",
#> "\n", "The FutureResult structure is ", list(
#> "under development"), " and may change at anytime,\n",
#> "e.g. elements may be renamed or removed. Because of this, please avoid\n",
#> "accessing the elements directly in code. Feel free to reach out if you need\n",
#> "to do so in your code.\n")), "\n", "\n", list(
#> "internal"), "\n"), `MulticoreFuture-class.Rd` = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("MulticoreFuture-class"), "\n", list("MulticoreFuture-class"),
#> "\n", list("resolved.MulticoreFuture"), "\n", list(
#> "A multicore future is a future whose value will be resolved asynchronously in a parallel process"),
#> "\n", list("\n", list(list("resolved"), list("MulticoreFuture")),
#> "(x, run = TRUE, timeout = NULL, ...)\n"), "\n",
#> list("\n", list("MulticoreFuture()"), " returns an object of class ",
#> list("MulticoreFuture"), ".\n"), "\n", list("\n",
#> "A multicore future is a future whose value will be resolved asynchronously in a parallel process\n"),
#> "\n", list(list("Usage"), list("\n", "\n", "To use 'multicore' futures, use ",
#> list("plan(multicore, ...)"), ", cf. ", list(
#> "multicore"), ".\n")), "\n", "\n", list("internal"),
#> "\n"), `MultiprocessFuture-class.Rd` = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in", "\n", "% R/backend_api-03.MultiprocessFutureBackend-class.R",
#> "\n", list("MultiprocessFuture-class"), "\n", list(
#> "MultiprocessFuture-class"), "\n", list("MultiprocessFuture"),
#> "\n", list("A multiprocess future is a future whose value will be resolved asynchronously in a parallel process"),
#> "\n", list("\n", "MultiprocessFuture(expr = NULL, substitute = TRUE, envir = parent.frame(), ...)\n"),
#> "\n", list("\n", list(list("expr"), list("An ", list(),
#> " ", list("expression"), ".")), "\n", "\n", list(
#> list("substitute"), list("If TRUE, argument ",
#> list("expr"), " is\n", list(list("substitute"),
#> "()"), ":ed, otherwise not.")), "\n", "\n",
#> list(list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n", "identified.")),
#> "\n", "\n", list(list(list()), list("Additional named elements passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("MultiprocessFuture()"), " returns an object of class ",
#> list("MultiprocessFuture"), ".\n"), "\n", list(
#> "\n", "A multiprocess future is a future whose value will be resolved asynchronously in a parallel process\n"),
#> "\n", list("internal"), "\n"), `UniprocessFuture-class.Rd` = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/backend_api-UniprocessFuture-class.R",
#> "\n", list("UniprocessFuture-class"), "\n", list(
#> "UniprocessFuture-class"), "\n", list("UniprocessFuture"),
#> "\n", list("An uniprocess future is a future whose value will be resolved synchronously in the current process"),
#> "\n", list("\n", "UniprocessFuture(expr = NULL, substitute = TRUE, envir = parent.frame(), ...)\n"),
#> "\n", list("\n", list(list("expr"), list("An ", list(),
#> " ", list("expression"), ".")), "\n", "\n", list(
#> list("substitute"), list("If TRUE, argument ",
#> list("expr"), " is\n", list(list("substitute"),
#> "()"), ":ed, otherwise not.")), "\n", "\n",
#> list(list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n", "identified.")),
#> "\n", "\n", list(list(list()), list("Additional named elements passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("UniprocessFuture()"), " returns an object of class ",
#> list("UniprocessFuture"), ".\n"), "\n", list(
#> "\n", "An uniprocess future is a future whose value will be resolved synchronously in the current process\n"),
#> "\n", list("internal"), "\n"), backtrace.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/utils_api-backtrace.R",
#> "\n", list("backtrace"), "\n", list("backtrace"),
#> "\n", list("Back trace the expressions evaluated when an error was caught"),
#> "\n", list("\n", "backtrace(future, envir = parent.frame(), ...)\n"),
#> "\n", list("\n", list(list("future"), list("A future with a caught error.")),
#> "\n", "\n", list(list("envir"), list("the environment where to locate the future.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A list with the future's call stack that led up to the error.\n"),
#> "\n", list("\n", "Back trace the expressions evaluated when an error was caught\n"),
#> "\n", list("\n", "my_log <- function(x) log(x)\n",
#> "foo <- function(...) my_log(...)\n", "\n", "f <- future({ foo(\"a\") })\n",
#> "res <- tryCatch({\n", " v <- value(f)\n", "}, error = function(ex) {\n",
#> " t <- backtrace(f)\n", " print(t)\n", "})\n",
#> list("\n", "## R CMD check: make sure any open connections are closed afterward\n",
#> "plan(sequential)\n"), "\n", "\n"), "\n"),
#> cancel.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-cancel.R",
#> "\n", list("cancel"), "\n", list("cancel"), "\n",
#> list("Cancel a future"), "\n", list("\n", "cancel(x, interrupt = TRUE, ...)\n"),
#> "\n", list("\n", list(list("x"), list("A Future.")),
#> "\n", "\n", list(list("interrupt"), list("If TRUE, running futures are interrupted, if the\n",
#> "future backend supports it.")), "\n", "\n",
#> list(list(list()), list("All arguments used by the S3 methods.")),
#> "\n"), "\n", list("\n", list("cancel()"), " returns (invisibly) the canceled ",
#> list("Future"), "s after\n", "flagging them as \"canceled\" and possibly interrupting them as well.\n",
#> "\n", "Canceling a lazy or a finished future has no effect.\n"),
#> "\n", list("\n", "Cancels futures, with the option to interrupt running ones.\n"),
#> "\n", list("\n", list("if ((interactive() || .Platform[[\"OS.type\"]] != \"windows\")) (if (getRversion() >= \"3.4\") withAutoprint else force)({ # examplesIf"),
#> "\n", "## Set up two parallel workers\n", "plan(multisession, workers = 2)\n",
#> "\n", "## Launch two long running future\n",
#> "fs <- lapply(c(1, 2), function(duration) {\n",
#> " future({\n", " Sys.sleep(duration)\n",
#> " 42\n", " })\n", "})\n", "\n", "## Wait until at least one of the futures is resolved\n",
#> "while (!any(resolved(fs))) Sys.sleep(0.1)\n",
#> "\n", "## Cancel the future that is not yet resolved\n",
#> "r <- resolved(fs)\n", "cancel(fs[!r])\n",
#> "\n", "## Get the value of the resolved future\n",
#> "f <- fs[r]\n", "v <- value(f)\n", "message(\"Result: \", v)\n",
#> "\n", "## The value of the canceled future is an error\n",
#> "try(v <- value(fs[!r]))\n", "\n", "## Shut down parallel workers\n",
#> "plan(sequential)\n", list("}) # examplesIf"),
#> "\n"), "\n", list("\n", "A canceled future can be ",
#> list(list("reset()")), " to a lazy, vanilla future\n",
#> "such that it can be relaunched, possible on another future backend.\n"),
#> "\n"), cluster.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.ClusterFutureBackend-class.R",
#> "\n", list("cluster"), "\n", list("cluster"),
#> "\n", list("Create a cluster future whose value will be resolved asynchronously in a parallel process"),
#> "\n", list("\n", "cluster(\n", " ...,\n", " workers = availableWorkers(constraints = \"connections\"),\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " persistent = FALSE,\n", " envir = parent.frame()\n",
#> ")\n"), "\n", list("\n", list(list("workers"),
#> list("A ", list(list("cluster")), " object,\n",
#> "a character vector of host names, a positive numeric scalar,\n",
#> "or a function.\n", "If a character vector or a numeric scalar, a ",
#> list("cluster"), " object\n", "is created using ",
#> list(list("makeClusterPSOCK"), "(workers)"),
#> ".\n", "If a function, it is called without arguments ",
#> list("when the future\n", "is created"),
#> " and its value is used to configure the workers.\n",
#> "The function should return any of the above types.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("persistent"), list("If FALSE, the evaluation environment is cleared\n",
#> "from objects prior to the evaluation of the future.")),
#> "\n", "\n", list(list("envir"), list("The ",
#> list("environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(list()),
#> list("Additional named elements passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A ClusterFuture.\n"), "\n", list(
#> "\n", list("WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A cluster future is a future that uses cluster evaluation,\n",
#> "which means that its ", list("value is computed and resolved in\n",
#> "parallel in another process"), ".\n", "\n",
#> "This function is must ", list("not"), " be called directly. Instead, the\n",
#> "typical usages are:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures via a single background R process on the local machine\n",
#> "plan(cluster, workers = I(1))\n", "\n",
#> "# Evaluate futures via two background R processes on the local machine\n",
#> "plan(cluster, workers = 2)\n", "\n", "# Evaluate futures via a single R process on another machine on on the\n",
#> "# local area network (LAN)\n", "plan(cluster, workers = \"raspberry-pi\")\n",
#> "\n", "# Evaluate futures via a single R process running on a remote machine\n",
#> "plan(cluster, workers = \"pi.example.org\")\n",
#> "\n", "# Evaluate futures via four R processes, one running on the local machine,\n",
#> "# two running on LAN machine 'n1' and one on a remote machine\n",
#> "plan(cluster, workers = c(\"localhost\", \"n1\", \"n1\", \"pi.example.org\"))\n"),
#> list(list("html"), list(list("</div>"))), "\n"),
#> "\n", list("\n", list("\n", "\n", "## Use cluster futures\n",
#> "cl <- parallel::makeCluster(2, timeout = 60)\n",
#> "plan(cluster, workers = cl)\n", "\n", "## A global variable\n",
#> "a <- 0\n", "\n", "## Create future (explicitly)\n",
#> "f <- future({\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "\n", "## A cluster future is evaluated in a separate process.\n",
#> "## Regardless, changing the value of a global variable will\n",
#> "## not affect the result of the future.\n",
#> "a <- 7\n", "print(a)\n", "\n", "v <- value(f)\n",
#> "print(v)\n", "stopifnot(v == 0)\n", "\n",
#> "## CLEANUP\n", "parallel::stopCluster(cl)\n",
#> "\n"), "\n"), "\n", list("\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n"), "\n"), clusterExportSticky.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-sticky_globals.R",
#> "\n", list("clusterExportSticky"), "\n", list(
#> "clusterExportSticky"), "\n", list("Export globals to the sticky-globals environment of the cluster nodes"),
#> "\n", list("\n", "clusterExportSticky(cl, globals)\n"),
#> "\n", list("\n", list(list("cl"), list("(cluster) A cluster object as returned by\n",
#> list(list("parallel::makeCluster()")), ".")),
#> "\n", "\n", list(list("globals"), list("(list) A named list of sticky globals to be exported.")),
#> "\n"), "\n", list("\n", "(invisible; cluster) The cluster object.\n"),
#> "\n", list("\n", "Export globals to the sticky-globals environment of the cluster nodes\n"),
#> "\n", list("\n", "This requires that the ", list(
#> "future"), " package is installed on the cluster\n",
#> "nodes.\n"), "\n", list("internal"), "\n"),
#> find_references.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-marshalling.R",
#> "\n", list("find_references"), "\n", list("find_references"),
#> "\n", list("assert_no_references"), "\n", list(
#> "Get the first or all references of an ", list(),
#> " object"), "\n", list("\n", "find_references(x, first_only = FALSE)\n",
#> "\n", "assert_no_references(\n", " x,\n",
#> " action = c(\"error\", \"warning\", \"message\", \"string\"),\n",
#> " source = c(\"globals\", \"value\")\n", ")\n"),
#> "\n", list("\n", list(list("x"), list("The ",
#> list(), " object to be checked.")), "\n", "\n",
#> list(list("first_only"), list("If ", list("TRUE"),
#> ", only the first reference is returned,\n",
#> "otherwise all references.")), "\n", "\n",
#> list(list("action"), list("Type of action to take if a reference is found.")),
#> "\n", "\n", list(list("source"), list("Is the source of ",
#> list("x"), " the globals or the value of the future?")),
#> "\n"), "\n", list("\n", list("find_references()"),
#> " returns a list of zero or more references\n",
#> "identified.\n", "\n", "If a reference is detected, an informative error, warning, message,\n",
#> "or a character string is produced, otherwise ",
#> list("NULL"), " is returned\n", "invisibly.\n"),
#> "\n", list("\n", "Get the first or all references of an ",
#> list(), " object\n", "\n", "Assert that there are no references among the identified globals\n"),
#> "\n", list("internal"), "\n"), future.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-future.R, R/utils_api-futureCall.R,",
#> "\n", "% R/utils_api-minifuture.R", "\n", list(
#> "future"), "\n", list("future"), "\n", list(
#> "futureCall"), "\n", list("minifuture"), "\n",
#> list("Create a future"), "\n", list("\n", "future(\n",
#> " expr,\n", " envir = parent.frame(),\n",
#> " substitute = TRUE,\n", " lazy = FALSE,\n",
#> " seed = FALSE,\n", " globals = TRUE,\n",
#> " packages = NULL,\n", " stdout = TRUE,\n",
#> " conditions = \"condition\",\n", " label = NULL,\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " ...\n", ")\n", "\n", "futureCall(\n", " FUN,\n",
#> " args = list(),\n", " envir = parent.frame(),\n",
#> " lazy = FALSE,\n", " seed = FALSE,\n", " globals = TRUE,\n",
#> " packages = NULL,\n", " stdout = TRUE,\n",
#> " conditions = \"condition\",\n", " earlySignal = FALSE,\n",
#> " label = NULL,\n", " gc = FALSE,\n", " ...\n",
#> ")\n", "\n", "minifuture(\n", " expr,\n",
#> " substitute = TRUE,\n", " globals = NULL,\n",
#> " packages = NULL,\n", " stdout = NA,\n",
#> " conditions = NULL,\n", " seed = NULL,\n",
#> " ...,\n", " envir = parent.frame()\n", ")\n"),
#> "\n", list("\n", list(list("expr"), list("An ",
#> list(), " ", list("expression"), ".")), "\n",
#> "\n", list(list("envir"), list("The ", list(
#> "environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list("substitute"),
#> list("If TRUE, argument ", list("expr"),
#> " is\n", list(list("substitute"), "()"),
#> ":ed, otherwise not.")), "\n", "\n", list(
#> list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("seed"), list("(optional) If TRUE, the random seed, that is, the state of the\n",
#> "random number generator (RNG) will be set such that statistically sound\n",
#> "random numbers are produced (also during parallelization).\n",
#> "If FALSE (default), it is assumed that the future expression does neither\n",
#> "need nor use random numbers generation.\n",
#> "To use a fixed random seed, specify a L'Ecuyer-CMRG seed (seven integer)\n",
#> "or a regular RNG seed (a single integer). If the latter, then a\n",
#> "L'Ecuyer-CMRG seed will be automatically created based on the given seed.\n",
#> "Furthermore, if FALSE, then the future will be monitored to make sure it\n",
#> "does not use random numbers. If it does and depending on the value of\n",
#> "option ", list("future.rng.onMisuse"), ", the check is\n",
#> "ignored, an informative warning, or error will be produced.\n",
#> "If ", list("seed"), " is NULL, then the effect is as with ",
#> list("seed = FALSE"), "\n", "but without the RNG check being performed.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, or a named list\n",
#> "to control how globals are handled.\n",
#> "For details, see section 'Globals used by future expressions'\n",
#> "in the help for ", list(list("future()")),
#> ".")), "\n", "\n", list(list("packages"),
#> list("(optional) a character vector specifying packages\n",
#> "to be attached in the ", list(), " environment evaluating the future.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE (default), then the standard output is captured,\n",
#> "and re-outputted when ", list("value()"),
#> " is called.\n", "If FALSE, any output is silenced (by sinking it to the null device as\n",
#> "it is outputted).\n", "Using ", list("stdout = structure(TRUE, drop = TRUE)"),
#> " causes the captured\n", "standard output to be dropped from the future object as soon as it has\n",
#> "been relayed. This can help decrease the overall memory consumed by\n",
#> "captured output across futures.\n", "Using ",
#> list("stdout = NA"), " fully avoids intercepting the standard output;\n",
#> "behavior of such unhandled standard output depends on the future backend.")),
#> "\n", "\n", list(list("conditions"), list("A character string of conditions classes to be captured\n",
#> "and relayed. The default is to relay all conditions, including messages\n",
#> "and warnings. To drop all conditions, use ",
#> list("conditions = character(0)"), ".\n",
#> "Errors are always relayed.\n", "Attribute ",
#> list("exclude"), " can be used to ignore specific classes, e.g.\n",
#> list("conditions = structure(\"condition\", exclude = \"message\")"),
#> " will capture\n", "all ", list("condition"),
#> " classes except those that inherits from the ",
#> list("message"), " class.\n", "Using ", list(
#> "conditions = structure(..., drop = TRUE)"),
#> " causes any captured\n", "conditions to be dropped from the future object as soon as it has\n",
#> "been relayed, e.g. by ", list("value(f)"),
#> ". This can help decrease the overall\n",
#> "memory consumed by captured conditions across futures.\n",
#> "Using ", list("conditions = NULL"), " (not recommended) avoids intercepting conditions,\n",
#> "except from errors; behavior of such unhandled conditions depends on the\n",
#> "future backend and the environment from which R runs.")),
#> "\n", "\n", list(list("label"), list("A character string label attached to the future.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("FUN"), list("A ", list("function"),
#> " to be evaluated.")), "\n", "\n", list(
#> list("args"), list("A ", list("list"), " of arguments passed to function ",
#> list("FUN"), ".")), "\n", "\n", list(list(
#> list()), list("Additional arguments passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("future()"), " returns ", list(
#> "Future"), " that evaluates expression ", list(
#> "expr"), ".\n", "\n", list("futureCall()"),
#> " returns a ", list("Future"), " that calls function ",
#> list("FUN"), " with\n", "arguments ", list(
#> "args"), ".\n", "\n", list("minifuture(expr)"),
#> " creates a future with minimal overhead, by disabling\n",
#> "user-friendly behaviors, e.g. automatic identification of global\n",
#> "variables and packages needed, and relaying of output. Unless you have\n",
#> "good reasons for using this function, please use ",
#> list(list("future()")), " instead.\n", "This function exists mainly for the purpose of profiling and identifying\n",
#> "which automatic features of ", list(list("future()")),
#> " introduce extra overhead.\n"), "\n", list(
#> "\n", list(list("html"), list(list(list("logo.png"),
#> list("options: style='float: right;' alt='logo' width='120'")))),
#> "\n", "Creates a future that evaluates an ",
#> list(), " expression or\n", "a future that calls an ",
#> list(), " function with a set of arguments.\n",
#> "How, when, and where these futures are evaluated can be configured\n",
#> "using ", list(list("plan()")), " such that it is evaluated in parallel on,\n",
#> "for instance, the current machine, on a remote machine, or via a\n",
#> "job queue on a compute cluster.\n", "Importantly, any ",
#> list(), " code using futures remains the same regardless\n",
#> "on these settings and there is no need to modify the code when\n",
#> "switching from, say, sequential to parallel processing.\n"),
#> "\n", list("\n", "The state of a future is either unresolved or resolved.\n",
#> "The value of a future can be retrieved using ",
#> list("v <- ", list("value"), "(f)"), ".\n",
#> "Querying the value of a non-resolved future will ",
#> list("block"), " the call\n", "until the future is resolved.\n",
#> "It is possible to check whether a future is resolved or not\n",
#> "without blocking by using ", list(list("resolved"),
#> "(f)"), ".\n", "It is possible to ", list(
#> list("cancel()")), " a future that is being resolved.\n",
#> "Failed, canceled, and interrupted futures can be ",
#> list(list("reset()")), " to a\n", "lazy, vanilla future that can be relaunched.\n",
#> "\n", "The ", list("futureCall()"), " function works analogously to\n",
#> list(list("do.call"), "()"), ", which calls a function with a set of\n",
#> "arguments. The difference is that ", list(
#> "do.call()"), " returns the value of\n",
#> "the call whereas ", list("futureCall()"),
#> " returns a future.\n"), "\n", list(list("Eager or lazy evaluation"),
#> list("\n", "\n", "By default, a future is resolved using ",
#> list("eager"), " evaluation\n", "(", list(
#> "lazy = FALSE"), "). This means that the expression starts to\n",
#> "be evaluated as soon as the future is created.\n",
#> "\n", "As an alternative, the future can be resolved using ",
#> list("lazy"), "\n", "evaluation (", list(
#> "lazy = TRUE"), "). This means that the expression\n",
#> "will only be evaluated when the value of the future is requested.\n",
#> list("Note that this means that the expression may not be evaluated\n",
#> "at all - it is guaranteed to be evaluated if the value is requested"),
#> ".\n")), "\n", "\n", list(list("Globals used by future expressions"),
#> list("\n", "\n", "Global objects (short ",
#> list("globals"), ") are objects (e.g. variables and\n",
#> "functions) that are needed in order for the future expression to be\n",
#> "evaluated while not being local objects that are defined by the future\n",
#> "expression. For example, in\n", list("\n",
#> " a <- 42\n", " f <- future({ b <- 2; a * b })\n"),
#> "\n", "variable ", list("a"), " is a global of future assignment ",
#> list("f"), " whereas\n", list("b"), " is a local variable.\n",
#> "In order for the future to be resolved successfully (and correctly),\n",
#> "all globals need to be gathered when the future is created such that\n",
#> "they are available whenever and wherever the future is resolved.\n",
#> "\n", "The default behavior (", list("globals = TRUE"),
#> "),\n", "is that globals are automatically identified and gathered.\n",
#> "More precisely, globals are identified via code inspection of the\n",
#> "future expression ", list("expr"), " and their values are retrieved with\n",
#> "environment ", list("envir"), " as the starting point (basically via\n",
#> list("get(global, envir = envir, inherits = TRUE)"),
#> ").\n", list("In most cases, such automatic collection of globals is sufficient\n",
#> "and less tedious and error prone than if they are manually specified"),
#> ".\n", "\n", "However, for full control, it is also possible to explicitly specify\n",
#> "exactly which the globals are by providing their names as a character\n",
#> "vector.\n", "In the above example, we could use\n",
#> list("\n", " a <- 42\n", " f <- future({ b <- 2; a * b }, globals = \"a\")\n"),
#> "\n", "\n", "Yet another alternative is to explicitly specify also their values\n",
#> "using a named list as in\n", list("\n",
#> " a <- 42\n", " f <- future({ b <- 2; a * b }, globals = list(a = a))\n"),
#> "\n", "or\n", list("\n", " f <- future({ b <- 2; a * b }, globals = list(a = 42))\n"),
#> "\n", "\n", "Specifying globals explicitly avoids the overhead added from\n",
#> "automatically identifying the globals and gathering their values.\n",
#> "Furthermore, if we know that the future expression does not make use\n",
#> "of any global variables, we can disable the automatic search for\n",
#> "globals by using\n", list("\n", " f <- future({ a <- 42; b <- 2; a * b }, globals = FALSE)\n"),
#> "\n", "\n", "Future expressions often make use of functions from one or more packages.\n",
#> "As long as these functions are part of the set of globals, the future\n",
#> "package will make sure that those packages are attached when the future\n",
#> "is resolved. Because there is no need for such globals to be frozen\n",
#> "or exported, the future package will not export them, which reduces\n",
#> "the amount of transferred objects.\n", "For example, in\n",
#> list("\n", " x <- rnorm(1000)\n", " f <- future({ median(x) })\n"),
#> "\n", "variable ", list("x"), " and ", list(
#> "median()"), " are globals, but only ",
#> list("x"), "\n", "is exported whereas ",
#> list("median()"), ", which is part of the ",
#> list("stats"), "\n", "package, is not exported. Instead it is made sure that the ",
#> list("stats"), "\n", "package is on the search path when the future expression is evaluated.\n",
#> "Effectively, the above becomes\n", list(
#> "\n", " x <- rnorm(1000)\n", " f <- future({\n",
#> " library(stats)\n", " median(x)\n",
#> " })\n"), "\n", "To manually specify this, one can either do\n",
#> list("\n", " x <- rnorm(1000)\n", " f <- future({\n",
#> " median(x)\n", " }, globals = list(x = x, median = stats::median)\n"),
#> "\n", "or\n", list("\n", " x <- rnorm(1000)\n",
#> " f <- future({\n", " library(stats)\n",
#> " median(x)\n", " }, globals = list(x = x))\n"),
#> "\n", "Both are effectively the same.\n",
#> "\n", "Although rarely needed, a combination of automatic identification and manual\n",
#> "specification of globals is supported via attributes ",
#> list("add"), " (to add\n", "false negatives) and ",
#> list("ignore"), " (to ignore false positives) on value\n",
#> list("TRUE"), ". For example, with\n", list(
#> "globals = structure(TRUE, ignore = \"b\", add = \"a\")"),
#> " any globals\n", "automatically identified, except ",
#> list("b"), ", will be used, in addition to\n",
#> "global ", list("a"), ".\n")), "\n", "\n",
#> list("\n", "## Evaluate futures in parallel\n",
#> "plan(multisession)\n", "\n", "## Data\n",
#> "x <- rnorm(100)\n", "y <- 2 * x + 0.2 + rnorm(100)\n",
#> "w <- 1 + x ^ 2\n", "\n", "\n", "## EXAMPLE: Regular assignments (evaluated sequentially)\n",
#> "fitA <- lm(y ~ x, weights = w) ## with offset\n",
#> "fitB <- lm(y ~ x - 1, weights = w) ## without offset\n",
#> "fitC <- {\n", " w <- 1 + abs(x) ## Different weights\n",
#> " lm(y ~ x, weights = w)\n", "}\n", "print(fitA)\n",
#> "print(fitB)\n", "print(fitC)\n", "\n", "\n",
#> "## EXAMPLE: Future assignments (evaluated in parallel)\n",
#> "fitA %<-% lm(y ~ x, weights = w) ## with offset\n",
#> "fitB %<-% lm(y ~ x - 1, weights = w) ## without offset\n",
#> "fitC %<-% {\n", " w <- 1 + abs(x)\n", " lm(y ~ x, weights = w)\n",
#> "}\n", "print(fitA)\n", "print(fitB)\n", "print(fitC)\n",
#> "\n", "\n", "## EXAMPLE: Explicitly create futures (evaluated in parallel)\n",
#> "## and retrieve their values\n", "fA <- future( lm(y ~ x, weights = w) )\n",
#> "fB <- future( lm(y ~ x - 1, weights = w) )\n",
#> "fC <- future({\n", " w <- 1 + abs(x)\n",
#> " lm(y ~ x, weights = w)\n", "})\n", "fitA <- value(fA)\n",
#> "fitB <- value(fB)\n", "fitC <- value(fC)\n",
#> "print(fitA)\n", "print(fitB)\n", "print(fitC)\n",
#> "\n", list("\n", "## Make sure to \"close\" an multisession workers on Windows\n",
#> "plan(sequential)\n"), "\n", "## EXAMPLE: futureCall() and do.call()\n",
#> "x <- 1:100\n", "y0 <- do.call(sum, args = list(x))\n",
#> "print(y0)\n", "\n", "f1 <- futureCall(sum, args = list(x))\n",
#> "y1 <- value(f1)\n", "print(y1)\n"), "\n",
#> list("\n", "How, when and where futures are resolved is given by the\n",
#> list("future backend"), ", which can be set by the end user using the\n",
#> list(list("plan()")), " function.\n"), "\n",
#> list("\n", "The future logo was designed by Dan LaBar and tweaked by Henrik Bengtsson.\n"),
#> "\n"), futureAssign.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/delayed_api-futureAssign.R,",
#> "\n", "% R/infix_api-01-futureAssign_OP.R, R/infix_api-02-globals_OP.R,",
#> "\n", "% R/infix_api-03-seed_OP.R, R/infix_api-04-stdout_OP.R,",
#> "\n", "% R/infix_api-05-conditions_OP.R, R/infix_api-06-lazy_OP.R,",
#> "\n", "% R/infix_api-07-label_OP.R, R/infix_api-08-plan_OP.R,",
#> "\n", "% R/infix_api-09-tweak_OP.R", "\n",
#> list("futureAssign"), "\n", list("futureAssign"),
#> "\n", list("%<-%"), "\n", list("%->%"), "\n",
#> list("%globals%"), "\n", list("%packages%"),
#> "\n", list("%seed%"), "\n", list("%stdout%"),
#> "\n", list("%conditions%"), "\n", list("%lazy%"),
#> "\n", list("%label%"), "\n", list("%plan%"),
#> "\n", list("%tweak%"), "\n", list("Create a future assignment"),
#> "\n", list("\n", "futureAssign(\n", " x,\n",
#> " value,\n", " envir = parent.frame(),\n",
#> " substitute = TRUE,\n", " lazy = FALSE,\n",
#> " seed = FALSE,\n", " globals = TRUE,\n",
#> " packages = NULL,\n", " stdout = TRUE,\n",
#> " conditions = \"condition\",\n", " earlySignal = FALSE,\n",
#> " label = NULL,\n", " gc = FALSE,\n", " ...,\n",
#> " assign.env = envir\n", ")\n", "\n", "x %<-% value\n",
#> "\n", "fassignment %globals% globals\n", "fassignment %packages% packages\n",
#> "\n", "fassignment %seed% seed\n", "\n", "fassignment %stdout% capture\n",
#> "\n", "fassignment %conditions% capture\n",
#> "\n", "fassignment %lazy% lazy\n", "\n", "fassignment %label% label\n",
#> "\n", "fassignment %plan% strategy\n", "\n",
#> "fassignment %tweak% tweaks\n"), "\n", list(
#> "\n", list(list("x"), list("the name of a future variable, which will hold the value\n",
#> "of the future expression (as a promise).")),
#> "\n", "\n", list(list("value"), list("An ",
#> list(), " ", list("expression"), ".")), "\n",
#> "\n", list(list("envir"), list("The ", list(
#> "environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list("substitute"),
#> list("If TRUE, argument ", list("expr"),
#> " is\n", list(list("substitute"), "()"),
#> ":ed, otherwise not.")), "\n", "\n", list(
#> list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("seed"), list("(optional) If TRUE, the random seed, that is, the state of the\n",
#> "random number generator (RNG) will be set such that statistically sound\n",
#> "random numbers are produced (also during parallelization).\n",
#> "If FALSE (default), it is assumed that the future expression does neither\n",
#> "need nor use random numbers generation.\n",
#> "To use a fixed random seed, specify a L'Ecuyer-CMRG seed (seven integer)\n",
#> "or a regular RNG seed (a single integer). If the latter, then a\n",
#> "L'Ecuyer-CMRG seed will be automatically created based on the given seed.\n",
#> "Furthermore, if FALSE, then the future will be monitored to make sure it\n",
#> "does not use random numbers. If it does and depending on the value of\n",
#> "option ", list("future.rng.onMisuse"), ", the check is\n",
#> "ignored, an informative warning, or error will be produced.\n",
#> "If ", list("seed"), " is NULL, then the effect is as with ",
#> list("seed = FALSE"), "\n", "but without the RNG check being performed.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, or a named list\n",
#> "to control how globals are handled.\n",
#> "For details, see section 'Globals used by future expressions'\n",
#> "in the help for ", list(list("future()")),
#> ".")), "\n", "\n", list(list("packages"),
#> list("(optional) a character vector specifying packages\n",
#> "to be attached in the ", list(), " environment evaluating the future.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE (default), then the standard output is captured,\n",
#> "and re-outputted when ", list("value()"),
#> " is called.\n", "If FALSE, any output is silenced (by sinking it to the null device as\n",
#> "it is outputted).\n", "Using ", list("stdout = structure(TRUE, drop = TRUE)"),
#> " causes the captured\n", "standard output to be dropped from the future object as soon as it has\n",
#> "been relayed. This can help decrease the overall memory consumed by\n",
#> "captured output across futures.\n", "Using ",
#> list("stdout = NA"), " fully avoids intercepting the standard output;\n",
#> "behavior of such unhandled standard output depends on the future backend.")),
#> "\n", "\n", list(list("conditions"), list("A character string of conditions classes to be captured\n",
#> "and relayed. The default is to relay all conditions, including messages\n",
#> "and warnings. To drop all conditions, use ",
#> list("conditions = character(0)"), ".\n",
#> "Errors are always relayed.\n", "Attribute ",
#> list("exclude"), " can be used to ignore specific classes, e.g.\n",
#> list("conditions = structure(\"condition\", exclude = \"message\")"),
#> " will capture\n", "all ", list("condition"),
#> " classes except those that inherits from the ",
#> list("message"), " class.\n", "Using ", list(
#> "conditions = structure(..., drop = TRUE)"),
#> " causes any captured\n", "conditions to be dropped from the future object as soon as it has\n",
#> "been relayed, e.g. by ", list("value(f)"),
#> ". This can help decrease the overall\n",
#> "memory consumed by captured conditions across futures.\n",
#> "Using ", list("conditions = NULL"), " (not recommended) avoids intercepting conditions,\n",
#> "except from errors; behavior of such unhandled conditions depends on the\n",
#> "future backend and the environment from which R runs.")),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("label"), list("A character string label attached to the future.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("assign.env"), list("The ",
#> list("environment"), " to which the variable\n",
#> "should be assigned.")), "\n", "\n", list(
#> list("fassignment"), list("The future assignment, e.g.\n",
#> list("x %<-% { expr }"), ".")), "\n", "\n",
#> list(list("capture"), list("If TRUE, the standard output will be captured, otherwise not.")),
#> "\n", "\n", list(list("strategy"), list("The backend controlling how the future is\n",
#> "resolved. See ", list(list("plan()")), " for further details.")),
#> "\n", "\n", list(list("tweaks"), list("A named list (or vector) with arguments that\n",
#> "should be changed relative to the current backend.")),
#> "\n", "\n", list(list(list()), list("Additional arguments passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("futureAssign()"), " and ", list(
#> "x %<-% expr"), " returns the ", list("Future"),
#> " invisibly,\n", "e.g. ", list("f <- futureAssign(\"x\", expr)"),
#> " and ", list("f <- (x %<-% expr)"), ".\n"),
#> "\n", list("\n", list("x %<-% value"), " (also known as a \"future assignment\") and\n",
#> list("futureAssign(\"x\", value)"), " create a ",
#> list("Future"), " that evaluates the expression\n",
#> "(", list("value"), ") and binds it to variable ",
#> list("x"), " (as a\n", list("promise"), "). The expression is evaluated in parallel\n",
#> "in the background. Later on, when ", list(
#> "x"), " is first queried, the value of future\n",
#> "is automatically retrieved as it were a regular variable and ",
#> list("x"), " is\n", "materialized as a regular value.\n"),
#> "\n", list("\n", "For a future created via a future assignment, ",
#> list("x %<-% value"), " or\n", list("futureAssign(\"x\", value)"),
#> ", the value is bound to a promise, which when\n",
#> "queried will internally call ", list(list(
#> "value()")), " on the future and which will then\n",
#> "be resolved into a regular variable bound to that value. For example, with\n",
#> "future assignment ", list("x %<-% value"),
#> ", the first time variable ", list("x"), " is queried\n",
#> "the call blocks if, and only if, the future is not yet resolved. As soon\n",
#> "as it is resolved, and any succeeding queries, querying ",
#> list("x"), " will\n", "immediately give the value.\n",
#> "\n", "The future assignment construct ", list(
#> "x %<-% value"), " is not a formal assignment\n",
#> "per se, but a binary infix operator on objects ",
#> list("x"), " and expression ", list("value"),
#> ".\n", "However, by using non-standard evaluation, this constructs can emulate an\n",
#> "assignment operator similar to ", list("x <- value"),
#> ". Due to ", list(), "'s precedence rules\n",
#> "of operators, future expressions often need to be explicitly bracketed,\n",
#> "e.g. ", list("x %<-% { a + b }"), ".\n"),
#> "\n", list(list("Adjust future arguments of a future assignment"),
#> list("\n", "\n", "\n", list(list("future()")),
#> " and ", list(list("futureAssign()")), " take several arguments that can be used\n",
#> "to explicitly specify what global variables and packages the future should\n",
#> "use. They can also be used to override default behaviors of the future,\n",
#> "e.g. whether output should be relayed or not. When using a future\n",
#> "assignment, these arguments can be specified via corresponding\n",
#> "assignment expression. For example, ",
#> list("x %<-% { rnorm(10) } %seed% TRUE"),
#> "\n", "corresponds to ", list("futureAssign(\"x\", { rnorm(10) }, seed = TRUE)"),
#> ". Here are\n", "a several examples.\n",
#> "\n", "To explicitly specify variables and functions that a future assignment\n",
#> "should use, use ", list("%globals%"), ". To explicitly specify which packages need\n",
#> "to be attached for the evaluate to success, use ",
#> list("%packages%"), ". For\n", "example,\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x <- rnorm(1000)\n", "> y %<-% { median(x) } %globals% list(x = x) %packages% \"stats\"\n",
#> "> y\n", "[1] -0.03956372\n"), list(list(
#> "html"), list(list("</div>"))), "\n", "\n",
#> "The ", list("median()"), " function is part of the 'stats' package.\n",
#> "\n", "To declare that you will generate random numbers, use ",
#> list("%seed%"), ", e.g.\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { rnorm(3) } %seed% TRUE\n",
#> "> x\n", "[1] -0.2590562 -1.2262495 0.8858702\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n", "\n", "To disable relaying of standard output (e.g. ",
#> list("print()"), ", ", list("cat()"), ", and\n",
#> list("str()"), "), while keeping relaying of conditions (e.g. ",
#> list("message()"), " and\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { cat(\"Hello\\n\"); message(\"Hi there\"); 42 } %stdout% FALSE\n",
#> "> y <- 13\n", "> z <- x + y\n", "Hi there\n",
#> "> z\n", "[1] 55\n"), list(list("html"),
#> list(list("</div>"))), "\n", "\n", "To disable relaying of conditions, use ",
#> list("%conditions%"), ", e.g.\n", "\n", list(
#> list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { cat(\"Hello\\n\"); message(\"Hi there\"); 42 } %conditions% character(0)\n",
#> "> y <- 13\n", "> z <- x + y\n", "Hello\n",
#> "> z\n", "[1] 55\n"), list(list("html"),
#> list(list("</div>"))), "\n", "\n", list(
#> list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { print(1:10); message(\"Hello\"); 42 } %stdout% FALSE\n",
#> "> y <- 13\n", "> z <- x + y\n", "Hello\n",
#> "> z\n", "[1] 55\n"), list(list("html"),
#> list(list("</div>"))), "\n", "\n", "To create a future without launching in such that it will only be\n",
#> "processed if the value is really needed, use ",
#> list("%lazy%"), ", e.g.\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { Sys.sleep(5); 42 } %lazy% TRUE\n",
#> "> y <- sum(1:10)\n", "> system.time(z <- x + y)\n",
#> " user system elapsed \n", " 0.004 0.000 5.008\n",
#> "> z\n", "[1] 97\n"), list(list("html"),
#> list(list("</div>"))), "\n")), "\n", "\n",
#> list(list("Error handling"), list("\n", "\n",
#> "\n", "Because future assignments are promises, errors produced by the the\n",
#> "future expression will not be signaled until the value of the future is\n",
#> "requested. For example, if you create a future assignment that produce\n",
#> "an error, you will not be affected by the error until you \"touch\" the\n",
#> "future-assignment variable. For example,\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { stop(\"boom\") }\n", "> y <- sum(1:10)\n",
#> "> z <- x + y\n", "Error in eval(quote({ : boom\n"),
#> list(list("html"), list(list("</div>"))), "\n")),
#> "\n", "\n", list(list("Use alternative future backend for future assignment"),
#> list("\n", "\n", "\n", "Futures are evaluated on the future backend that the user has specified\n",
#> "by ", list(list("plan()")), ". With regular futures, we can temporarily use another future\n",
#> "backend by wrapping our code in ", list(
#> "with(plan(...), { ... }]"), ", or temporarily\n",
#> "inside a function using ", list("with(plan(...), local = TRUE)"),
#> ". To achieve the\n", "same for a specific future assignment, use ",
#> list("%plan%"), ", e.g.\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> plan(multisession)\n", "> x %<-% { 42 }\n",
#> "> y %<-% { 13 } %plan% sequential\n",
#> "> z <- x + y\n", "> z\n", "[1] 55\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n", "\n", "Here ", list("x"), " is resolved in the background via the ",
#> list("multisession"), " backend,\n", "whereas ",
#> list("y"), " is resolved sequentially in the main R session.\n")),
#> "\n", "\n", list(list("Getting the future object of a future assignment"),
#> list("\n", "\n", "\n", "The underlying ", list(
#> "Future"), " of a future variable ", list(
#> "x"), " can be retrieved without\n", "blocking using ",
#> list("f <- ", list("futureOf"), "(x)"), ", e.g.\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { stop(\"boom\") }\n", "> f_x <- futureOf(x)\n",
#> "> resolved(f_x)\n", "[1] TRUE\n", "> x\n",
#> "Error in eval(quote({ : boom\n", "> value(f_x)\n",
#> "Error in eval(quote({ : boom\n"), list(
#> list("html"), list(list("</div>"))), "\n",
#> "\n", "Technically, both the future and the variable (promise) are assigned at\n",
#> "the same time to environment ", list("assign.env"),
#> " where the name of the future is\n", list(
#> ".future_<name>"), ".\n")), "\n", "\n"),
#> futureOf.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/delayed_api-futureOf.R",
#> "\n", list("futureOf"), "\n", list("futureOf"),
#> "\n", list("Get the future of a future variable"),
#> "\n", list("\n", "futureOf(\n", " var = NULL,\n",
#> " envir = parent.frame(),\n", " mustExist = TRUE,\n",
#> " default = NA,\n", " drop = FALSE\n", ")\n"),
#> "\n", list("\n", list(list("var"), list("the variable. If NULL, all futures in the\n",
#> "environment are returned.")), "\n", "\n",
#> list(list("envir"), list("the environment where to search from.")),
#> "\n", "\n", list(list("mustExist"), list("If TRUE and the variable does not exists, then\n",
#> "an informative error is thrown, otherwise NA is returned.")),
#> "\n", "\n", list(list("default"), list("the default value if future was not found.")),
#> "\n", "\n", list(list("drop"), list("if TRUE and ",
#> list("var"), " is NULL, then returned list\n",
#> "only contains futures, otherwise also ",
#> list("default"), " values.")), "\n"), "\n",
#> list("\n", "A ", list("Future"), " (or ", list(
#> "default"), ").\n", "If ", list("var"), " is NULL, then a named list of Future:s are returned.\n"),
#> "\n", list("\n", "Get the future of a future variable that has been created directly\n",
#> "or indirectly via ", list(list("future()")),
#> ".\n"), "\n", list("\n", "a %<-% { 1 }\n",
#> "\n", "f <- futureOf(a)\n", "print(f)\n", "\n",
#> "b %<-% { 2 }\n", "\n", "f <- futureOf(b)\n",
#> "print(f)\n", "\n", "## All futures\n", "fs <- futureOf()\n",
#> "print(fs)\n", "\n", "\n", "## Futures part of environment\n",
#> "env <- new.env()\n", "env$c %<-% { 3 }\n",
#> "\n", "f <- futureOf(env$c)\n", "print(f)\n",
#> "\n", "f2 <- futureOf(c, envir = env)\n", "print(f2)\n",
#> "\n", "f3 <- futureOf(\"c\", envir = env)\n",
#> "print(f3)\n", "\n", "fs <- futureOf(envir = env)\n",
#> "print(fs)\n"), "\n"), futureSessionInfo.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-futureSessionInfo.R",
#> "\n", list("futureSessionInfo"), "\n", list("futureSessionInfo"),
#> "\n", list("Get future-specific session information and validate current backend"),
#> "\n", list("\n", "futureSessionInfo(test = TRUE, anonymize = TRUE)\n"),
#> "\n", list("\n", list(list("test"), list("If TRUE, one or more futures are created to query workers\n",
#> "and validate their information.")), "\n",
#> "\n", list(list("anonymize"), list("If TRUE, user names and host names are anonymized.")),
#> "\n"), "\n", list("\n", "Nothing.\n"), "\n",
#> list("\n", "Get future-specific session information and validate current backend\n"),
#> "\n", list("\n", "plan(multisession, workers = 2)\n",
#> "futureSessionInfo()\n", "plan(sequential)\n"),
#> "\n"), futures.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-futures.R",
#> "\n", list("futures"), "\n", list("futures"),
#> "\n", list("Get all futures in a container"),
#> "\n", list("\n", "futures(x, ...)\n"), "\n",
#> list("\n", list(list("x"), list("An environment, a list, or a list environment.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "An object of same type as ",
#> list("x"), " and with the same names\n", "and/or dimensions, if set.\n"),
#> "\n", list("\n", "Gets all futures in an environment, a list, or a list environment\n",
#> "and returns an object of the same class (and dimensions).\n",
#> "Non-future elements are returned as is.\n"),
#> "\n", list("\n", "This function is useful for retrieve futures that were created via\n",
#> "future assignments (", list("%<-%"), ") and therefore stored as promises.\n",
#> "This function turns such promises into standard ",
#> list("Future"), "\n", "objects.\n"), "\n"),
#> getExpression.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("getExpression"), "\n", list("getExpression"),
#> "\n", list("getExpression.Future"), "\n", list(
#> "Inject code for the next type of future to use for nested futures"),
#> "\n", list("\n", "getExpression(future, ...)\n"),
#> "\n", list("\n", list(list("future"), list("Current future.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A future expression with code injected to set what\n",
#> "type of future to use for nested futures, iff any.\n"),
#> "\n", list("\n", "Inject code for the next type of future to use for nested futures\n"),
#> "\n", list("\n", "If there is no future backend specified after this one, the default\n",
#> "is to use ", list("sequential"), " futures. This conservative approach protects\n",
#> "against spawning off recursive futures by mistake, especially\n",
#> list("multicore"), " and ", list("multisession"),
#> " ones.\n", "The default will also set ", list(
#> "options(mc.cores = 1L)"), " (*) so that\n",
#> "no parallel ", list(), " processes are spawned off by functions such as\n",
#> list("parallel::mclapply()"), " and friends.\n",
#> "\n", "Currently it is not possible to specify what type of nested\n",
#> "futures to be used, meaning the above default will always be\n",
#> "used.\n", "See ", list(list("https://github.com/futureverse/future/issues/37"),
#> list("Issue #37")), "\n", "for plans on adding support for custom nested future types.\n",
#> "\n", "(*) Ideally we would set ", list("mc.cores = 0"),
#> " but that will unfortunately\n", "cause ",
#> list("mclapply()"), " and friends to generate an error saying\n",
#> "\"'mc.cores' must be >= 1\". Ideally those functions should\n",
#> "fall back to using the non-multicore alternative in this\n",
#> "case, e.g. ", list("mclapply(...)"), " => ",
#> list("lapply(...)"), ".\n", "See ", list("https://github.com/HenrikBengtsson/Wishlist-for-R/issues/7"),
#> "\n", "for a discussion on this.\n"), "\n",
#> list("internal"), "\n"), getGlobalsAndPackages.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-globals.R",
#> "\n", list("getGlobalsAndPackages"), "\n", list(
#> "getGlobalsAndPackages"), "\n", list("Retrieves global variables of an expression and their associated packages"),
#> "\n", list("\n", "getGlobalsAndPackages(\n",
#> " expr,\n", " envir = parent.frame(),\n",
#> " tweak = tweakExpression,\n", " globals = TRUE,\n",
#> " locals = getOption(\"future.globals.globalsOf.locals\", TRUE),\n",
#> " resolve = getOption(\"future.globals.resolve\"),\n",
#> " persistent = FALSE,\n", " maxSize = getOption(\"future.globals.maxSize\", 500 * 1024^2),\n",
#> " onReference = getOption(\"future.globals.onReference\", \"ignore\"),\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "expr"), list("An ", list(), " expression whose globals should be found.")),
#> "\n", "\n", list(list("envir"), list("The environment from which globals should be searched.")),
#> "\n", "\n", list(list("tweak"), list("(optional) A function that takes an expression and returned a modified one.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, a named list, or a ",
#> list("Globals"), " object. If TRUE, globals are identified by code inspection based on ",
#> list("expr"), " and ", list("tweak"), " searching from environment ",
#> list("envir"), ". If FALSE, no globals are used. If a character vector, then globals are identified by lookup based their names ",
#> list("globals"), " searching from environment ",
#> list("envir"), ". If a named list or a Globals object, the globals are used as is.")),
#> "\n", "\n", list(list("locals"), list("Should globals part of any \"local\" environment of\n",
#> "a function be included or not?")), "\n",
#> "\n", list(list("resolve"), list("If TRUE, any future that is a global variables (or part of one) is resolved and replaced by a \"constant\" future.")),
#> "\n", "\n", list(list("persistent"), list("If TRUE, non-existing globals (= identified in expression but not found in memory) are always silently ignored and assumed to be existing in the evaluation environment. If FALSE, non-existing globals are by default ignored, but may also trigger an informative error if option ",
#> list("future.globals.onMissing"), " in ",
#> list("\"error\""), " (should only be used for troubleshooting).")),
#> "\n", "\n", list(list("maxSize"), list("The maximum allowed total size (in bytes) of globals---for\n",
#> "the purpose of preventing too large exports / transfers happening by\n",
#> "mistake. If the total size of the global objects are greater than this\n",
#> "limit, an informative error message is produced. If\n",
#> list("maxSize = +Inf"), ", then this assertion is skipped. (Default: 500 MiB).")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A named list with elements ",
#> list("expr"), " (the tweaked expression), ",
#> list("globals"), " (a named list of class ",
#> list("FutureGlobals"), ") and ", list("packages"),
#> " (a character string).\n"), "\n", list("\n",
#> "Retrieves global variables of an expression and their associated packages\n"),
#> "\n", list("\n", "Internally, ", list(list("globalsOf"),
#> "()"), " is used to identify globals and associated packages from the expression.\n"),
#> "\n", list("internal"), "\n"), makeClusterFuture.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-makeClusterFuture.R",
#> "\n", list("makeClusterFuture"), "\n", list("makeClusterFuture"),
#> "\n", list("FUTURE"), "\n", list("Create a Future Cluster of Stateless Workers for Parallel Processing"),
#> "\n", list("\n", "makeClusterFuture(specs = nbrOfWorkers(), ...)\n"),
#> "\n", list("\n", list(list("specs"), list("Ignored.\n",
#> "If specified, the value should equal ", list(
#> "nbrOfWorkers()"), " (default).\n", "A missing value corresponds to specifying ",
#> list("nbrOfWorkers()"), ".\n", "This argument exists only to support\n",
#> list("parallel::makeCluster(NA, type = future::FUTURE)"),
#> ".")), "\n", "\n", list(list(list()), list(
#> "Named arguments passed to ", list(list("future()")),
#> ".")), "\n"), "\n", list("\n", "Returns a ",
#> list("parallel"), " ", list("cluster"), " object of class ",
#> list("FutureCluster"), ".\n"), "\n", list("\n",
#> list("WARNING: Please note that this sets up a stateless set of cluster nodes,\n",
#> "which means that ", list("clusterEvalQ(cl, { a <- 3.14 })"),
#> " will have no effect.\n", "Consider this a first beta version and use it with great care,\n",
#> "particularly because of the stateless nature of the cluster.\n",
#> "For now, I recommend to manually validate that you can get identical\n",
#> "results using this cluster type with what you get from using the\n",
#> "classical ", list("parallel::makeCluster()"),
#> " cluster type."), "\n"), "\n", list(list(
#> "Future Clusters are Stateless"), list("\n",
#> "\n", "Traditionally, a cluster nodes has a one-to-one mapping to a cluster\n",
#> "worker process. For example, ", list("cl <- makeCluster(2, type = \"PSOCK\")"),
#> "\n", "launches two parallel worker processes in the background, where\n",
#> "cluster node ", list("cl[[1]]"), " maps to worker #1 and node ",
#> list("cl[[2]]"), " to\n", "worker #2, and that never changes through the lifespan of these\n",
#> "workers. This one-to-one mapping allows for deterministic\n",
#> "configuration of workers. For examples, some code may assign globals\n",
#> "with values specific to each worker, e.g.\n",
#> list("clusterEvalQ(cl[1], { a <- 3.14 })"),
#> " and\n", list("clusterEvalQ(cl[2], { a <- 2.71 })"),
#> ".\n", "\n", "In contrast, there is no one-to-one mapping between cluster nodes\n",
#> "and the parallel workers when using a future cluster. This is because\n",
#> "we cannot make assumptions on where are parallel task will be\n",
#> "processed. Where a parallel task is processes is up to the future\n",
#> "backend to decide - some backends do this deterministically, whereas\n",
#> "others other resolves task at the first available worker. Also, the\n",
#> "worker processes might be ", list("transient"),
#> " for some future backends, i.e.\n", "the only exist for the life-span of the parallel task and then\n",
#> "terminates.\n", "\n", "Because of this, one must not rely in node-specific behaviors,\n",
#> "because that concept does not make sense with a future cluster.\n",
#> "To protect against this, any attempt to address a subset of future\n",
#> "cluster nodes, results in an error, e.g. ",
#> list("clusterEvalQ(cl[1], ...)"), ",\n", list(
#> "clusterEvalQ(cl[1:2], ...)"), ", and ",
#> list("clusterEvalQ(cl[2:1], ...)"), " in\n",
#> "the above example will all give an error.\n",
#> "\n", "Exceptions to the latter limitation are ",
#> list("clusterSetRNGStream()"), "\n", "and ",
#> list("clusterExport()"), ", which can be safely used with future clusters.\n",
#> "See below for more details.\n", "If ", list(
#> "clusterEvalQ()"), " is called, the call is ignored, and a warning\n",
#> "is produced.\n")), "\n", "\n", list(list("clusterSetRNGStream"),
#> list("\n", "\n", list(list("parallel::clusterSetRNGStream()")),
#> " distributes \"L'Ecuyer-CMRG\" RNG\n", "streams to the cluster nodes, which record them such that the next\n",
#> "round of futures will use them. When used, the RNG state after the\n",
#> "futures are resolved are recorded accordingly, such that the next\n",
#> "round again of future will use those, and so on. This strategy\n",
#> "makes sure ", list("clusterSetRNGStream()"),
#> " has the expected effect although\n", "futures are stateless.\n")),
#> "\n", "\n", list(list("clusterExport"), list(
#> "\n", "\n", list(list("parallel::clusterExport()")),
#> " assign values to the cluster nodes.\n", "Specifically, these values are recorded and are used as globals\n",
#> "for all futures created there on.\n")), "\n",
#> "\n", list("\n", list("if ((getRversion() >= \"4.4.0\")) (if (getRversion() >= \"3.4\") withAutoprint else force)({ # examplesIf"),
#> "\n", "plan(multisession)\n", "cl <- makeClusterFuture()\n",
#> "\n", "parallel::clusterSetRNGStream(cl)\n",
#> "\n", "y <- parallel::parLapply(cl, 11:13, function(x) {\n",
#> " message(\"Process ID: \", Sys.getpid())\n",
#> " mean(rnorm(n = x))\n", "})\n", "str(y)\n",
#> "\n", "plan(sequential)\n", list("}) # examplesIf"),
#> "\n"), "\n", list("internal"), "\n"), mandelbrot.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/demo_api-mandelbrot.R",
#> "\n", list("mandelbrot"), "\n", list("mandelbrot"),
#> "\n", list("as.raster.Mandelbrot"), "\n", list(
#> "plot.Mandelbrot"), "\n", list("mandelbrot_tiles"),
#> "\n", list("mandelbrot.matrix"), "\n", list("mandelbrot.numeric"),
#> "\n", list("Mandelbrot convergence counts"),
#> "\n", list("\n", "mandelbrot(...)\n", "\n", list(
#> list("mandelbrot"), list("matrix")), "(Z, maxIter = 200L, tau = 2, ...)\n",
#> "\n", list(list("mandelbrot"), list("numeric")),
#> "(\n", " xmid = -0.75,\n", " ymid = 0,\n",
#> " side = 3,\n", " resolution = 400L,\n",
#> " maxIter = 200L,\n", " tau = 2,\n", " ...\n",
#> ")\n"), "\n", list("\n", list(list("Z"), list(
#> "A complex matrix for which convergence\n",
#> "counts should be calculated.")), "\n", "\n",
#> list(list("maxIter"), list("Maximum number of iterations per bin.")),
#> "\n", "\n", list(list("tau"), list("A threshold; the radius when calling\n",
#> "divergence (Mod(z) > tau).")), "\n", "\n",
#> list(list("xmid, ymid, side, resolution"),
#> list("Alternative specification of\n", "the complex plane ",
#> list("Z"), ", where\n", list("mean(Re(Z)) == xmid"),
#> ",\n", list("mean(Im(Z)) == ymid"), ",\n",
#> list("diff(range(Re(Z))) == side"), ",\n",
#> list("diff(range(Im(Z))) == side"), ", and\n",
#> list("dim(Z) == c(resolution, resolution)"),
#> ".")), "\n"), "\n", list("\n", "Returns an integer matrix (of class Mandelbrot) with\n",
#> "non-negative counts.\n"), "\n", list("\n",
#> "Mandelbrot convergence counts\n"), "\n", list(
#> "\n", "counts <- mandelbrot(xmid = -0.75, ymid = 0, side = 3)\n",
#> "str(counts)\n", list("\n", "plot(counts)\n"),
#> "\n", "\n", list("\n", "demo(\"mandelbrot\", package = \"future\", ask = FALSE)\n"),
#> "\n", "\n"), "\n", list("\n", "The internal Mandelbrot algorithm was inspired by and\n",
#> "adopted from similar GPL code of Martin Maechler available\n",
#> "from ftp://stat.ethz.ch/U/maechler/R/ on 2005-02-18 (sic!).\n"),
#> "\n", list("internal"), "\n"), multicore.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("multicore"), "\n", list("multicore"),
#> "\n", list("Create a multicore future whose value will be resolved asynchronously in a forked parallel process"),
#> "\n", list("\n", "multicore(\n", " ...,\n",
#> " workers = availableCores(constraints = \"multicore\"),\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " envir = parent.frame()\n", ")\n"), "\n",
#> list("\n", list(list("workers"), list("The number of parallel processes to use.\n",
#> "If a function, it is called without arguments ",
#> list("when the future\n", "is created"), " and its value is used to configure the workers.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(
#> list()), list("Additional named elements to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A ", list("Future"), ".\n", "If ",
#> list("workers == 1"), ", then all processing using done in the\n",
#> "current/main ", list(), " session and we therefore fall back to using a\n",
#> "sequential future. To override this fallback, use ",
#> list("workers = I(1)"), ".\n", "This is also the case whenever multicore processing is not supported,\n",
#> "e.g. on Windows.\n"), "\n", list("\n", list(
#> "WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A multicore future is a future that uses multicore evaluation,\n",
#> "which means that its ", list("value is computed and resolved in\n",
#> "parallel in another process"), ".\n", "\n",
#> "This function is must ", list("not"), " be called directly. Instead, the\n",
#> "typical usages are:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures in parallel on the local machine via as many forked\n",
#> "# processes as available to the current R process\n",
#> "plan(multicore)\n", "\n", "# Evaluate futures in parallel on the local machine via two forked processes\n",
#> "plan(multicore, workers = 2)\n"), list(list(
#> "html"), list(list("</div>"))), "\n"), "\n",
#> list(list("Support for forked (\"multicore\") processing"),
#> list("\n", "\n", "Not all operating systems support process forking and thereby not multicore\n",
#> "futures. For instance, forking is not supported on Microsoft Windows.\n",
#> "Moreover, process forking may break some R environments such as RStudio.\n",
#> "Because of this, the future package disables process forking also in\n",
#> "such cases. See ", list(list("parallelly::supportsMulticore()")),
#> " for details.\n", "Trying to create multicore futures on non-supported systems or when\n",
#> "forking is disabled will result in multicore futures falling back to\n",
#> "becoming ", list("sequential"), " futures. If used in RStudio, there will be an\n",
#> "informative warning:\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode r\">"))),
#> list("> plan(multicore)\n", "Warning message:\n",
#> "In supportsMulticoreAndRStudio(...) :\n",
#> " [ONE-TIME WARNING] Forked processing ('multicore') is not supported when\n",
#> "running R from RStudio because it is considered unstable. For more details,\n",
#> "how to control forked processing or not, and how to silence this warning in\n",
#> "future R sessions, see ?parallelly::supportsMulticore\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n")), "\n", "\n", list("\n", "## Use multicore futures\n",
#> "plan(multicore)\n", "\n", "## A global variable\n",
#> "a <- 0\n", "\n", "## Create future (explicitly)\n",
#> "f <- future({\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "\n", "## A multicore future is evaluated in a separate forked\n",
#> "## process. Changing the value of a global variable\n",
#> "## will not affect the result of the future.\n",
#> "a <- 7\n", "print(a)\n", "\n", "v <- value(f)\n",
#> "print(v)\n", "stopifnot(v == 0)\n"), "\n",
#> list("\n", "For processing in multiple background ",
#> list(), " sessions, see\n", list("multisession"),
#> " futures.\n", "\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n", "\n", "Use ", list(list("parallelly::availableCores()")),
#> " to see the total number of\n", "cores that are available for the current ",
#> list(), " session.\n", "Use ", list(list("availableCores"),
#> "(\"multicore\") > 1L"), " to check\n", "whether multicore futures are supported or not on the current\n",
#> "system.\n"), "\n"), multisession.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in", "\n",
#> "% R/backend_api-13.MultisessionFutureBackend-class.R",
#> "\n", list("multisession"), "\n", list("multisession"),
#> "\n", list("Create a multisession future whose value will be resolved asynchronously in a parallel ",
#> list(), " session"), "\n", list("\n", "multisession(\n",
#> " ...,\n", " workers = availableCores(),\n",
#> " lazy = FALSE,\n", " rscript_libs = .libPaths(),\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " envir = parent.frame()\n", ")\n"), "\n",
#> list("\n", list(list("workers"), list("The number of parallel processes to use.\n",
#> "If a function, it is called without arguments ",
#> list("when the future\n", "is created"), " and its value is used to configure the workers.")),
#> "\n", "\n", list(list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("rscript_libs"), list(
#> "A character vector of ", list(), " package library folders that\n",
#> "the workers should use. The default is ",
#> list(".libPaths()"), " so that multisession\n",
#> "workers inherits the same library path as the main ",
#> list(), " session.\n", "To avoid this, use ",
#> list("plan(multisession, ..., rscript_libs = NULL)"),
#> ".\n", list("Important: Note that the library path is set on the workers when they are\n",
#> "created, i.e. when ", list("plan(multisession)"),
#> " is called. Any changes to\n", list(".libPaths()"),
#> " in the main R session after the workers have been created\n",
#> "will have no effect."), "\n", "This is passed down as-is to ",
#> list(list("parallelly::makeClusterPSOCK()")),
#> ".")), "\n", "\n", list(list("gc"), list(
#> "If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(
#> list()), list("Additional arguments passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A MultisessionFuture.\n", "If ",
#> list("workers == 1"), ", then all processing is done in the\n",
#> "current/main ", list(), " session and we therefore fall back to using a\n",
#> "lazy future. To override this fallback, use ",
#> list("workers = I(1)"), ".\n"), "\n", list(
#> "\n", list("WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A multisession future is a future that uses multisession evaluation,\n",
#> "which means that its ", list("value is computed and resolved in\n",
#> "parallel in another ", list(), " session"),
#> ".\n", "\n", "This function is must ", list(
#> "not"), " be called directly. Instead, the\n",
#> "typical usages are:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures in parallel on the local machine via as many background\n",
#> "# processes as available to the current R process\n",
#> "plan(multisession)\n", "\n", "# Evaluate futures in parallel on the local machine via two background\n",
#> "# processes\n", "plan(multisession, workers = 2)\n"),
#> list(list("html"), list(list("</div>"))), "\n",
#> "\n", "The background ", list(), " sessions (the \"workers\") are created using\n",
#> list(list("makeClusterPSOCK()")), ".\n", "\n",
#> "For the total number of\n", list(), " sessions available including the current/main ",
#> list(), " process, see\n", list(list("parallelly::availableCores()")),
#> ".\n", "\n", "A multisession future is a special type of cluster future.\n"),
#> "\n", list("\n", list("\n", "\n", "## Use multisession futures\n",
#> "plan(multisession)\n", "\n", "## A global variable\n",
#> "a <- 0\n", "\n", "## Create future (explicitly)\n",
#> "f <- future({\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "\n", "## A multisession future is evaluated in a separate R session.\n",
#> "## Changing the value of a global variable will not affect\n",
#> "## the result of the future.\n", "a <- 7\n",
#> "print(a)\n", "\n", "v <- value(f)\n", "print(v)\n",
#> "stopifnot(v == 0)\n", "\n", "## Explicitly close multisession workers by switching plan\n",
#> "plan(sequential)\n"), "\n"), "\n", list("\n",
#> "For processing in multiple forked ", list(),
#> " sessions, see\n", list("multicore"), " futures.\n",
#> "\n", "Use ", list(list("parallelly::availableCores()")),
#> " to see the total number of\n", "cores that are available for the current ",
#> list(), " session.\n"), "\n"), nbrOfWorkers.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-nbrOfWorkers.R",
#> "\n", list("nbrOfWorkers"), "\n", list("nbrOfWorkers"),
#> "\n", list("nbrOfFreeWorkers"), "\n", list("Get the number of workers available"),
#> "\n", list("\n", "nbrOfWorkers(evaluator = NULL)\n",
#> "\n", "nbrOfFreeWorkers(evaluator = NULL, background = FALSE, ...)\n"),
#> "\n", list("\n", list(list("evaluator"), list(
#> "A future evaluator function.\n", "If NULL (default), the current evaluator as returned\n",
#> "by ", list(list("plan()")), " is used.")),
#> "\n", "\n", list(list("background"), list("If TRUE, only workers that can process a future in the\n",
#> "background are considered. If FALSE, also workers running in the main ",
#> list(), "\n", "process are considered, e.g. when using the 'sequential' backend.")),
#> "\n", "\n", list(list(list()), list("Not used; reserved for future use.")),
#> "\n"), "\n", list("\n", list("nbrOfWorkers()"),
#> " returns a positive number in ", list(list(
#> "{1, 2, 3, ...}")), ", which\n", "for some future backends may also be ",
#> list("+Inf"), ".\n", "\n", list("nbrOfFreeWorkers()"),
#> " returns a non-negative number in\n", list(
#> list("{0, 1, 2, 3, ...}")), " which is less than or equal to ",
#> list("nbrOfWorkers()"), ".\n"), "\n", list(
#> "\n", "Get the number of workers available\n"),
#> "\n", list("\n", "plan(multisession)\n", "nbrOfWorkers() ## == availableCores()\n",
#> "\n", "plan(sequential)\n", "nbrOfWorkers() ## == 1\n"),
#> "\n"), nullcon.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-basic.R",
#> "\n", list("nullcon"), "\n", list("nullcon"),
#> "\n", list("Creates a connection to the system null device"),
#> "\n", list("\n", "nullcon()\n"), "\n", list("\n",
#> "Returns a open, binary ", list(list("base::connection()")),
#> ".\n"), "\n", list("\n", "Creates a connection to the system null device\n"),
#> "\n", list("internal"), "\n"), plan.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-plan-with.R, R/utils_api-plan.R,",
#> "\n", "% R/utils_api-tweak.R", "\n", list("with.FutureStrategyList"),
#> "\n", list("with.FutureStrategyList"), "\n",
#> list("plan"), "\n", list("tweak"), "\n", list(
#> "Evaluate an expression using a temporarily set future plan"),
#> "\n", list("\n", list(list("with"), list("FutureStrategyList")),
#> "(data, expr, ..., local = FALSE, envir = parent.frame(), .cleanup = NA)\n",
#> "\n", "plan(\n", " strategy = NULL,\n", " ...,\n",
#> " substitute = TRUE,\n", " .skip = FALSE,\n",
#> " .call = TRUE,\n", " .cleanup = NA,\n",
#> " .init = TRUE\n", ")\n", "\n", "tweak(strategy, ..., penvir = parent.frame())\n"),
#> "\n", list("\n", list(list("data"), list("The future plan to use temporarily.")),
#> "\n", "\n", list(list("expr"), list("The R expression to be evaluated.")),
#> "\n", "\n", list(list("local"), list("If TRUE, then the future plan specified by ",
#> list("data"), "\n", "is applied temporarily in the calling frame. Argument ",
#> list("expr"), " must\n", "not be specified if ",
#> list("local = TRUE"), ".")), "\n", "\n",
#> list(list("envir"), list("The environment where the future plan should be set and the\n",
#> "expression evaluated.")), "\n", "\n", list(
#> list(".cleanup"), list("(internal) Used to stop implicitly started clusters.")),
#> "\n", "\n", list(list("strategy"), list("An existing future function or the name of one.")),
#> "\n", "\n", list(list("substitute"), list("If ",
#> list("TRUE"), ", the ", list("strategy"),
#> " expression is\n", list("substitute()"),
#> ":d, otherwise not.")), "\n", "\n", list(
#> list(".skip"), list("(internal) If ", list(
#> "TRUE"), ", then attempts to set a future backend\n",
#> "that is the same as what is currently in use, will be skipped.")),
#> "\n", "\n", list(list(".call"), list("(internal) Used for recording the call to this function.")),
#> "\n", "\n", list(list(".init"), list("(internal) Used to initiate workers.")),
#> "\n", "\n", list(list("penvir"), list("The environment used when searching for a future\n",
#> "function by its name.")), "\n", "\n", list(
#> list(list()), list("Named arguments to replace the defaults of existing\n",
#> "arguments.")), "\n"), "\n", list("\n",
#> "The value of the expression evaluated (invisibly).\n",
#> "\n", list("plan()"), " returns a the previous plan invisibly if a new future backend\n",
#> "is chosen, otherwise it returns the current one visibly.\n",
#> "\n", "a future function.\n"), "\n", list("\n",
#> "This function allows ", list("the user"),
#> " to plan the future, more specifically,\n",
#> "it specifies how ", list(list("future()")),
#> ":s are resolved,\n", "e.g. sequentially or in parallel.\n"),
#> "\n", list("\n", "The default backend is ", list(
#> list("sequential")), ", but another one can be set\n",
#> "using ", list("plan()"), ", e.g. ", list("plan(multisession)"),
#> " will launch parallel workers\n", "running in the background, which then will be used to resolve future.\n",
#> "To shut down background workers launched this way, call ",
#> list("plan(sequential)"), ".\n"), "\n", list(
#> list("Built-in evaluation strategies"), list(
#> "\n", "\n", "The ", list("future"), " package provides the following built-in backends:\n",
#> "\n", list("\n", list(list(list(list("sequential")),
#> ":"), list("\n", "Resolves futures sequentially in the current ",
#> list(), " process, e.g.\n", list("plan(sequential)"),
#> ".\n")), "\n", list(list(list(list("multisession")),
#> ":"), list("\n", "Resolves futures asynchronously (in parallel) in separate\n",
#> list(), " sessions running in the background on the same machine, e.g.\n",
#> list("plan(multisession)"), " and ", list(
#> "plan(multisession, workers = 2)"), ".\n")),
#> "\n", list(list(list(list("multicore")),
#> ":"), list("\n", "Resolves futures asynchronously (in parallel) in separate\n",
#> list("forked"), " ", list(), " processes running in the background on\n",
#> "the same machine, e.g.\n", list("plan(multicore)"),
#> " and ", list("plan(multicore, workers = 2)"),
#> ".\n", "This backend is not supported on Windows.\n")),
#> "\n", list(list(list(list("cluster")),
#> ":"), list("\n", "Resolves futures asynchronously (in parallel) in separate\n",
#> list(), " sessions running typically on one or more machines, e.g.\n",
#> list("plan(cluster)"), ", ", list("plan(cluster, workers = 2)"),
#> ", and\n", list("plan(cluster, workers = c(\"n1\", \"n1\", \"n2\", \"server.remote.org\"))"),
#> ".\n")), "\n"), "\n")), "\n", "\n", list(
#> list("Other evaluation strategies available"),
#> list("\n", "\n", "\n", "In addition to the built-in ones, additional parallel backends are\n",
#> "implemented in future-backend packages ",
#> list("future.callr"), " and\n", list("future.mirai"),
#> " that leverage R package ", list("callr"),
#> " and\n", list("mirai"), ":\n", "\n", list(
#> "\n", list(list(list("callr"), ":"), list(
#> "\n", "Similar to ", list("multisession"),
#> ", this resolved futures in parallel in\n",
#> "background ", list(), " sessions on the local machine via the ",
#> list("callr"), "\n", "package, e.g. ",
#> list("plan(future.callr::callr)"), " and\n",
#> list("plan(future.callr::callr, workers = 2)"),
#> ". The difference is that\n", "each future is processed in a fresh parallel R worker, which is\n",
#> "automatically shut down as soon as the future is resolved.\n",
#> "This can help decrease the overall memory. Moreover, contrary\n",
#> "to ", list("multisession"), ", ", list(
#> "callr"), " does not rely on socket connections,\n",
#> "which means it is not limited by the number of connections that\n",
#> list(), " can have open at any time.\n")),
#> "\n", "\n", list(list(list("mirai_multisession"),
#> ":"), list("\n", "Similar to ", list(
#> "multisession"), ", this resolved futures in parallel in\n",
#> "background ", list(), " sessions on the local machine via the ",
#> list("mirai"), "\n", "package, e.g. ",
#> list("plan(future.mirai::mirai_multisession)"),
#> " and\n", list("plan(future.mirai::mirai_multisession, workers = 2)"),
#> ".\n")), "\n", "\n", list(list(list("mirai_cluster"),
#> ":"), list("\n", "Similar to ", list(
#> "cluster"), ", this resolved futures in parallel via\n",
#> "pre-configured ", list(), " ", list(
#> "mirai"), " daemon processes, e.g.\n",
#> list("plan(future.mirai::mirai_cluster)"),
#> ".\n")), "\n"), "\n", "\n", "Another example is the ",
#> list("future.batchtools"), " package, which leverages\n",
#> list("batchtools"), " package, to resolve futures via high-performance compute\n",
#> "(HPC) job schedulers, e.g. LSF, Slurm, TORQUE/PBS, Grid Engine, and\n",
#> "OpenLava;\n", "\n", list("\n", list(list(
#> list("batchtools_slurm"), ":"), list("\n",
#> "The backend resolved futures via the Slurm scheduler, e.g.\n",
#> list("plan(future.batchtools::batchtools_slurm)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_torque"),
#> ":"), list("\n", "The backend resolved futures via the TORQUE/PBS scheduler, e.g.\n",
#> list("plan(future.batchtools::batchtools_torque)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_sge"),
#> ":"), list("\n", "The backend resolved futures via the Grid Engine (SGE, AGE) scheduler,\n",
#> "e.g. ", list("plan(future.batchtools::batchtools_sge)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_lsf"),
#> ":"), list("\n", "The backend resolved futures via the Load Sharing Facility (LSF)\n",
#> "scheduler, e.g. ", list("plan(future.batchtools::batchtools_lsf)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_openlava"),
#> ":"), list("\n", "The backend resolved futures via the OpenLava scheduler, e.g.\n",
#> list("plan(future.batchtools::batchtools_openlava)"),
#> ".\n")), "\n"), "\n")), "\n", "\n", list(
#> list("For package developers"), list("\n",
#> "\n", "\n", "Please refrain from modifying the future backend inside your packages /\n",
#> "functions, i.e. do not call ", list("plan()"),
#> " in your code. Instead, leave\n", "the control on what backend to use to the end user. This idea is part of\n",
#> "the core philosophy of the future framework---as a developer you can never\n",
#> "know what future backends the user have access to. Moreover, by not making\n",
#> "any assumptions about what backends are available, your code will also work\n",
#> "automatically with any new backends developed after you wrote your code.\n",
#> "\n", "If you think it is necessary to modify the future backend within a\n",
#> "function, then make sure to undo the changes when exiting the function.\n",
#> "This can be achieved by using ", list("with(plan(...), local = TRUE)"),
#> ", e.g.\n", "\n", list("\n", " my_fcn <- function(x) {\n",
#> " with(plan(multisession), local = TRUE)\n",
#> " y <- analyze(x)\n", " summarize(y)\n",
#> " }\n"), "\n", "\n", "This is important because the end-user might have already set the future\n",
#> "strategy elsewhere for other purposes and will most likely not known that\n",
#> "calling your function will break their setup.\n",
#> list("Remember, your package and its functions might be used in a greater\n",
#> "context where multiple packages and functions are involved and those might\n",
#> "also rely on the future framework, so it is important to avoid stepping on\n",
#> "others' toes."), "\n")), "\n", "\n", list(
#> list("Using plan() in scripts and vignettes"),
#> list("\n", "\n", "\n", "When writing scripts or vignettes that use futures, try to place any\n",
#> "call to ", list("plan()"), " as far up (i.e. as early on) in the code as possible.\n",
#> "This will help users to quickly identify where the future plan is set up\n",
#> "and allow them to modify it to their computational resources.\n",
#> "Even better is to leave it to the user to set the ",
#> list("plan()"), " prior to\n", list("source()"),
#> ":ing the script or running the vignette.\n",
#> "If a ", list(list(".future.R")), " exists in the current directory and / or in\n",
#> "the user's home directory, it is sourced when the ",
#> list("future"), " package is\n", list("loaded"),
#> ". Because of this, the ", list(".future.R"),
#> " file provides a\n", "convenient place for users to set the ",
#> list("plan()"), ".\n", "This behavior can be controlled via an ",
#> list(), " option---see\n", list("future options"),
#> " for more details.\n")), "\n", "\n", list(
#> "\n", "# Evaluate a future using the 'multisession' plan\n",
#> "with(plan(multisession, workers = 2), {\n",
#> " f <- future(Sys.getpid())\n", " w_pid <- value(f)\n",
#> "})\n", "print(c(main = Sys.getpid(), worker = w_pid))\n",
#> "\n", "\n", "\n", "# Evaluate a future locally using the 'multisession' plan\n",
#> "local({\n", " with(plan(multisession, workers = 2), local = TRUE)\n",
#> "\n", " f <- future(Sys.getpid())\n", " w_pid <- value(f)\n",
#> " print(c(main = Sys.getpid(), worker = w_pid))\n",
#> "})\n", "\n", "\n", "a <- b <- c <- NA_real_\n",
#> "\n", "# An sequential future\n", "plan(sequential)\n",
#> "f <- future({\n", " a <- 7\n", " b <- 3\n",
#> " c <- 2\n", " a * b * c\n", "})\n", "y <- value(f)\n",
#> "print(y)\n", "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n", "\n", "# A sequential future with lazy evaluation\n",
#> "plan(sequential)\n", "f <- future({\n", " a <- 7\n",
#> " b <- 3\n", " c <- 2\n", " a * b * c\n",
#> "}, lazy = TRUE)\n", "y <- value(f)\n", "print(y)\n",
#> "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n", "\n", "# A multicore future (specified as a string)\n",
#> "plan(\"multicore\")\n", "f <- future({\n",
#> " a <- 7\n", " b <- 3\n", " c <- 2\n", " a * b * c\n",
#> "})\n", "y <- value(f)\n", "print(y)\n", "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n", "## Multisession futures gives an error on R CMD check on\n",
#> "## Windows (but not Linux or macOS) for unknown reasons.\n",
#> "## The same code works in package tests.\n",
#> list("\n", "\n", "# A multisession future (specified via a string variable)\n",
#> "plan(\"future::multisession\")\n", "f <- future({\n",
#> " a <- 7\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "y <- value(f)\n",
#> "print(y)\n", "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n"), "\n", "\n", "\n", "## Explicitly specifying number of workers\n",
#> "## (default is parallelly::availableCores())\n",
#> "plan(multicore, workers = 2)\n", "message(\"Number of parallel workers: \", nbrOfWorkers())\n",
#> "\n", "\n", "## Explicitly close multisession workers by switching plan\n",
#> "plan(sequential)\n"), "\n", list("\n", "Use ",
#> list(list("plan()")), " to set a future to become the\n",
#> "new default strategy.\n"), "\n"), private_length.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-basic.R",
#> "\n", list(".length"), "\n", list(".length"),
#> "\n", list("Gets the length of an object without dispatching"),
#> "\n", list("\n", ".length(x)\n"), "\n", list(
#> "\n", list(list("x"), list("Any ", list(),
#> " object.")), "\n"), "\n", list("\n", "A non-negative integer.\n"),
#> "\n", list("\n", "Gets the length of an object without dispatching\n"),
#> "\n", list("\n", "This function returns ", list(
#> "length(unclass(x))"), ", but tries to avoid\n",
#> "calling ", list("unclass(x)"), " unless necessary.\n"),
#> "\n", list("\n", list(list(".subset"), "()"),
#> " and ", list(list(".subset2"), "()"), ".\n"),
#> "\n", list("internal"), "\n"), `re-exports.Rd` = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/000.re-exports.R",
#> "\n", list("re-exports"), "\n", list("re-exports"),
#> "\n", list("as.cluster"), "\n", list("availableCores"),
#> "\n", list("availableWorkers"), "\n", list("makeClusterPSOCK"),
#> "\n", list("supportsMulticore"), "\n", list("Functions Moved to 'parallelly'"),
#> "\n", list("\n", "The following function used to be part of ",
#> list("future"), " but has since\n", "been migrated to ",
#> list("parallelly"), ". The migration started with\n",
#> list("future"), " 1.20.0 (November 2020). They were moved because they\n",
#> "are also useful outside of the ", list("future"),
#> " framework.\n"), "\n", list("\n", list("If you are using any of these from the ",
#> list("future"), " package, please\n", "switch to use the ones from the ",
#> list("parallelly"), " package. Thank you!"),
#> "\n", list("\n", list(), " ", list(list("parallelly::as.cluster()")),
#> "\n", list(), " ", list(list("parallelly::autoStopCluster()")),
#> " (no longer re-exported)\n", list(), " ",
#> list(list("parallelly::availableCores()")),
#> "\n", list(), " ", list(list("parallelly::availableWorkers()")),
#> "\n", list(), " ", list(list("parallelly::makeClusterMPI()")),
#> " (no longer re-exported)\n", list(),
#> " ", list(list("parallelly::makeClusterPSOCK()")),
#> "\n", list(), " ", list(list("parallelly::makeNodePSOCK()")),
#> " (no longer re-exported)\n", list(),
#> " ", list(list("parallelly::supportsMulticore()")),
#> "\n"), "\n", "\n", "For backward-compatible reasons, ",
#> list("some"), " of these functions remain\n",
#> "available as exact copies also from this package (as re-exports), e.g.\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("cl <- parallelly::makeClusterPSOCK(2)\n"),
#> list(list("html"), list(list("</div>"))), "\n",
#> "\n", "can still be accessed as:\n", "\n",
#> list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("cl <- future::makeClusterPSOCK(2)\n"),
#> list(list("html"), list(list("</div>"))), "\n",
#> "\n", list("Note that it is the goal to remove all of the above from this package."),
#> "\n"), "\n", list("internal"), "\n"), readImmediateConditions.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-immediateCondition.R",
#> "\n", list("readImmediateConditions"), "\n",
#> list("readImmediateConditions"), "\n", list("saveImmediateCondition"),
#> "\n", list("Writes and Reads 'immediateCondition' RDS Files"),
#> "\n", list("\n", "readImmediateConditions(\n",
#> " path = immediateConditionsPath(rootPath = rootPath),\n",
#> " rootPath = tempdir(),\n", " pattern = \"[.]rds$\",\n",
#> " include = getOption(\"future.relay.immediate\", \"immediateCondition\"),\n",
#> " signal = FALSE,\n", " remove = TRUE\n",
#> ")\n", "\n", "saveImmediateCondition(\n", " cond,\n",
#> " path = immediateConditionsPath(rootPath = rootPath),\n",
#> " rootPath = tempdir()\n", ")\n"), "\n", list(
#> "\n", list(list("path"), list("(character string) The folder where the RDS files are.")),
#> "\n", "\n", list(list("pattern"), list("(character string) A regular expression selecting\n",
#> "the RDS files to be read.")), "\n", "\n",
#> list(list("include"), list("(character vector) The class or classes of the objects\n",
#> "to be kept.")), "\n", "\n", list(list("signal"),
#> list("(logical) If TRUE, the condition read are signaled.")),
#> "\n", "\n", list(list("remove"), list("(logical) If TRUE, the RDS files used are removed on exit.")),
#> "\n", "\n", list(list("cond"), list("A condition of class ",
#> list("immediateCondition"), ".")), "\n"),
#> "\n", list("\n", list("readImmediateConditions()"),
#> " returns an unnamed ", list("base::list"),
#> " of\n", "named lists with elements ", list(
#> "condition"), " and ", list("signaled"),
#> ", where\n", "the ", list("condition"), " elements hold ",
#> list("immediateCondition"), " objects.\n",
#> "\n", list("saveImmediateCondition()"), " returns, invisibly, the pathname of\n",
#> "the RDS written.\n"), "\n", list("\n", "Writes and Reads 'immediateCondition' RDS Files\n"),
#> "\n", list("internal"), "\n"), requestCore.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("requestCore"), "\n", list("requestCore"),
#> "\n", list("Request a core for multicore processing"),
#> "\n", list("\n", "requestCore(\n", " await,\n",
#> " workers = availableCores(constraints = \"multicore\"),\n",
#> " timeout,\n", " delta,\n", " alpha\n",
#> ")\n"), "\n", list("\n", list(list("await"),
#> list("A function used to try to \"collect\"\n",
#> "finished multicore subprocesses.")), "\n",
#> "\n", list(list("workers"), list("Total number of workers available.")),
#> "\n", "\n", list(list("timeout"), list("Maximum waiting time (in seconds) allowed\n",
#> "before a timeout error is generated.")),
#> "\n", "\n", list(list("delta"), list("Then base interval (in seconds) to wait\n",
#> "between each try.")), "\n", "\n", list(list(
#> "alpha"), list("A multiplicative factor used to increase\n",
#> "the wait interval after each try.")), "\n"),
#> "\n", list("\n", "Invisible TRUE. If no cores are available after\n",
#> "extensive waiting, then a timeout error is thrown.\n"),
#> "\n", list("\n", "If no cores are available, the current process\n",
#> "blocks until a core is available.\n"), "\n",
#> list("internal"), "\n"), reset.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-reset.R",
#> "\n", list("reset"), "\n", list("reset"), "\n",
#> list("Reset a finished, failed, canceled, or interrupted future to a lazy future"),
#> "\n", list("\n", "reset(x, ...)\n"), "\n", list(
#> "\n", list(list("x"), list("A Future.")), "\n",
#> "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", list("reset()"), " returns a lazy, vanilla ",
#> list("Future"), " that can be relaunched.\n",
#> "Resetting a running future results in a ",
#> list("FutureError"), ".\n"), "\n", list("\n",
#> "A future that has successfully completed, ",
#> list("canceled"), ", interrupted,\n", "or has failed due to an error, can be relaunched after resetting it.\n"),
#> "\n", list("\n", "A lazy, vanilla ", list("Future"),
#> " can be reused in another R session. For\n",
#> "instance, if we do:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode\">"))),
#> list("library(future)\n", "a <- 2\n", "f <- future(42 * a, lazy = TRUE)\n",
#> "saveRDS(f, \"myfuture.rds\")\n"), list(list(
#> "html"), list(list("</div>"))), "\n", "\n",
#> "Then we can read and evaluate the future in another R session using:\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("library(future)\n", "f <- readRDS(\"myfuture.rds\")\n",
#> "v <- value(f)\n", "print(v)\n", "#> [1] 84\n"),
#> list(list("html"), list(list("</div>"))), "\n"),
#> "\n", list("\n", "## Like mean(), but fails 90% of the time\n",
#> "shaky_mean <- function(x) {\n", " if (as.double(Sys.time()) %% 1 < 0.90) stop(\"boom\")\n",
#> " mean(x)\n", "}\n", "\n", "x <- rnorm(100)\n",
#> "\n", "## Calculate the mean of 'x' with a risk of failing randomly\n",
#> "f <- future({ shaky_mean(x) })\n", "\n", "## Relaunch until success\n",
#> "repeat({\n", " v <- tryCatch(value(f), error = identity)\n",
#> " if (!inherits(v, \"error\")) break\n", " message(\"Resetting failed future, and retry in 0.1 seconds\")\n",
#> " f <- reset(f)\n", " Sys.sleep(0.1)\n",
#> "})\n", "cat(\"mean:\", v, \"\\n\")\n"), "\n"),
#> resetWorkers.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-plan.R",
#> "\n", list("resetWorkers"), "\n", list("resetWorkers"),
#> "\n", list("Free up active background workers"),
#> "\n", list("\n", "resetWorkers(x, ...)\n"), "\n",
#> list("\n", list(list("x"), list("A FutureStrategy.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "Free up active background workers\n"),
#> "\n", list("\n", "This function will resolve any active futures that is currently\n",
#> "being evaluated on background workers.\n"),
#> "\n", list("\n", "resetWorkers(plan())\n", "\n"),
#> "\n", list("internal"), "\n"), resolve.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-resolve.R",
#> "\n", list("resolve"), "\n", list("resolve"),
#> "\n", list("Resolve one or more futures synchronously"),
#> "\n", list("\n", "resolve(\n", " x,\n", " idxs = NULL,\n",
#> " recursive = 0,\n", " result = FALSE,\n",
#> " stdout = FALSE,\n", " signal = FALSE,\n",
#> " force = FALSE,\n", " sleep = getOption(\"future.wait.interval\", 0.01),\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "x"), list("A ", list("Future"), " to be resolved, or a list, an environment, or a\n",
#> "list environment of futures to be resolved.")),
#> "\n", "\n", list(list("idxs"), list("(optional) integer or logical index specifying the subset of\n",
#> "elements to check.")), "\n", "\n", list(
#> list("recursive"), list("A non-negative number specifying how deep of a recursion\n",
#> "should be done. If TRUE, an infinite recursion is used. If FALSE or zero,\n",
#> "no recursion is performed.")), "\n", "\n",
#> list(list("result"), list("(internal) If TRUE, the results are ",
#> list("retrieved"), ", otherwise not.\n",
#> "Note that this only collects the results from the parallel worker, which\n",
#> "can help lower the overall latency if there are multiple concurrent futures.\n",
#> "This does ", list("not"), " return the collected results.")),
#> "\n", "\n", list(list("stdout"), list("(internal) If TRUE, captured standard output is relayed, otherwise not.")),
#> "\n", "\n", list(list("signal"), list("(internal) If TRUE, captured ",
#> list("conditions"), " are relayed,\n", "otherwise not.")),
#> "\n", "\n", list(list("force"), list("(internal) If TRUE, captured standard output and captured\n",
#> list("conditions"), " already relayed is relayed again, otherwise not.")),
#> "\n", "\n", list(list("sleep"), list("Number of seconds to wait before checking if futures have been\n",
#> "resolved since last time.")), "\n", "\n",
#> list(list(list()), list("Not used.")), "\n"),
#> "\n", list("\n", "Returns ", list("x"), " (regardless of subsetting or not).\n",
#> "If ", list("signal"), " is TRUE and one of the futures produces an error, then\n",
#> "that error is produced.\n"), "\n", list("\n",
#> "This function provides an efficient mechanism for waiting for multiple\n",
#> "futures in a container (e.g. list or environment) to be resolved while in\n",
#> "the meanwhile retrieving values of already resolved futures.\n"),
#> "\n", list("\n", "This function is resolves synchronously, i.e. it blocks until ",
#> list("x"), " and\n", "any containing futures are resolved.\n"),
#> "\n", list("\n", "To resolve a future ", list(
#> "variable"), ", first retrieve its\n", list(
#> "Future"), " object using ", list(list("futureOf()")),
#> ", e.g.\n", list("resolve(futureOf(x))"), ".\n"),
#> "\n"), resolved.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-resolved.R",
#> "\n", list("resolved"), "\n", list("resolved"),
#> "\n", list("Check whether a future is resolved or not"),
#> "\n", list("\n", "resolved(x, ...)\n"), "\n",
#> list("\n", list(list("x"), list("A ", list("Future"),
#> ", a list, or an environment (which also\n",
#> "includes ", list("list environment"), ").")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A logical of the same length and dimensions as ",
#> list("x"), ".\n", "Each element is TRUE unless the corresponding element is a\n",
#> "non-resolved future in case it is FALSE.\n"),
#> "\n", list("\n", "Check whether a future is resolved or not\n"),
#> "\n", list("\n", "This method needs to be implemented by the class that implement\n",
#> "the Future API. The implementation should return either TRUE or FALSE\n",
#> "and must never throw an error (except for ",
#> list("FutureError"), ":s which indicate\n",
#> "significant, often unrecoverable infrastructure problems).\n",
#> "It should also be possible to use the method for polling the\n",
#> "future until it is resolved (without having to wait infinitely long),\n",
#> "e.g. ", list("while (!resolved(future)) Sys.sleep(5)"),
#> ".\n"), "\n"), result.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("result.Future"), "\n", list("result.Future"),
#> "\n", list("result"), "\n", list("Get the results of a resolved future"),
#> "\n", list("\n", list(list("result"), list("Future")),
#> "(future, ...)\n"), "\n", list("\n", list(list(
#> "future"), list("A ", list("Future"), ".")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "The ", list("FutureResult"),
#> " object.\n"), "\n", list("\n", "Get the results of a resolved future\n"),
#> "\n", list("\n", "This function is only part of the ",
#> list("backend"), " Future API.\n", "This function is ",
#> list("not"), " part of the frontend Future API.\n"),
#> "\n", list("internal"), "\n"), run.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("run.Future"), "\n", list("run.Future"),
#> "\n", list("run"), "\n", list("Run a future"),
#> "\n", list("\n", list(list("run"), list("Future")),
#> "(future, ...)\n"), "\n", list("\n", list(list(
#> "future"), list("A ", list("Future"), ".")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "The ", list("Future"),
#> " object.\n"), "\n", list("\n", "Run a future\n"),
#> "\n", list("\n", "This function can only be called once per future.\n",
#> "Further calls will result in an informative error.\n",
#> "If a future is not run when its value is queried,\n",
#> "then it is run at that point.\n"), "\n", list(
#> "internal"), "\n"), save_rds.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-immediateCondition.R",
#> "\n", list("save_rds"), "\n", list("save_rds"),
#> "\n", list("Robustly Saves an Object to RDS File Atomically"),
#> "\n", list("\n", "save_rds(object, pathname, ...)\n"),
#> "\n", list("\n", list(list("object"), list("The ",
#> list(), " object to be save.")), "\n", "\n",
#> list(list("pathname"), list("RDS file to written.")),
#> "\n", "\n", list(list(list()), list("(optional) Additional arguments passed to ",
#> list(list("base::saveRDS()")), ".")), "\n"),
#> "\n", list("\n", "The pathname of the RDS written.\n"),
#> "\n", list("\n", "Robustly Saves an Object to RDS File Atomically\n"),
#> "\n", list("\n", "Uses ", list("base::saveRDS"),
#> " internally but writes the object atomically by first\n",
#> "writing to a temporary file which is then renamed.\n"),
#> "\n", list("internal"), "\n"), sequential.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.SequentialFutureBackend-class.R",
#> "\n", list("sequential"), "\n", list("sequential"),
#> "\n", list("uniprocess"), "\n", list("Create a sequential future whose value will be in the current ",
#> list(), " session"), "\n", list("\n", "sequential(..., gc = FALSE, earlySignal = FALSE, envir = parent.frame())\n"),
#> "\n", list("\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ", list(
#> "earlySignal"), " is TRUE (more\n", "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(
#> list()), list("Additional named elements to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A ", list("Future"), ".\n"), "\n",
#> list("\n", list("WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A sequential future is a future that is evaluated sequentially in the\n",
#> "current ", list(), " session similarly to how ",
#> list(), " expressions are evaluated in ", list(),
#> ".\n", "The only difference to ", list(), " itself is that globals are validated\n",
#> "by default just as for all other types of futures in this package.\n",
#> "\n", "This function is must ", list("not"),
#> " be called directly. Instead, the\n", "typical usages are:\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures sequentially in the current R process\n",
#> "plan(sequential)\n"), list(list("html"),
#> list(list("</div>"))), "\n"), "\n", list(
#> "\n", "## Use sequential futures\n", "plan(sequential)\n",
#> "\n", "## A global variable\n", "a <- 0\n",
#> "\n", "## Create a sequential future\n", "f <- future({\n",
#> " b <- 3\n", " c <- 2\n", " a * b * c\n",
#> "})\n", "\n", "## Since 'a' is a global variable in future 'f' which\n",
#> "## is eagerly resolved (default), this global has already\n",
#> "## been resolved / incorporated, and any changes to 'a'\n",
#> "## at this point will _not_ affect the value of 'f'.\n",
#> "a <- 7\n", "print(a)\n", "\n", "v <- value(f)\n",
#> "print(v)\n", "stopifnot(v == 0)\n"), "\n"),
#> sessionDetails.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-sessionDetails.R",
#> "\n", list("sessionDetails"), "\n", list("sessionDetails"),
#> "\n", list("Outputs details on the current ",
#> list(), " session"), "\n", list("\n", "sessionDetails(env = FALSE)\n"),
#> "\n", list("\n", list(list("env"), list("If TRUE, ",
#> list("Sys.getenv()"), " information is returned.")),
#> "\n"), "\n", list("\n", "Invisibly a list of all details.\n"),
#> "\n", list("\n", "Outputs details on the current ",
#> list(), " session\n"), "\n", list("internal"),
#> "\n"), signalConditions.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-signalConditions.R",
#> "\n", list("signalConditions"), "\n", list("signalConditions"),
#> "\n", list("Signals Captured Conditions"), "\n",
#> list("\n", "signalConditions(\n", " future,\n",
#> " include = \"condition\",\n", " exclude = NULL,\n",
#> " resignal = TRUE,\n", " ...\n", ")\n"),
#> "\n", list("\n", list(list("future"), list("A resolved ",
#> list("Future"), ".")), "\n", "\n", list(list(
#> "include"), list("A character string of ",
#> list("condition"), "\n", "classes to signal.")),
#> "\n", "\n", list(list("exclude"), list("A character string of ",
#> list("condition"), "\n", "classes ", list(
#> "not"), " to signal.")), "\n", "\n", list(
#> list("resignal"), list("If TRUE, then already signaled conditions are signaled\n",
#> "again, otherwise not.")), "\n", "\n",
#> list(list(list()), list("Not used.")), "\n"),
#> "\n", list("\n", "Returns the ", list("Future"),
#> " where conditioned that were signaled\n",
#> "have been flagged to have been signaled.\n"),
#> "\n", list("\n", "Captured conditions that meet the ",
#> list("include"), " and ", list("exclude"),
#> "\n", "requirements are signaled ", list("in the order as they were captured"),
#> ".\n"), "\n", list("\n", "Conditions are signaled by\n",
#> list(list("signalCondition"), "()"), ".\n"),
#> "\n", list("internal"), "\n"), sticky_globals.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-sticky_globals.R",
#> "\n", list("sticky_globals"), "\n", list("sticky_globals"),
#> "\n", list("Place a sticky-globals environment immediately after the global environment"),
#> "\n", list("\n", "sticky_globals(erase = FALSE, name = \"future:sticky_globals\", pos = 2L)\n"),
#> "\n", list("\n", list(list("erase"), list("(logical) If TRUE, the environment is erased, otherwise not.")),
#> "\n", "\n", list(list("name"), list("(character) The name of the environment on the ",
#> list("base::search"), "\n", "path.")), "\n",
#> "\n", list(list("pos"), list("(integer) The position on the search path where the\n",
#> "environment should be positioned. If ",
#> list("pos == 0L"), ", then the environment\n",
#> "is detached, if it exists.")), "\n"), "\n",
#> list("\n", "(invisible; environment) The environment.\n"),
#> "\n", list("\n", "Place a sticky-globals environment immediately after the global environment\n"),
#> "\n", list("internal"), "\n"), usedCores.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("usedCores"), "\n", list("usedCores"),
#> "\n", list("Get number of cores currently used"),
#> "\n", list("\n", "usedCores()\n"), "\n", list(
#> "\n", "A non-negative integer.\n"), "\n", list(
#> "\n", "Get number of children (and don't count the current process)\n",
#> "used by the current ", list(), " session. The number of children\n",
#> "is the total number of subprocesses launched by this\n",
#> "process that are still running and whose values have yet\n",
#> "not been collected.\n"), "\n", list("internal"),
#> "\n"), value.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-value.R",
#> "\n", list("value"), "\n", list("value"), "\n",
#> list("value.Future"), "\n", list("value.list"),
#> "\n", list("value.listenv"), "\n", list("value.environment"),
#> "\n", list("The value of a future or the values of all elements in a container"),
#> "\n", list("\n", "value(...)\n", "\n", list(list(
#> "value"), list("Future")), "(future, stdout = TRUE, signal = TRUE, drop = FALSE, ...)\n",
#> "\n", list(list("value"), list("list")), "(\n",
#> " x,\n", " idxs = NULL,\n", " recursive = 0,\n",
#> " reduce = NULL,\n", " stdout = TRUE,\n",
#> " signal = TRUE,\n", " cancel = TRUE,\n",
#> " interrupt = cancel,\n", " inorder = TRUE,\n",
#> " drop = FALSE,\n", " force = TRUE,\n", " sleep = getOption(\"future.wait.interval\", 0.01),\n",
#> " ...\n", ")\n", "\n", list(list("value"),
#> list("listenv")), "(\n", " x,\n", " idxs = NULL,\n",
#> " recursive = 0,\n", " reduce = NULL,\n",
#> " stdout = TRUE,\n", " signal = TRUE,\n",
#> " cancel = TRUE,\n", " interrupt = cancel,\n",
#> " inorder = TRUE,\n", " drop = FALSE,\n",
#> " force = TRUE,\n", " sleep = getOption(\"future.wait.interval\", 0.01),\n",
#> " ...\n", ")\n", "\n", list(list("value"),
#> list("environment")), "(x, ...)\n"), "\n",
#> list("\n", list(list("future, x"), list("A ",
#> list("Future"), ", an environment, a list, or a list environment.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE, standard output captured while resolving futures\n",
#> "is relayed, otherwise not.")), "\n", "\n",
#> list(list("signal"), list("If TRUE, ", list(
#> "conditions"), " captured while resolving\n",
#> "futures are relayed, otherwise not.")),
#> "\n", "\n", list(list("drop"), list("If TRUE, resolved futures are minimized in size and invalidated\n",
#> "as soon the as their values have been collected and any output and\n",
#> "conditions have been relayed.\n", "Combining ",
#> list("drop = TRUE"), " with ", list("inorder = FALSE"),
#> " reduces the memory use\n", "sooner, especially avoiding the risk of holding on to future values until\n",
#> "the very end.")), "\n", "\n", list(list(
#> "idxs"), list("(optional) integer or logical index specifying the subset of\n",
#> "elements to check.")), "\n", "\n", list(
#> list("recursive"), list("A non-negative number specifying how deep of a recursion\n",
#> "should be done. If TRUE, an infinite recursion is used. If FALSE or zero,\n",
#> "no recursion is performed.")), "\n", "\n",
#> list(list("reduce"), list("An optional function for reducing all the values.\n",
#> "Optional attribute ", list("init"), " can be used to set initial value for the\n",
#> "reduction. If not specified, the first value will be used as the\n",
#> "initial value.\n", "Reduction of values is done as soon as possible, but always in the\n",
#> "same order as ", list("x"), ", unless ",
#> list("inorder"), " is FALSE.")), "\n", "\n",
#> list(list("cancel, interrupt"), list("If TRUE and ",
#> list("signal"), " is TRUE, non-resolved futures\n",
#> "are canceled as soon as an error is detected in one of the futures,\n",
#> "before signaling the error. Argument ",
#> list("interrupt"), " is passed to ", list(
#> "cancel()"), "\n", "controlling whether non-resolved futures should also be interrupted.")),
#> "\n", "\n", list(list("inorder"), list("If TRUE, then standard output and conditions are relayed,\n",
#> "and value reduction, is done in the order the futures occur in ",
#> list("x"), ", but\n", "always as soon as possible. This is achieved by buffering the details\n",
#> "until they can be released. By setting ",
#> list("inorder = FALSE"), ", no buffering\n",
#> "takes place and everything is relayed and reduced as soon as a new future\n",
#> "is resolved. Regardlessly, the values are always returned in the same\n",
#> "order as ", list("x"), ".")), "\n", "\n",
#> list(list("force"), list("(internal) If TRUE, captured standard output and captured\n",
#> list("conditions"), " already relayed is relayed again, otherwise not.")),
#> "\n", "\n", list(list("sleep"), list("Number of seconds to wait before checking if futures have been\n",
#> "resolved since last time.")), "\n", "\n",
#> list(list(list()), list("All arguments used by the S3 methods.")),
#> "\n"), "\n", list("\n", list("value()"), " of a Future object returns the value of the future, which can\n",
#> "be any type of ", list(), " object.\n", "\n",
#> list("value()"), " of a list, an environment, or a list environment returns an\n",
#> "object with the same number of elements and of the same class.\n",
#> "Names and dimension attributes are preserved, if available.\n",
#> "All future elements are replaced by their corresponding ",
#> list("value()"), " values.\n", "For all other elements, the existing object is kept as-is.\n",
#> "\n", "If ", list("signal"), " is TRUE and one of the futures produces an error, then\n",
#> "that error is relayed. Any remaining, non-resolved futures in ",
#> list("x"), " are\n", "canceled, prior to signaling such an error.\n"),
#> "\n", list("\n", "Gets the value of a future or the values of all elements (including futures)\n",
#> "in a container such as a list, an environment, or a list environment.\n",
#> "If one or more futures is unresolved, then this function blocks until all\n",
#> "queried futures are resolved.\n"), "\n", list(
#> "\n", "## ------------------------------------------------------\n",
#> "## A single future\n", "## ------------------------------------------------------\n",
#> "x <- sample(100, size = 50)\n", "f <- future(mean(x))\n",
#> "v <- value(f)\n", "message(\"The average of 50 random numbers in [1,100] is: \", v)\n",
#> "\n", "\n", "\n", "## ------------------------------------------------------\n",
#> "## Ten futures\n", "## ------------------------------------------------------\n",
#> "xs <- replicate(10, { list(sample(100, size = 50)) })\n",
#> "fs <- lapply(xs, function(x) { future(mean(x)) })\n",
#> "\n", "## The 10 values as a list (because 'fs' is a list)\n",
#> "vs <- value(fs)\n", "message(\"The ten averages are:\")\n",
#> "str(vs)\n", "\n", "## The 10 values as a vector (by manually unlisting)\n",
#> "vs <- value(fs)\n", "vs <- unlist(vs)\n",
#> "message(\"The ten averages are: \", paste(vs, collapse = \", \"))\n",
#> "\n", "## The values as a vector (by reducing)\n",
#> "vs <- value(fs, reduce = `c`)\n", "message(\"The ten averages are: \", paste(vs, collapse = \", \"))\n",
#> "\n", "## Calculate the sum of the averages (by reducing)\n",
#> "total <- value(fs, reduce = `sum`)\n", "message(\"The sum of the ten averages is: \", total)\n"),
#> "\n"), `zzz-future.options.Rd` = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-options.R",
#> "\n", list("zzz-future.options"), "\n", list(
#> "zzz-future.options"), "\n", list("future.options"),
#> "\n", list("future.startup.script"), "\n", list(
#> "future.debug"), "\n", list("future.demo.mandelbrot.region"),
#> "\n", list("future.demo.mandelbrot.nrow"), "\n",
#> list("future.fork.multithreading.enable"), "\n",
#> list("future.globals.maxSize"), "\n", list("future.globals.method"),
#> "\n", list("future.globals.onMissing"), "\n",
#> list("future.globals.resolve"), "\n", list("future.globals.onReference"),
#> "\n", list("future.plan"), "\n", list("future.onFutureCondition.keepFuture"),
#> "\n", list("future.resolve.recursive"), "\n",
#> list("future.connections.onMisuse"), "\n", list(
#> "future.defaultDevice.onMisuse"), "\n", list(
#> "future.devices.onMisuse"), "\n", list("future.globalenv.onMisuse"),
#> "\n", list("future.rng.onMisuse"), "\n", list(
#> "future.wait.alpha"), "\n", list("future.wait.interval"),
#> "\n", list("future.wait.timeout"), "\n", list(
#> "future.output.windows.reencode"), "\n", list(
#> "future.journal"), "\n", list("future.globals.objectSize.method"),
#> "\n", list("future.ClusterFuture.clusterEvalQ"),
#> "\n", list("R_FUTURE_STARTUP_SCRIPT"), "\n",
#> list("R_FUTURE_DEBUG"), "\n", list("R_FUTURE_DEMO_MANDELBROT_REGION"),
#> "\n", list("R_FUTURE_DEMO_MANDELBROT_NROW"),
#> "\n", list("R_FUTURE_FORK_MULTITHREADING_ENABLE"),
#> "\n", list("R_FUTURE_GLOBALS_MAXSIZE"), "\n",
#> list("R_FUTURE_GLOBALS_METHOD"), "\n", list("R_FUTURE_GLOBALS_ONMISSING"),
#> "\n", list("R_FUTURE_GLOBALS_RESOLVE"), "\n",
#> list("R_FUTURE_GLOBALS_ONREFERENCE"), "\n", list(
#> "R_FUTURE_PLAN"), "\n", list("R_FUTURE_ONFUTURECONDITION_KEEPFUTURE"),
#> "\n", list("R_FUTURE_RESOLVE_RECURSIVE"), "\n",
#> list("R_FUTURE_CONNECTIONS_ONMISUSE"), "\n",
#> list("R_FUTURE_DEVICES_ONMISUSE"), "\n", list(
#> "R_FUTURE_DEFAULTDEVICE_ONMISUSE"), "\n", list(
#> "R_FUTURE_GLOBALENV_ONMISUSE"), "\n", list(
#> "R_FUTURE_RNG_ONMISUSE"), "\n", list("R_FUTURE_WAIT_ALPHA"),
#> "\n", list("R_FUTURE_WAIT_INTERVAL"), "\n", list(
#> "R_FUTURE_WAIT_TIMEOUT"), "\n", list("R_FUTURE_RESOLVED_TIMEOUT"),
#> "\n", list("R_FUTURE_OUTPUT_WINDOWS_REENCODE"),
#> "\n", list("R_FUTURE_JOURNAL"), "\n", list("R_FUTURE_GLOBALS_OBJECTSIZE_METHOD"),
#> "\n", list("R_FUTURE_CLUSTERFUTURE_CLUSTEREVALQ"),
#> "\n", list("future.cmdargs"), "\n", list(".future.R"),
#> "\n", list("Options used for futures"), "\n",
#> list("\n", "Below are the ", list(), " options and environment variables that are used by the\n",
#> list("future"), " package and packages enhancing it.",
#> list(), "\n", list(), "\n", list("WARNING: Note that the names and the default values of these options may\n",
#> "change in future versions of the package. Please use with care until\n",
#> "further notice."), "\n"), "\n", list(list(
#> "Packages must not change future options"),
#> list("\n", "\n", "\n", "Just like for other R options, as a package developer you must ",
#> list("not"), " change\n", "any of the below ",
#> list("future.*"), " options. Only the end-user should set these.\n",
#> "If you find yourself having to tweak one of the options, make sure to\n",
#> "undo your changes immediately afterward. For example, if you want to\n",
#> "bump up the ", list("future.globals.maxSize"),
#> " limit when creating a future,\n", "use something like the following inside your function:\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("oopts <- options(future.globals.maxSize = 1.0 * 1e9) ## 1.0 GB\n",
#> "on.exit(options(oopts))\n", "f <- future({ expr }) ## Launch a future with large objects\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n")), "\n", "\n", list(list("Options for controlling futures"),
#> list("\n", "\n", list("\n", list(list(list(
#> "future.plan"), ":"), list("(character string or future function) Default future backend used unless otherwise specified via ",
#> list(list("plan()")), ". This will also be the future plan set when calling ",
#> list("plan(\"default\")"), ". If not specified, this option may be set when the ",
#> list("future"), " package is ", list("loaded"),
#> " if command-line option ", list("--parallel=ncores"),
#> " (short ", list("-p ncores"), ") is specified; if ",
#> list("ncores > 1"), ", then option ", list(
#> "future.plan"), " is set to ", list("multisession"),
#> " otherwise ", list("sequential"), " (in addition to option ",
#> list("mc.cores"), " being set to ", list(
#> "ncores"), ", if ", list("ncores >= 1"),
#> "). (Default: ", list("sequential"), ")")),
#> "\n", "\n", list(list(list("future.globals.maxSize"),
#> ":"), list("(numeric) Maximum allowed total size (in bytes) of global variables identified. This is used to protect against exporting too large objects to parallel workers by mistake. Transferring large objects over a network, or over the internet, can be slow and therefore introduce a large bottleneck that increases the overall processing time. It can also result in large egress or ingress costs, which may exist on some systems. If set of ",
#> list("+Inf"), ", then the check for large globals is skipped. (Default: ",
#> list("500 * 1024 ^ 2"), " = 500 MiB)")),
#> "\n", "\n", list(list(list("future.globals.onReference"),
#> ": (", list("beta feature - may change"),
#> ")"), list("(character string) Controls whether the identified globals should be scanned for so called ",
#> list("references"), " (e.g. external pointers and connections) or not. It is unlikely that another ",
#> list(), " process (\"worker\") can use a global that uses a internal reference of the master ",
#> list(), " process---we call such objects ",
#> list("non-exportable globals"), ".\n",
#> "If this option is ", list("\"error\""),
#> ", an informative error message is produced if a non-exportable global is detected.\n",
#> "If ", list("\"warning\""), ", a warning is produced, but the processing will continue; it is likely that the future will be resolved with a run-time error unless processed in the master ",
#> list(), " process (e.g. ", list("plan(sequential)"),
#> " and ", list("plan(multicore)"), ").\n",
#> "If ", list("\"ignore\""), ", no scan is performed.\n",
#> "(Default: ", list("\"ignore\""), " but may change)\n")),
#> "\n", "\n", list(list(list("future.resolve.recursive"),
#> ":"), list("(integer) An integer specifying the maximum recursive depth to which futures should be resolved. If negative, nothing is resolved. If ",
#> list("0"), ", only the future itself is resolved. If ",
#> list("1"), ", the future and any of its elements that are futures are resolved, and so on. If ",
#> list("+Inf"), ", infinite search depth is used. (Default: ",
#> list("0"), ")")), "\n", "\n", list(list(
#> list("future.onFutureCondition.keepFuture"),
#> ":"), list("(logical) If ", list("TRUE"),
#> ", a ", list("FutureCondition"), " keeps a copy of the ",
#> list("Future"), " object that triggered the condition. If ",
#> list("FALSE"), ", it is dropped. (Default: ",
#> list("TRUE"), ")")), "\n", "\n", list(list(
#> list("future.wait.timeout"), ":"), list(
#> "(numeric) Maximum waiting time (in seconds) for a future to resolve or for a free worker to become available before a timeout error is generated. (Default: ",
#> list("30 * 24 * 60 * 60"), " (= 30 days))")),
#> "\n", "\n", list(list(list("future.wait.interval"),
#> ":"), list("(numeric) Initial interval (in\n",
#> "seconds) between polls. This controls the polling frequency for finding\n",
#> "an available worker when all workers are currently busy. It also controls\n",
#> "the polling frequency of ", list("resolve()"),
#> ". (Default: ", list("0.01"), " = 1 ms)")),
#> "\n", "\n", list(list(list("future.wait.alpha"),
#> ":"), list("(numeric) Positive scale factor used to increase the interval after each poll. (Default: ",
#> list("1.01"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Options for built-in sanity checks"),
#> list("\n", "\n", "\n", "Ideally, the evaluation of a future should have no side effects. To\n",
#> "protect against unexpected side effects, the future framework comes\n",
#> "with a set of built-in tools for checking against this.\n",
#> "Below R options control these built-in checks and what should happen\n",
#> "if they fail. You may modify them for troubleshooting purposes, but\n",
#> "please refrain from disabling these checks when there is an underlying\n",
#> "problem that should be fixed.\n", "\n",
#> list("Beta features: Please consider these checks to be \"under construction\"."),
#> "\n", "\n", list("\n", list(list(list("future.connections.onMisuse"),
#> ":"), list("(character string)\n", "A future must close any connections it opens and must not close\n",
#> "connections it did not open itself.\n",
#> "If such misuse is detected and this option is set to ",
#> list("\"error\""), ",\n", "then an informative error is produced. If it is set to ",
#> list("\"warning\""), ",\n", "a warning is produced. If",
#> list("\"ignore\""), ", no check is performed.\n",
#> "(Default: ", list("\"warning\""), ")\n")),
#> "\n", "\n", list(list(list("future.defaultDevice.onMisuse"),
#> ":"), list("(character string)\n", "A future must open graphics devices explicitly, if it creates new\n",
#> "plots. It should not rely on the default graphics device that\n",
#> "is given by R option ", list("\"default\""),
#> ", because that rarely does what\n",
#> "is intended.\n", "If such misuse is detected and this option is set to ",
#> list("\"error\""), ",\n", "then an informative error is produced. If it is set to ",
#> list("\"warning\""), ",\n", "a warning is produced. If",
#> list("\"ignore\""), ", no check is performed.\n",
#> "(Default: ", list("\"warning\""), ")\n")),
#> "\n", "\n", list(list(list("future.devices.onMisuse"),
#> ":"), list("(character string)\n", "A future must close any graphics devices it opens and must not close\n",
#> "devices it did not open itself.\n",
#> "If such misuse is detected and this option is set to ",
#> list("\"error\""), ",\n", "then an informative error is produced. If it is set to ",
#> list("\"warning\""), ",\n", "a warning is produced. If",
#> list("\"ignore\""), ", no check is performed.\n",
#> "(Default: ", list("\"warning\""), ")\n")),
#> "\n", "\n", list(list(list("future.globalenv.onMisuse"),
#> ":"), list("(character string)\n", "Assigning variables to the global environment for the purpose of using\n",
#> "the variable at a later time makes no sense with futures, because the\n",
#> "next the future may be evaluated in different R process.\n",
#> "To protect against mistakes, the future framework attempts to detect\n",
#> "when variables are added to the global environment.\n",
#> "If this is detected, and this option is set to ",
#> list("\"error\""), ", then an\n", "informative error is produced. If ",
#> list("\"warning\""), ", then a warning is\n",
#> "produced. If ", list("\"ignore\""),
#> ", no check is performed.\n", "(Default: ",
#> list("\"ignore\""), ")\n")), "\n", "\n",
#> list(list(list("future.rng.onMisuse"),
#> ":"), list("(character string)\n", "If random numbers are used in futures, then parallel RNG should be\n",
#> list("declared"), " in order to get statistical sound RNGs. You can declare\n",
#> "this by specifying future argument ",
#> list("seed = TRUE"), ". The defaults in the\n",
#> "future framework assume that ", list(
#> "no"), " random number generation (RNG) is\n",
#> "taken place in the future expression because L'Ecuyer-CMRG RNGs come\n",
#> "with an unnecessary overhead if not needed.\n",
#> "To protect against mistakes of not declaring use of the RNG, the\n",
#> "future framework detects when random numbers were used despite not\n",
#> "declaring such use.\n", "If this is detected, and this options is set ",
#> list("\"error\""), ", then an\n", "informative error is produced. If ",
#> list("\"warning\""), ", then a warning is\n",
#> "produced. If ", list("\"ignore\""),
#> ", no check is performed.\n", "(Default: ",
#> list("\"warning\""), ")\n")), "\n"),
#> "\n")), "\n", "\n", list(list("Options for debugging futures"),
#> list("\n", "\n", list("\n", list(list(list(
#> "future.debug"), ":"), list("(logical) If ",
#> list("TRUE"), ", extensive debug messages are generated. (Default: ",
#> list("FALSE"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Options for controlling package startup"),
#> list("\n", "\n", list("\n", list(list(list(
#> "future.startup.script"), ":"), list("(character vector or a logical) Specifies zero of more future startup scripts to be sourced when the ",
#> list("future"), " package is ", list("attached"),
#> ". It is only the first existing script that is sourced. If none of the specified files exist, nothing is sourced---there will be neither a warning nor an error.\n",
#> "If this option is not specified, environment variable ",
#> list("R_FUTURE_STARTUP_SCRIPT"), " is considered, where multiple scripts may be separated by either a colon (",
#> list(":"), ") or a semicolon (", list(";"),
#> "). If neither is set, or either is set to ",
#> list("TRUE"), ", the default is to look for a ",
#> list(".future.R"), " script in the current directory and then in the user's home directory. To disable future startup scripts, set the option or the environment variable to ",
#> list("FALSE"), ". ", list("Importantly"),
#> ", this option is ", list("always"), " set to ",
#> list("FALSE"), " if the ", list("future"),
#> " package is loaded as part of a future expression being evaluated, e.g. in a background process. In order words, they are sourced in the main ",
#> list(), " process but not in future processes. (Default: ",
#> list("TRUE"), " in main ", list(), " process and ",
#> list("FALSE"), " in future processes / during future evaluation)")),
#> "\n", "\n", list(list(list("future.cmdargs"),
#> ":"), list("(character vector) Overrides ",
#> list(list("commandArgs"), "()"), " when the ",
#> list("future"), " package is ", list("loaded"),
#> ".")), "\n"), "\n")), "\n", "\n", list(
#> list("Options for configuring low-level system behaviors"),
#> list("\n", "\n", "\n", list("\n", list(list(
#> list("future.fork.multithreading.enable"),
#> " (", list("beta feature - may change"),
#> "):"), list("(logical) Enable or disable ",
#> list("multi-threading"), " while using ",
#> list("forked"), " parallel processing. If ",
#> list("FALSE"), ", different multi-thread library settings are overridden such that they run in single-thread mode. Specifically, multi-threading will be disabled for OpenMP (which requires the ",
#> list("RhpcBLASctl"), " package) and for ",
#> list("RcppParallel"), ". If ", list("TRUE"),
#> ", or not set (the default), multi-threading is allowed. Parallelization via multi-threaded processing (done in native code by some packages and external libraries) while at the same time using forked (aka \"multicore\") parallel processing is known to unstable. Note that this is not only true when using ",
#> list("plan(multicore)"), " but also when using, for instance, ",
#> list(list("mclapply"), "()"), " of the ",
#> list("parallel"), " package. (Default: not set)")),
#> "\n", "\n", list(list(list("future.output.windows.reencode"),
#> ":"), list("(logical) Enable or disable re-encoding of UTF-8 symbols that were incorrectly encoded while captured. In R (< 4.2.0) and on older versions of MS Windows, R cannot capture UTF-8 symbols as-is when they are captured from the standard output. For examples, a UTF-8 check mark symbol (",
#> list("\"\\u2713\""), ") would be relayed as ",
#> list("\"<U+2713>\""), " (a string with eight ASCII characters). Setting this option to ",
#> list("TRUE"), " will cause ", list("value()"),
#> " to attempt to recover the intended UTF-8 symbols from ",
#> list("<U+nnnn>"), " string components, if, and only if, the string was captured by a future resolved on MS Windows. (Default: ",
#> list("TRUE"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Options for demos"), list("\n",
#> "\n", list("\n", list(list(list("future.demo.mandelbrot.region"),
#> ":"), list("(integer) Either a named list of ",
#> list(list("mandelbrot()")), " arguments or an integer in {1, 2, 3} specifying a predefined Mandelbrot region. (Default: ",
#> list("1L"), ")")), "\n", "\n", list(list(
#> list("future.demo.mandelbrot.nrow"), ":"),
#> list("(integer) Number of rows and columns of tiles. (Default: ",
#> list("3L"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Deprecated or for internal prototyping"),
#> list("\n", "\n", "\n", "The following options exists only for troubleshooting purposes and must not\n",
#> "be used in production. If used, there is a risk that the results are\n",
#> "non-reproducible if processed elsewhere. To lower the risk of them being\n",
#> "used by mistake, they are marked as deprecated and will produce warnings\n",
#> "if set.\n", "\n", list("\n", list(list(list(
#> "future.globals.onMissing"), ":"), list(
#> "(character string) Action to take when non-existing global variables (\"globals\" or \"unknowns\") are identified when the future is created. If ",
#> list("\"error\""), ", an error is generated immediately. If ",
#> list("\"ignore\""), ", no action is taken and an attempt to evaluate the future expression will be made. The latter is useful when there is a risk for false-positive globals being identified, e.g. when future expression contains non-standard evaluation (NSE). (Default: ",
#> list("\"ignore\""), ")")), "\n", "\n",
#> list(list(list("future.globals.method"),
#> ":"), list("(character string) Method used to identify globals. For details, see ",
#> list(list("globalsOf"), "()"), ". (Default: ",
#> list("\"ordered\""), ")")), "\n", "\n",
#> list(list(list("future.globals.resolve"),
#> ":"), list("(logical) If ", list("TRUE"),
#> ", globals that are ", list(list("Future")),
#> " objects (typically created as ", list(
#> "explicit"), " futures) will be resolved and have their values (using ",
#> list("value()"), ") collected. Because searching for unresolved futures among globals (including their content) can be expensive, the default is not to do it and instead leave it to the run-time checks that assert proper ownership when resolving futures and collecting their values. (Default: ",
#> list("FALSE"), ")")), "\n"), "\n")),
#> "\n", "\n", list(list("Environment variables that set R options"),
#> list("\n", "\n", "All of the above ", list(),
#> " ", list("future.*"), " options can be set by corresponding\n",
#> "environment variable ", list("R_FUTURE_*"),
#> " ", list("when the ", list("future"), " package is\n",
#> "loaded"), ". This means that those environment variables must be set before\n",
#> "the ", list("future"), " package is loaded in order to have an effect.\n",
#> "For example, if ", list("R_FUTURE_RNG_ONMISUSE=\"ignore\""),
#> ", then option\n", list("future.rng.onMisuse"),
#> " is set to ", list("\"ignore\""), " (character string).\n",
#> "Similarly, if ", list("R_FUTURE_GLOBALS_MAXSIZE=\"50000000\""),
#> ", then option\n", list("future.globals.maxSize"),
#> " is set to ", list("50000000"), " (numeric).\n")),
#> "\n", "\n", list(list("Options moved to the 'parallelly' package"),
#> list("\n", "\n", "Several functions have been moved to the ",
#> list("parallelly"), " package:\n", list("\n",
#> list(), " ", list(list("parallelly::availableCores()")),
#> "\n", list(), " ", list(list("parallelly::availableWorkers()")),
#> "\n", list(), " ", list(list("parallelly::makeClusterMPI()")),
#> "\n", list(), " ", list(list("parallelly::makeClusterPSOCK()")),
#> "\n", list(), " ", list(list("parallelly::makeNodePSOCK()")),
#> "\n", list(), " ", list(list("parallelly::supportsMulticore()")),
#> "\n"), "\n", "\n", "The options and environment variables controlling those have been adjusted\n",
#> "accordingly to have different prefixes.\n",
#> "For example, option ", list("future.fork.enable"),
#> " has been renamed to\n", list("parallelly.fork.enable"),
#> " and the corresponding environment variable\n",
#> list("R_FUTURE_FORK_ENABLE"), " has been renamed to\n",
#> list("R_PARALLELLY_FORK_ENABLE"), ".\n",
#> "For backward compatibility reasons, the ",
#> list("parallelly"), " package will\n", "support both versions for a long foreseeable time.\n",
#> "See the ", list("parallelly::parallelly.options"),
#> " page for the settings.\n")), "\n", "\n",
#> list("\n", "# Allow at most 5 MB globals per futures\n",
#> "options(future.globals.maxSize = 5e6)\n",
#> "\n", "# Be strict; catch all RNG mistakes\n",
#> "options(future.rng.onMisuse = \"error\")\n",
#> "\n", "\n"), "\n", list("\n", "To set ", list(),
#> " options or environment variables when ",
#> list(), " starts (even before the ", list("future"),
#> " package is loaded), see the ", list("Startup"),
#> " help page. The ", list(list("https://cran.r-project.org/package=startup"),
#> list(list("startup"))), " package provides a friendly mechanism for configurating ",
#> list(), "'s startup process.\n"), "\n")), source = list(
#> `Future-class.Rd` = "R/backend_api-Future-class.R",
#> FutureBackend.Rd = c("R/backend_api-01-FutureBackend-class.R",
#> "R/backend_api-03.MultiprocessFutureBackend-class.R",
#> "R/backend_api-11.ClusterFutureBackend-class.R",
#> "R/backend_api-11.MulticoreFutureBackend-class.R",
#> "R/backend_api-11.SequentialFutureBackend-class.R",
#> "R/backend_api-13.MultisessionFutureBackend-class.R"
#> ), FutureCondition.Rd = c("R/protected_api-FutureCondition-class.R",
#> "R/protected_api-journal.R"), FutureGlobals.Rd = "R/protected_api-FutureGlobals-class.R",
#> FutureResult.Rd = "R/protected_api-FutureResult-class.R",
#> `MulticoreFuture-class.Rd` = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> `MultiprocessFuture-class.Rd` = "R/backend_api-03.MultiprocessFutureBackend-class.R",
#> `UniprocessFuture-class.Rd` = "R/backend_api-UniprocessFuture-class.R",
#> backtrace.Rd = "R/utils_api-backtrace.R", cancel.Rd = "R/core_api-cancel.R",
#> cluster.Rd = "R/backend_api-11.ClusterFutureBackend-class.R",
#> clusterExportSticky.Rd = "R/utils-sticky_globals.R",
#> find_references.Rd = "R/utils-marshalling.R", future.Rd = c("R/core_api-future.R",
#> "R/utils_api-futureCall.R", "R/utils_api-minifuture.R"
#> ), futureAssign.Rd = c("R/delayed_api-futureAssign.R",
#> "R/infix_api-01-futureAssign_OP.R", "R/infix_api-02-globals_OP.R",
#> "R/infix_api-03-seed_OP.R", "R/infix_api-04-stdout_OP.R",
#> "R/infix_api-05-conditions_OP.R", "R/infix_api-06-lazy_OP.R",
#> "R/infix_api-07-label_OP.R", "R/infix_api-08-plan_OP.R",
#> "R/infix_api-09-tweak_OP.R"), futureOf.Rd = "R/delayed_api-futureOf.R",
#> futureSessionInfo.Rd = "R/utils_api-futureSessionInfo.R",
#> futures.Rd = "R/protected_api-futures.R", getExpression.Rd = "R/backend_api-Future-class.R",
#> getGlobalsAndPackages.Rd = "R/protected_api-globals.R",
#> makeClusterFuture.Rd = "R/utils_api-makeClusterFuture.R",
#> mandelbrot.Rd = "R/demo_api-mandelbrot.R", multicore.Rd = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> multisession.Rd = "R/backend_api-13.MultisessionFutureBackend-class.R",
#> nbrOfWorkers.Rd = "R/utils_api-nbrOfWorkers.R", nullcon.Rd = "R/utils-basic.R",
#> plan.Rd = c("R/utils_api-plan-with.R", "R/utils_api-plan.R",
#> "R/utils_api-tweak.R"), private_length.Rd = "R/utils-basic.R",
#> `re-exports.Rd` = "R/000.re-exports.R", readImmediateConditions.Rd = "R/utils-immediateCondition.R",
#> requestCore.Rd = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> reset.Rd = "R/core_api-reset.R", resetWorkers.Rd = "R/utils_api-plan.R",
#> resolve.Rd = "R/protected_api-resolve.R", resolved.Rd = "R/core_api-resolved.R",
#> result.Rd = "R/backend_api-Future-class.R", run.Rd = "R/backend_api-Future-class.R",
#> save_rds.Rd = "R/utils-immediateCondition.R", sequential.Rd = "R/backend_api-11.SequentialFutureBackend-class.R",
#> sessionDetails.Rd = "R/utils_api-sessionDetails.R",
#> signalConditions.Rd = "R/protected_api-signalConditions.R",
#> sticky_globals.Rd = "R/utils-sticky_globals.R", usedCores.Rd = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> value.Rd = "R/core_api-value.R", `zzz-future.options.Rd` = "R/utils-options.R"),
#> keywords = list("internal", "internal", "internal", "internal",
#> "internal", "internal", "internal", "internal", character(0),
#> character(0), character(0), "internal", "internal",
#> character(0), character(0), character(0), character(0),
#> character(0), "internal", "internal", "internal",
#> "internal", character(0), character(0), character(0),
#> "internal", character(0), "internal", "internal",
#> "internal", "internal", character(0), "internal",
#> character(0), character(0), "internal", "internal",
#> "internal", character(0), "internal", "internal",
#> "internal", "internal", character(0), character(0)),
#> concepts = list(character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0)), internal = c(TRUE, TRUE,
#> TRUE, TRUE, TRUE, TRUE, TRUE, TRUE, FALSE, FALSE, FALSE,
#> TRUE, TRUE, FALSE, FALSE, FALSE, FALSE, FALSE, TRUE,
#> TRUE, TRUE, TRUE, FALSE, FALSE, FALSE, TRUE, FALSE, TRUE,
#> TRUE, TRUE, TRUE, FALSE, TRUE, FALSE, FALSE, TRUE, TRUE,
#> TRUE, FALSE, TRUE, TRUE, TRUE, TRUE, FALSE, FALSE), lifecycle = list(
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL)),
#> tutorials = list(name = character(0), file_out = character(0),
#> title = character(0), pagetitle = character(0), url = character(0)),
#> vignettes = list(name = c("future-1-overview", "future-2-output",
#> "future-2b-backend", "future-3-topologies", "future-4-issues",
#> "future-4-non-exportable-objects", "future-5-startup", "future-6-future-api-backend-specification",
#> "future-7-for-package-developers", "future-8-how-future-is-validated"
#> ), type = c("rmd", "rmd", "rmd", "rmd", "rmd", "rmd", "rmd",
#> "rmd", "rmd", "rmd"), file_in = c("vignettes/future-1-overview.Rmd",
#> "vignettes/future-2-output.Rmd", "vignettes/future-2b-backend.Rmd",
#> "vignettes/future-3-topologies.Rmd", "vignettes/future-4-issues.Rmd",
#> "vignettes/future-4-non-exportable-objects.Rmd", "vignettes/future-5-startup.Rmd",
#> "vignettes/future-6-future-api-backend-specification.Rmd",
#> "vignettes/future-7-for-package-developers.Rmd", "vignettes/future-8-how-future-is-validated.Rmd"
#> ), file_out = c("articles/future-1-overview.html", "articles/future-2-output.html",
#> "articles/future-2b-backend.html", "articles/future-3-topologies.html",
#> "articles/future-4-issues.html", "articles/future-4-non-exportable-objects.html",
#> "articles/future-5-startup.html", "articles/future-6-future-api-backend-specification.html",
#> "articles/future-7-for-package-developers.html", "articles/future-8-how-future-is-validated.html"
#> ), title = c("A Future for R: A Comprehensive Overview",
#> "A Future for R: Text and Message Output", "A Future for R: Available Future Backends",
#> "A Future for R: Future Topologies", "A Future for R: Common Issues with Solutions",
#> "A Future for R: Non-Exportable Objects", "A Future for R: Controlling Default Future Strategy",
#> "A Future for R: Future API Backend Specification", "A Future for R: Best Practices for Package Developers",
#> "A Future for R: How the Future Framework is Validated"),
#> description = c(NA_character_, NA_character_, NA_character_,
#> NA_character_, NA_character_, NA_character_, NA_character_,
#> NA_character_, NA_character_, NA_character_), depth = c(1L,
#> 1L, 1L, 1L, 1L, 1L, 1L, 1L, 1L, 1L))), examples = TRUE,
#> run_dont_run = FALSE, seed = 1014L, lazy = FALSE, override = list(),
#> install = FALSE, preview = FALSE, new_process = FALSE, devel = FALSE,
#> cli_colors = 16777216L, hyperlinks = TRUE)), envir = base::quote(<environment>),
#> quote = base::quote(TRUE))
#>
#> [[12]]
#> (function (..., cli_colors, hyperlinks)
#> {
#> options(cli.num_colors = cli_colors, cli.hyperlink = hyperlinks,
#> cli.hyperlink_run = hyperlinks)
#> pkgdown::build_site(...)
#> })(pkg = base::quote(list(package = "future", version = "1.58.0",
#> src_path = "/tmp/henrik/RtmpJraagA/future", meta = list(url = "https://future.futureverse.org",
#> home = list(links = list(list(text = "Roadmap/Milestones",
#> href = "https://github.com/futureverse/future/milestones"),
#> list(text = "The Futureverse Project", href = "https://www.futureverse.org/"),
#> list(text = "Futureverse User Forum", href = "https://github.com/futureverse/future/discussions"))),
#> navbar = list(structure = list(right = c("search", "futureverse",
#> "pkgs", "cran", "github", "lightswitch")), components = list(
#> futureverse = list(icon = "fas fa-home", href = "https://www.futureverse.org/"),
#> pkgs = list(text = "Packages", menu = list(list(text = "doFuture (map-reduce)",
#> href = "https://doFuture.futureverse.org"), list(
#> text = "furrr (map-reduce)", href = "https://furrr.futureverse.org"),
#> list(text = "future", href = "https://future.futureverse.org"),
#> list(text = "future.apply (map-reduce)", href = "https://future.apply.futureverse.org"),
#> list(text = "future.batchtools (backend)", href = "https://future.batchtools.futureverse.org"),
#> list(text = "future.callr (backend)", href = "https://future.callr.futureverse.org"),
#> list(text = "future.mirai (backend)", href = "https://future.mirai.futureverse.org"),
#> list(text = "future.tests", href = "https://future.tests.futureverse.org"),
#> list(text = "globals", href = "https://globals.futureverse.org"),
#> list(text = "listenv", href = "https://listenv.futureverse.org"),
#> list(text = "parallelly", href = "https://parallelly.futureverse.org"),
#> list(text = "progressr", href = "https://progressr.futureverse.org"),
#> list(text = "BiocParallel.FutureParam (experimental)",
#> href = "https://BiocParallel.FutureParam.futureverse.org"),
#> list(text = "future.tools (experimental)", href = "https://future.tools.futureverse.org"),
#> list(text = "future.mapreduce (experimental)",
#> href = "https://future.mapreduce.futureverse.org"),
#> list(text = "marshal (experimental)", href = "https://marshal.futureverse.org"))),
#> cran = list(icon = "fab fa-r-project", href = "https://cloud.r-project.org/package=future"))),
#> search = list(exclude = "README_ja.md"), template = list(
#> params = list(docsearch = list(api_key = "aa6e02fc501886fb0f7c91ac4e300456",
#> index_name = "futureverse", algoliaOptions = list(
#> facetFilters = "project:future")), ganalytics = "G-SB3EQSD9FR"),
#> bootstrap = 5L, `light-switch` = TRUE)), desc = <environment>,
#> bs_version = 5L, development = list(destination = "dev",
#> mode = "default", version_label = "muted", in_dev = FALSE,
#> prefix = ""), prefix = "", dst_path = "/tmp/henrik/RtmpJraagA/future/docs",
#> lang = "en-US", install_metadata = FALSE, figures = list(
#> dev = "ragg::agg_png", dpi = 96L, dev.args = list(),
#> fig.ext = "png", fig.width = 7.29166666666667, fig.height = NULL,
#> fig.retina = 2L, fig.asp = 0.618046971569839, bg = NULL,
#> other.parameters = list()), repo = list(url = list(home = "https://github.com/futureverse/future/",
#> source = "https://github.com/futureverse/future/blob/HEAD/",
#> issue = "https://github.com/futureverse/future/issues/",
#> user = "https://github.com/")), topics = list(name = c(`Future-class.Rd` = "Future-class",
#> FutureBackend.Rd = "FutureBackend", FutureCondition.Rd = "FutureCondition",
#> FutureGlobals.Rd = "FutureGlobals", FutureResult.Rd = "FutureResult",
#> `MulticoreFuture-class.Rd` = "MulticoreFuture-class", `MultiprocessFuture-class.Rd` = "MultiprocessFuture-class",
#> `UniprocessFuture-class.Rd` = "UniprocessFuture-class", backtrace.Rd = "backtrace",
#> cancel.Rd = "cancel", cluster.Rd = "cluster", clusterExportSticky.Rd = "clusterExportSticky",
#> find_references.Rd = "find_references", future.Rd = "future",
#> futureAssign.Rd = "futureAssign", futureOf.Rd = "futureOf",
#> futureSessionInfo.Rd = "futureSessionInfo", futures.Rd = "futures",
#> getExpression.Rd = "getExpression", getGlobalsAndPackages.Rd = "getGlobalsAndPackages",
#> makeClusterFuture.Rd = "makeClusterFuture", mandelbrot.Rd = "mandelbrot",
#> multicore.Rd = "multicore", multisession.Rd = "multisession",
#> nbrOfWorkers.Rd = "nbrOfWorkers", nullcon.Rd = "nullcon",
#> plan.Rd = "with.FutureStrategyList", private_length.Rd = ".length",
#> `re-exports.Rd` = "re-exports", readImmediateConditions.Rd = "readImmediateConditions",
#> requestCore.Rd = "requestCore", reset.Rd = "reset", resetWorkers.Rd = "resetWorkers",
#> resolve.Rd = "resolve", resolved.Rd = "resolved", result.Rd = "result.Future",
#> run.Rd = "run.Future", save_rds.Rd = "save_rds", sequential.Rd = "sequential",
#> sessionDetails.Rd = "sessionDetails", signalConditions.Rd = "signalConditions",
#> sticky_globals.Rd = "sticky_globals", usedCores.Rd = "usedCores",
#> value.Rd = "value", `zzz-future.options.Rd` = "zzz-future.options"
#> ), file_in = c("Future-class.Rd", "FutureBackend.Rd", "FutureCondition.Rd",
#> "FutureGlobals.Rd", "FutureResult.Rd", "MulticoreFuture-class.Rd",
#> "MultiprocessFuture-class.Rd", "UniprocessFuture-class.Rd",
#> "backtrace.Rd", "cancel.Rd", "cluster.Rd", "clusterExportSticky.Rd",
#> "find_references.Rd", "future.Rd", "futureAssign.Rd", "futureOf.Rd",
#> "futureSessionInfo.Rd", "futures.Rd", "getExpression.Rd",
#> "getGlobalsAndPackages.Rd", "makeClusterFuture.Rd", "mandelbrot.Rd",
#> "multicore.Rd", "multisession.Rd", "nbrOfWorkers.Rd", "nullcon.Rd",
#> "plan.Rd", "private_length.Rd", "re-exports.Rd", "readImmediateConditions.Rd",
#> "requestCore.Rd", "reset.Rd", "resetWorkers.Rd", "resolve.Rd",
#> "resolved.Rd", "result.Rd", "run.Rd", "save_rds.Rd", "sequential.Rd",
#> "sessionDetails.Rd", "signalConditions.Rd", "sticky_globals.Rd",
#> "usedCores.Rd", "value.Rd", "zzz-future.options.Rd"), file_out = c("Future-class.html",
#> "FutureBackend.html", "FutureCondition.html", "FutureGlobals.html",
#> "FutureResult.html", "MulticoreFuture-class.html", "MultiprocessFuture-class.html",
#> "UniprocessFuture-class.html", "backtrace.html", "cancel.html",
#> "cluster.html", "clusterExportSticky.html", "find_references.html",
#> "future.html", "futureAssign.html", "futureOf.html", "futureSessionInfo.html",
#> "futures.html", "getExpression.html", "getGlobalsAndPackages.html",
#> "makeClusterFuture.html", "mandelbrot.html", "multicore.html",
#> "multisession.html", "nbrOfWorkers.html", "nullcon.html",
#> "plan.html", "private_length.html", "re-exports.html", "readImmediateConditions.html",
#> "requestCore.html", "reset.html", "resetWorkers.html", "resolve.html",
#> "resolved.html", "result.html", "run.html", "save_rds.html",
#> "sequential.html", "sessionDetails.html", "signalConditions.html",
#> "sticky_globals.html", "usedCores.html", "value.html", "zzz-future.options.html"
#> ), alias = list(`Future-class.Rd` = c("Future-class", "Future"
#> ), FutureBackend.Rd = c("FutureBackend", "launchFuture",
#> "listFutures", "interruptFuture", "validateFutureGlobals",
#> "stopWorkers", "MultiprocessFutureBackend", "ClusterFutureBackend",
#> "MulticoreFutureBackend", "SequentialFutureBackend", "MultisessionFutureBackend"
#> ), FutureCondition.Rd = c("FutureCondition", "FutureMessage",
#> "FutureWarning", "FutureError", "RngFutureCondition", "RngFutureWarning",
#> "RngFutureError", "UnexpectedFutureResultError", "GlobalEnvMisuseFutureCondition",
#> "GlobalEnvMisuseFutureWarning", "GlobalEnvMisuseFutureError",
#> "ConnectionMisuseFutureCondition", "ConnectionMisuseFutureWarning",
#> "ConnectionMisuseFutureError", "DeviceMisuseFutureCondition",
#> "DeviceMisuseFutureWarning", "DeviceMisuseFutureError", "DefaultDeviceMisuseFutureCondition",
#> "DefaultDeviceMisuseFutureWarning", "DefaultDeviceMisuseFutureError",
#> "FutureLaunchError", "FutureInterruptError", "FutureCanceledError",
#> "FutureDroppedError", "FutureJournalCondition"), FutureGlobals.Rd = c("FutureGlobals",
#> "as.FutureGlobals", "as.FutureGlobals.FutureGlobals", "as.FutureGlobals.Globals",
#> "as.FutureGlobals.list", "[.FutureGlobals", "c.FutureGlobals",
#> "unique.FutureGlobals"), FutureResult.Rd = c("FutureResult",
#> "as.character.FutureResult", "print.FutureResult"), `MulticoreFuture-class.Rd` = c("MulticoreFuture-class",
#> "resolved.MulticoreFuture"), `MultiprocessFuture-class.Rd` = c("MultiprocessFuture-class",
#> "MultiprocessFuture"), `UniprocessFuture-class.Rd` = c("UniprocessFuture-class",
#> "UniprocessFuture"), backtrace.Rd = "backtrace", cancel.Rd = "cancel",
#> cluster.Rd = "cluster", clusterExportSticky.Rd = "clusterExportSticky",
#> find_references.Rd = c("find_references", "assert_no_references"
#> ), future.Rd = c("future", "futureCall", "minifuture"
#> ), futureAssign.Rd = c("futureAssign", "%<-%", "%->%",
#> "%globals%", "%packages%", "%seed%", "%stdout%", "%conditions%",
#> "%lazy%", "%label%", "%plan%", "%tweak%"), futureOf.Rd = "futureOf",
#> futureSessionInfo.Rd = "futureSessionInfo", futures.Rd = "futures",
#> getExpression.Rd = c("getExpression", "getExpression.Future"
#> ), getGlobalsAndPackages.Rd = "getGlobalsAndPackages",
#> makeClusterFuture.Rd = c("makeClusterFuture", "FUTURE"
#> ), mandelbrot.Rd = c("mandelbrot", "as.raster.Mandelbrot",
#> "plot.Mandelbrot", "mandelbrot_tiles", "mandelbrot.matrix",
#> "mandelbrot.numeric"), multicore.Rd = "multicore", multisession.Rd = "multisession",
#> nbrOfWorkers.Rd = c("nbrOfWorkers", "nbrOfFreeWorkers"
#> ), nullcon.Rd = "nullcon", plan.Rd = c("with.FutureStrategyList",
#> "plan", "tweak"), private_length.Rd = ".length", `re-exports.Rd` = c("re-exports",
#> "as.cluster", "availableCores", "availableWorkers", "makeClusterPSOCK",
#> "supportsMulticore"), readImmediateConditions.Rd = c("readImmediateConditions",
#> "saveImmediateCondition"), requestCore.Rd = "requestCore",
#> reset.Rd = "reset", resetWorkers.Rd = "resetWorkers",
#> resolve.Rd = "resolve", resolved.Rd = "resolved", result.Rd = c("result.Future",
#> "result"), run.Rd = c("run.Future", "run"), save_rds.Rd = "save_rds",
#> sequential.Rd = c("sequential", "uniprocess"), sessionDetails.Rd = "sessionDetails",
#> signalConditions.Rd = "signalConditions", sticky_globals.Rd = "sticky_globals",
#> usedCores.Rd = "usedCores", value.Rd = c("value", "value.Future",
#> "value.list", "value.listenv", "value.environment"),
#> `zzz-future.options.Rd` = c("zzz-future.options", "future.options",
#> "future.startup.script", "future.debug", "future.demo.mandelbrot.region",
#> "future.demo.mandelbrot.nrow", "future.fork.multithreading.enable",
#> "future.globals.maxSize", "future.globals.method", "future.globals.onMissing",
#> "future.globals.resolve", "future.globals.onReference",
#> "future.plan", "future.onFutureCondition.keepFuture",
#> "future.resolve.recursive", "future.connections.onMisuse",
#> "future.defaultDevice.onMisuse", "future.devices.onMisuse",
#> "future.globalenv.onMisuse", "future.rng.onMisuse", "future.wait.alpha",
#> "future.wait.interval", "future.wait.timeout", "future.output.windows.reencode",
#> "future.journal", "future.globals.objectSize.method",
#> "future.ClusterFuture.clusterEvalQ", "R_FUTURE_STARTUP_SCRIPT",
#> "R_FUTURE_DEBUG", "R_FUTURE_DEMO_MANDELBROT_REGION",
#> "R_FUTURE_DEMO_MANDELBROT_NROW", "R_FUTURE_FORK_MULTITHREADING_ENABLE",
#> "R_FUTURE_GLOBALS_MAXSIZE", "R_FUTURE_GLOBALS_METHOD",
#> "R_FUTURE_GLOBALS_ONMISSING", "R_FUTURE_GLOBALS_RESOLVE",
#> "R_FUTURE_GLOBALS_ONREFERENCE", "R_FUTURE_PLAN", "R_FUTURE_ONFUTURECONDITION_KEEPFUTURE",
#> "R_FUTURE_RESOLVE_RECURSIVE", "R_FUTURE_CONNECTIONS_ONMISUSE",
#> "R_FUTURE_DEVICES_ONMISUSE", "R_FUTURE_DEFAULTDEVICE_ONMISUSE",
#> "R_FUTURE_GLOBALENV_ONMISUSE", "R_FUTURE_RNG_ONMISUSE",
#> "R_FUTURE_WAIT_ALPHA", "R_FUTURE_WAIT_INTERVAL", "R_FUTURE_WAIT_TIMEOUT",
#> "R_FUTURE_RESOLVED_TIMEOUT", "R_FUTURE_OUTPUT_WINDOWS_REENCODE",
#> "R_FUTURE_JOURNAL", "R_FUTURE_GLOBALS_OBJECTSIZE_METHOD",
#> "R_FUTURE_CLUSTERFUTURE_CLUSTEREVALQ", "future.cmdargs",
#> ".future.R")), funs = list(`Future-class.Rd` = "Future()",
#> FutureBackend.Rd = c("FutureBackend()", "launchFuture()",
#> "listFutures()", "interruptFuture()", "validateFutureGlobals()",
#> "stopWorkers()", "MultiprocessFutureBackend()", "ClusterFutureBackend()",
#> "MulticoreFutureBackend()", "SequentialFutureBackend()",
#> "MultisessionFutureBackend()"), FutureCondition.Rd = c("FutureCondition()",
#> "FutureMessage()", "FutureWarning()", "FutureError()",
#> "RngFutureCondition()", "RngFutureWarning()", "RngFutureError()",
#> "UnexpectedFutureResultError()", "GlobalEnvMisuseFutureCondition()",
#> "GlobalEnvMisuseFutureWarning()", "GlobalEnvMisuseFutureError()",
#> "ConnectionMisuseFutureCondition()", "ConnectionMisuseFutureWarning()",
#> "ConnectionMisuseFutureError()", "DeviceMisuseFutureCondition()",
#> "DeviceMisuseFutureWarning()", "DeviceMisuseFutureError()",
#> "DefaultDeviceMisuseFutureCondition()", "DefaultDeviceMisuseFutureWarning()",
#> "DefaultDeviceMisuseFutureError()", "FutureLaunchError()",
#> "FutureInterruptError()", "FutureCanceledError()", "FutureDroppedError()",
#> "FutureJournalCondition()"), FutureGlobals.Rd = "FutureGlobals()",
#> FutureResult.Rd = c("FutureResult()", "as.character(<i><FutureResult></i>)",
#> "print(<i><FutureResult></i>)"), `MulticoreFuture-class.Rd` = "resolved(<i><MulticoreFuture></i>)",
#> `MultiprocessFuture-class.Rd` = "MultiprocessFuture()",
#> `UniprocessFuture-class.Rd` = "UniprocessFuture()", backtrace.Rd = "backtrace()",
#> cancel.Rd = "cancel()", cluster.Rd = "cluster()", clusterExportSticky.Rd = "clusterExportSticky()",
#> find_references.Rd = c("find_references()", "assert_no_references()"
#> ), future.Rd = c("future()", "futureCall()", "minifuture()"
#> ), futureAssign.Rd = c("futureAssign()", "`%<-%`",
#> "`%globals%`", "`%packages%`", "`%seed%`", "`%stdout%`",
#> "`%conditions%`", "`%lazy%`", "`%label%`", "`%plan%`",
#> "`%tweak%`"), futureOf.Rd = "futureOf()", futureSessionInfo.Rd = "futureSessionInfo()",
#> futures.Rd = "futures()", getExpression.Rd = "getExpression()",
#> getGlobalsAndPackages.Rd = "getGlobalsAndPackages()",
#> makeClusterFuture.Rd = "makeClusterFuture()", mandelbrot.Rd = "mandelbrot()",
#> multicore.Rd = "multicore()", multisession.Rd = "multisession()",
#> nbrOfWorkers.Rd = c("nbrOfWorkers()", "nbrOfFreeWorkers()"
#> ), nullcon.Rd = "nullcon()", plan.Rd = c("with(<i><FutureStrategyList></i>)",
#> "plan()", "tweak()"), private_length.Rd = ".length()",
#> `re-exports.Rd` = character(0), readImmediateConditions.Rd = c("readImmediateConditions()",
#> "saveImmediateCondition()"), requestCore.Rd = "requestCore()",
#> reset.Rd = "reset()", resetWorkers.Rd = "resetWorkers()",
#> resolve.Rd = "resolve()", resolved.Rd = "resolved()",
#> result.Rd = "result(<i><Future></i>)", run.Rd = "run(<i><Future></i>)",
#> save_rds.Rd = "save_rds()", sequential.Rd = "sequential()",
#> sessionDetails.Rd = "sessionDetails()", signalConditions.Rd = "signalConditions()",
#> sticky_globals.Rd = "sticky_globals()", usedCores.Rd = "usedCores()",
#> value.Rd = "value()", `zzz-future.options.Rd` = character(0)),
#> title = c(`Future-class.Rd` = "A future represents a value that will be available at some point in the future",
#> FutureBackend.Rd = "Configure a backend that controls how and where futures are evaluated",
#> FutureCondition.Rd = "A condition (message, warning, or error) that occurred while orchestrating a future",
#> FutureGlobals.Rd = "A representation of a set of globals used with futures",
#> FutureResult.Rd = "Results from resolving a future",
#> `MulticoreFuture-class.Rd` = "A multicore future is a future whose value will be resolved asynchronously in a parallel process",
#> `MultiprocessFuture-class.Rd` = "A multiprocess future is a future whose value will be resolved asynchronously in a parallel process",
#> `UniprocessFuture-class.Rd` = "An uniprocess future is a future whose value will be resolved synchronously in the current process",
#> backtrace.Rd = "Back trace the expressions evaluated when an error was caught",
#> cancel.Rd = "Cancel a future", cluster.Rd = "Create a cluster future whose value will be resolved asynchronously in a parallel process",
#> clusterExportSticky.Rd = "Export globals to the sticky-globals environment of the cluster nodes",
#> find_references.Rd = "Get the first or all references of an <span style=\"R\">R</span> object",
#> future.Rd = "Create a future", futureAssign.Rd = "Create a future assignment",
#> futureOf.Rd = "Get the future of a future variable",
#> futureSessionInfo.Rd = "Get future-specific session information and validate current backend",
#> futures.Rd = "Get all futures in a container", getExpression.Rd = "Inject code for the next type of future to use for nested futures",
#> getGlobalsAndPackages.Rd = "Retrieves global variables of an expression and their associated packages",
#> makeClusterFuture.Rd = "Create a Future Cluster of Stateless Workers for Parallel Processing",
#> mandelbrot.Rd = "Mandelbrot convergence counts", multicore.Rd = "Create a multicore future whose value will be resolved asynchronously in a forked parallel process",
#> multisession.Rd = "Create a multisession future whose value will be resolved asynchronously in a parallel <span style=\"R\">R</span> session",
#> nbrOfWorkers.Rd = "Get the number of workers available",
#> nullcon.Rd = "Creates a connection to the system null device",
#> plan.Rd = "Evaluate an expression using a temporarily set future plan",
#> private_length.Rd = "Gets the length of an object without dispatching",
#> `re-exports.Rd` = "Functions Moved to 'parallelly'",
#> readImmediateConditions.Rd = "Writes and Reads 'immediateCondition' RDS Files",
#> requestCore.Rd = "Request a core for multicore processing",
#> reset.Rd = "Reset a finished, failed, canceled, or interrupted future to a lazy future",
#> resetWorkers.Rd = "Free up active background workers",
#> resolve.Rd = "Resolve one or more futures synchronously",
#> resolved.Rd = "Check whether a future is resolved or not",
#> result.Rd = "Get the results of a resolved future", run.Rd = "Run a future",
#> save_rds.Rd = "Robustly Saves an Object to RDS File Atomically",
#> sequential.Rd = "Create a sequential future whose value will be in the current <span style=\"R\">R</span> session",
#> sessionDetails.Rd = "Outputs details on the current <span style=\"R\">R</span> session",
#> signalConditions.Rd = "Signals Captured Conditions",
#> sticky_globals.Rd = "Place a sticky-globals environment immediately after the global environment",
#> usedCores.Rd = "Get number of cores currently used",
#> value.Rd = "The value of a future or the values of all elements in a container",
#> `zzz-future.options.Rd` = "Options used for futures"),
#> rd = list(`Future-class.Rd` = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("Future-class"), "\n", list("Future-class"),
#> "\n", list("Future"), "\n", list("A future represents a value that will be available at some point in the future"),
#> "\n", list("\n", "Future(\n", " expr = NULL,\n",
#> " envir = parent.frame(),\n", " substitute = TRUE,\n",
#> " stdout = TRUE,\n", " conditions = \"condition\",\n",
#> " globals = list(),\n", " packages = NULL,\n",
#> " seed = FALSE,\n", " lazy = FALSE,\n", " gc = FALSE,\n",
#> " earlySignal = FALSE,\n", " label = NULL,\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "expr"), list("An ", list(), " ", list("expression"),
#> ".")), "\n", "\n", list(list("envir"), list("The ",
#> list("environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list("substitute"),
#> list("If TRUE, argument ", list("expr"), " is\n",
#> list(list("substitute"), "()"), ":ed, otherwise not.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE (default), then the standard output is captured,\n",
#> "and re-outputted when ", list("value()"),
#> " is called.\n", "If FALSE, any output is silenced (by sinking it to the null device as\n",
#> "it is outputted).\n", "Using ", list("stdout = structure(TRUE, drop = TRUE)"),
#> " causes the captured\n", "standard output to be dropped from the future object as soon as it has\n",
#> "been relayed. This can help decrease the overall memory consumed by\n",
#> "captured output across futures.\n", "Using ",
#> list("stdout = NA"), " fully avoids intercepting the standard output;\n",
#> "behavior of such unhandled standard output depends on the future backend.")),
#> "\n", "\n", list(list("conditions"), list("A character string of conditions classes to be captured\n",
#> "and relayed. The default is to relay all conditions, including messages\n",
#> "and warnings. To drop all conditions, use ",
#> list("conditions = character(0)"), ".\n", "Errors are always relayed.\n",
#> "Attribute ", list("exclude"), " can be used to ignore specific classes, e.g.\n",
#> list("conditions = structure(\"condition\", exclude = \"message\")"),
#> " will capture\n", "all ", list("condition"),
#> " classes except those that inherits from the ",
#> list("message"), " class.\n", "Using ", list(
#> "conditions = structure(..., drop = TRUE)"),
#> " causes any captured\n", "conditions to be dropped from the future object as soon as it has\n",
#> "been relayed, e.g. by ", list("value(f)"),
#> ". This can help decrease the overall\n", "memory consumed by captured conditions across futures.\n",
#> "Using ", list("conditions = NULL"), " (not recommended) avoids intercepting conditions,\n",
#> "except from errors; behavior of such unhandled conditions depends on the\n",
#> "future backend and the environment from which R runs.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, or a named list\n",
#> "to control how globals are handled.\n", "For details, see section 'Globals used by future expressions'\n",
#> "in the help for ", list(list("future()")),
#> ".")), "\n", "\n", list(list("packages"), list(
#> "(optional) a character vector specifying packages\n",
#> "to be attached in the ", list(), " environment evaluating the future.")),
#> "\n", "\n", list(list("seed"), list("(optional) If TRUE, the random seed, that is, the state of the\n",
#> "random number generator (RNG) will be set such that statistically sound\n",
#> "random numbers are produced (also during parallelization).\n",
#> "If FALSE (default), it is assumed that the future expression does neither\n",
#> "need nor use random numbers generation.\n",
#> "To use a fixed random seed, specify a L'Ecuyer-CMRG seed (seven integer)\n",
#> "or a regular RNG seed (a single integer). If the latter, then a\n",
#> "L'Ecuyer-CMRG seed will be automatically created based on the given seed.\n",
#> "Furthermore, if FALSE, then the future will be monitored to make sure it\n",
#> "does not use random numbers. If it does and depending on the value of\n",
#> "option ", list("future.rng.onMisuse"), ", the check is\n",
#> "ignored, an informative warning, or error will be produced.\n",
#> "If ", list("seed"), " is NULL, then the effect is as with ",
#> list("seed = FALSE"), "\n", "but without the RNG check being performed.")),
#> "\n", "\n", list(list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ", list(
#> "earlySignal"), " is TRUE (more\n", "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list("Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(list(
#> "label"), list("A character string label attached to the future.")),
#> "\n", "\n", list(list(list()), list("Additional named elements of the future.")),
#> "\n"), "\n", list("\n", list("Future()"), " returns an object of class ",
#> list("Future"), ".\n"), "\n", list("\n", "A ",
#> list("future"), " is an abstraction for a ",
#> list("value"), " that may\n", "available at some point in the future. A future can either be\n",
#> list("unresolved"), " or ", list("resolved"),
#> ", a state which can be checked\n", "with ",
#> list(list("resolved()")), ". As long as it is ",
#> list("unresolved"), ", the\n", "value is not available. As soon as it is ",
#> list("resolved"), ", the value\n", "is available via ",
#> list(list("value"), "()"), ".\n"), "\n", list(
#> "\n", "A Future object is itself an ", list("environment"),
#> ".\n"), "\n", list("\n", "One function that creates a Future is ",
#> list(list("future()")), ".\n", "It returns a Future that evaluates an ",
#> list(), " expression in the future.\n", "An alternative approach is to use the ",
#> list(list("%<-%")), " infix\n", "assignment operator, which creates a future from the\n",
#> "right-hand-side (RHS) ", list(), " expression and assigns its future value\n",
#> "to a variable as a ", list(list("promise")),
#> ".\n"), "\n", list("internal"), "\n"), FutureBackend.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/backend_api-01-FutureBackend-class.R,",
#> "\n", "% R/backend_api-03.MultiprocessFutureBackend-class.R,",
#> "\n", "% R/backend_api-11.ClusterFutureBackend-class.R,",
#> "\n", "% R/backend_api-11.MulticoreFutureBackend-class.R,",
#> "\n", "% R/backend_api-11.SequentialFutureBackend-class.R,",
#> "\n", "% R/backend_api-13.MultisessionFutureBackend-class.R",
#> "\n", list("FutureBackend"), "\n", list("FutureBackend"),
#> "\n", list("launchFuture"), "\n", list("listFutures"),
#> "\n", list("interruptFuture"), "\n", list("validateFutureGlobals"),
#> "\n", list("stopWorkers"), "\n", list("MultiprocessFutureBackend"),
#> "\n", list("ClusterFutureBackend"), "\n", list("MulticoreFutureBackend"),
#> "\n", list("SequentialFutureBackend"), "\n", list(
#> "MultisessionFutureBackend"), "\n", list("Configure a backend that controls how and where futures are evaluated"),
#> "\n", list("\n", "FutureBackend(\n", " ...,\n",
#> " earlySignal = FALSE,\n", " gc = FALSE,\n",
#> " maxSizeOfObjects = getOption(\"future.globals.maxSize\", +Inf),\n",
#> " interrupts = TRUE,\n", " hooks = FALSE\n",
#> ")\n", "\n", "launchFuture(backend, future, ...)\n",
#> "\n", "listFutures(backend, ...)\n", "\n", "interruptFuture(backend, future, ...)\n",
#> "\n", "validateFutureGlobals(backend, future, ...)\n",
#> "\n", "stopWorkers(backend, ...)\n", "\n", "MultiprocessFutureBackend(\n",
#> " ...,\n", " wait.timeout = getOption(\"future.wait.timeout\", 24 * 60 * 60),\n",
#> " wait.interval = getOption(\"future.wait.interval\", 0.01),\n",
#> " wait.alpha = getOption(\"future.wait.alpha\", 1.01)\n",
#> ")\n", "\n", "ClusterFutureBackend(\n", " workers = availableWorkers(constraints = \"connections\"),\n",
#> " gc = TRUE,\n", " earlySignal = TRUE,\n",
#> " interrupts = FALSE,\n", " persistent = FALSE,\n",
#> " ...\n", ")\n", "\n", "MulticoreFutureBackend(\n",
#> " workers = availableCores(constraints = \"multicore\"),\n",
#> " maxSizeOfObjects = +Inf,\n", " ...\n", ")\n",
#> "\n", "SequentialFutureBackend(..., maxSizeOfObjects = +Inf)\n",
#> "\n", "MultisessionFutureBackend(\n", " workers = availableCores(),\n",
#> " rscript_libs = .libPaths(),\n", " interrupts = TRUE,\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "earlySignal"), list("Overrides the default behavior on whether futures\n",
#> "should resignal (\"relay\") conditions captured as soon as possible, or\n",
#> "delayed, for instance, until ", list(list("value()")),
#> " is called on the future.\n", "(Default: ",
#> list("FALSE"), ")")), "\n", "\n", list(list("gc"),
#> list("Overrides the default behavior of whether futures should trigger\n",
#> "garbage collection via ", list(list("gc()")),
#> " on the parallel worker after the value has\n",
#> "been collected from the worker.\n", "This can help to release memory sooner than letting R itself on the parallel\n",
#> "worker decided when it is needed. Releasing memory sooner can help to fit\n",
#> "more parallel workers on a machine with limited amount of total memory.\n",
#> "(Default: ", list("FALSE"), ")")), "\n", "\n",
#> list(list("maxSizeOfObjects"), list("The maximum allowed total size, in bytes, of all\n",
#> "objects to and from the parallel worker allows.\n",
#> "This can help to protect against unexpectedly large data transfers between\n",
#> "the parent process and the parallel workers - data that is often transferred\n",
#> "over the network, which sometimes also includes the internet. For instance,\n",
#> "if you sit at home and have set up a future backend with workers running\n",
#> "remotely at your university or company, then you might want to use this\n",
#> "protection to avoid transferring giga- or terabytes of data without noticing.\n",
#> "(Default: ", list(list("500 \\cdot 1024^2")),
#> " bytes = 500 MiB, unless overridden by a\n",
#> "FutureBackend subclass, or by R option ",
#> list("future.globals.maxSize"), " (sic!))")),
#> "\n", "\n", list(list("interrupts"), list("If FALSE, attempts to interrupt futures will not take\n",
#> "place on this backend, even if the backend supports it. This is useful\n",
#> "when, for instance, it takes a long time to interrupt a future.")),
#> "\n", "\n", list(list("backend"), list("a ",
#> list("FutureBackend"), ".")), "\n", "\n", list(
#> list("future"), list("a ", list("Future"),
#> " to be started.")), "\n", "\n", list(list(
#> "wait.timeout"), list("Number of seconds before timing out.")),
#> "\n", "\n", list(list("wait.interval"), list(
#> "Baseline number of seconds between retries.")),
#> "\n", "\n", list(list("wait.alpha"), list("Scale factor increasing waiting interval after each\n",
#> "attempt.")), "\n", "\n", list(list("workers"),
#> list("...")), "\n", "\n", list(list("persistent"),
#> list("(deprecated) ...")), "\n", "\n", list(
#> list(list()), list("(optional) not used.")),
#> "\n"), "\n", list("\n", list("FutureBackend()"),
#> " returns a FutureBackend object, which inherits an\n",
#> "environment. Specific future backends are defined by subclasses\n",
#> "implementing the FutureBackend API.\n", "\n",
#> list("launchFuture()"), " returns the launched ",
#> list("Future"), " object.\n", "\n", list("interruptFuture()"),
#> " returns the interrupted ", list("Future"),
#> " object,\n", "if supported, other the unmodified future.\n",
#> "\n", list("stopWorkers()"), " returns TRUE if the workers were shut down,\n",
#> "otherwise FALSE.\n"), "\n", list("\n", list(
#> "This functionality is only for developers who wish to implement their\n",
#> "own future backend. End-users and package developers use futureverse,\n",
#> "does not need to know about these functions."),
#> "\n", "\n", "If you are looking for available future backends to choose from, please\n",
#> "see the 'A Future for R: Available Future Backends' vignette and\n",
#> list("https://www.futureverse.org/backends.html"),
#> ".\n"), "\n", list("\n", "The ", list("ClusterFutureBackend"),
#> " is selected by\n", list("plan(cluster, workers = workers)"),
#> ".\n", "\n", "The ", list("MulticoreFutureBackend"),
#> " backend is selected by\n", list("plan(multicore, workers = workers)"),
#> ".\n", "\n", "The ", list("SequentialFutureBackend"),
#> " is selected by ", list("plan(sequential)"),
#> ".\n", "\n", "The ", list("MultisessionFutureBackend"),
#> " backend is selected by\n", list("plan(multisession, workers = workers)"),
#> ".\n"), "\n", list(list("The FutureBackend API"),
#> list("\n", "\n", "The ", list("FutureBackend"),
#> " class specifies FutureBackend API,\n", "that all backends must implement and comply to. Specifically,\n")),
#> "\n", "\n", list("\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n", "\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n"), "\n", list("internal"), "\n"), FutureCondition.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/protected_api-FutureCondition-class.R,",
#> "\n", "% R/protected_api-journal.R", "\n", list(
#> "FutureCondition"), "\n", list("FutureCondition"),
#> "\n", list("FutureMessage"), "\n", list("FutureWarning"),
#> "\n", list("FutureError"), "\n", list("RngFutureCondition"),
#> "\n", list("RngFutureWarning"), "\n", list("RngFutureError"),
#> "\n", list("UnexpectedFutureResultError"), "\n",
#> list("GlobalEnvMisuseFutureCondition"), "\n", list(
#> "GlobalEnvMisuseFutureWarning"), "\n", list("GlobalEnvMisuseFutureError"),
#> "\n", list("ConnectionMisuseFutureCondition"), "\n",
#> list("ConnectionMisuseFutureWarning"), "\n", list(
#> "ConnectionMisuseFutureError"), "\n", list("DeviceMisuseFutureCondition"),
#> "\n", list("DeviceMisuseFutureWarning"), "\n", list(
#> "DeviceMisuseFutureError"), "\n", list("DefaultDeviceMisuseFutureCondition"),
#> "\n", list("DefaultDeviceMisuseFutureWarning"), "\n",
#> list("DefaultDeviceMisuseFutureError"), "\n", list(
#> "FutureLaunchError"), "\n", list("FutureInterruptError"),
#> "\n", list("FutureCanceledError"), "\n", list("FutureDroppedError"),
#> "\n", list("FutureJournalCondition"), "\n", list(
#> "A condition (message, warning, or error) that occurred while orchestrating a future"),
#> "\n", list("\n", "FutureCondition(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "FutureMessage(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "FutureWarning(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "FutureError(message, call = NULL, uuid = future[[\"uuid\"]], future = NULL)\n",
#> "\n", "RngFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " uuid = future[[\"uuid\"]],\n",
#> " future = NULL\n", ")\n", "\n", "RngFutureWarning(...)\n",
#> "\n", "RngFutureError(...)\n", "\n", "UnexpectedFutureResultError(future, hint = NULL)\n",
#> "\n", "GlobalEnvMisuseFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " differences = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "GlobalEnvMisuseFutureWarning(...)\n",
#> "\n", "GlobalEnvMisuseFutureError(...)\n", "\n",
#> "ConnectionMisuseFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " differences = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "ConnectionMisuseFutureWarning(...)\n",
#> "\n", "ConnectionMisuseFutureError(...)\n", "\n",
#> "DeviceMisuseFutureCondition(\n", " message = NULL,\n",
#> " call = NULL,\n", " differences = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "DeviceMisuseFutureWarning(...)\n",
#> "\n", "DeviceMisuseFutureError(...)\n", "\n",
#> "DefaultDeviceMisuseFutureCondition(\n", " message = NULL,\n",
#> " incidents = NULL,\n", " call = NULL,\n",
#> " uuid = future[[\"uuid\"]],\n", " future = NULL\n",
#> ")\n", "\n", "DefaultDeviceMisuseFutureWarning(...)\n",
#> "\n", "DefaultDeviceMisuseFutureError(...)\n",
#> "\n", "FutureLaunchError(..., future = NULL)\n",
#> "\n", "FutureInterruptError(..., future = NULL)\n",
#> "\n", "FutureCanceledError(..., future = NULL)\n",
#> "\n", "FutureDroppedError(..., future = NULL)\n",
#> "\n", "FutureJournalCondition(\n", " message,\n",
#> " journal,\n", " call = NULL,\n", " uuid = future[[\"uuid\"]],\n",
#> " future = NULL\n", ")\n"), "\n", list("\n",
#> list(list("message"), list("A message condition.")),
#> "\n", "\n", list(list("call"), list("The call stack that led up to the condition.")),
#> "\n", "\n", list(list("uuid"), list("A universally unique identifier for the future associated with\n",
#> "this FutureCondition.")), "\n", "\n", list(
#> list("future"), list("The ", list("Future"),
#> " involved.")), "\n", "\n", list(list("hint"),
#> list("(optional) A string with a suggestion on what might be wrong.")),
#> "\n"), "\n", list("\n", "An object of class FutureCondition which inherits from class\n",
#> list("condition"), " and FutureMessage, FutureWarning,\n",
#> "and FutureError all inherits from FutureCondition.\n",
#> "Moreover, a FutureError inherits from ", list(
#> "error"), ",\n", "a FutureWarning from ", list(
#> "warning"), ", and\n", "a FutureMessage from ",
#> list("message"), ".\n"), "\n", list("\n", "While ",
#> list("orchestrating"), " (creating, launching, querying, collection)\n",
#> "futures, unexpected run-time errors (and other types of conditions) may\n",
#> "occur. Such conditions are coerced to a corresponding FutureCondition\n",
#> "class to help distinguish them from conditions that occur due to the\n",
#> list("evaluation"), " of the future.\n"), "\n",
#> list("internal"), "\n"), FutureGlobals.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/protected_api-FutureGlobals-class.R",
#> "\n", list("FutureGlobals"), "\n", list("FutureGlobals"),
#> "\n", list("as.FutureGlobals"), "\n", list("as.FutureGlobals.FutureGlobals"),
#> "\n", list("as.FutureGlobals.Globals"), "\n", list(
#> "as.FutureGlobals.list"), "\n", list("[.FutureGlobals"),
#> "\n", list("c.FutureGlobals"), "\n", list("unique.FutureGlobals"),
#> "\n", list("A representation of a set of globals used with futures"),
#> "\n", list("\n", "FutureGlobals(object = list(), resolved = FALSE, total_size = NA_real_, ...)\n"),
#> "\n", list("\n", list(list("object"), list("A named list.")),
#> "\n", "\n", list(list("resolved"), list("A logical indicating whether these globals\n",
#> "have been scanned for and resolved futures or not.")),
#> "\n", "\n", list(list("total_size"), list("The total size of all globals, if known.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "An object of class ",
#> list("FutureGlobals"), ".\n"), "\n", list("\n",
#> "A representation of a set of globals used with futures\n"),
#> "\n", list("\n", "This class extends the ", list(
#> "Globals"), " class by adding\n", "attributes ",
#> list("resolved"), " and ", list("total_size"),
#> ".\n"), "\n", list("internal"), "\n"), FutureResult.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/protected_api-FutureResult-class.R",
#> "\n", list("FutureResult"), "\n", list("FutureResult"),
#> "\n", list("as.character.FutureResult"), "\n", list(
#> "print.FutureResult"), "\n", list("Results from resolving a future"),
#> "\n", list("\n", "FutureResult(\n", " value = NULL,\n",
#> " visible = TRUE,\n", " stdout = NULL,\n",
#> " conditions = NULL,\n", " rng = FALSE,\n",
#> " ...,\n", " uuid = NULL,\n", " started = .POSIXct(NA_real_),\n",
#> " finished = Sys.time(),\n", " version = \"1.8\"\n",
#> ")\n", "\n", list(list("as.character"), list(
#> "FutureResult")), "(x, ...)\n", "\n", list(
#> list("print"), list("FutureResult")), "(x, ...)\n"),
#> "\n", list("\n", list(list("value"), list("The value of the future expression.\n",
#> "If the expression was not fully resolved (e.g. an error) occurred,\n",
#> "the the value is ", list("NULL"), ".")), "\n",
#> "\n", list(list("visible"), list("If TRUE, the value was visible, otherwise invisible.")),
#> "\n", "\n", list(list("conditions"), list("A list of zero or more list elements each containing\n",
#> "a captured ", list("condition"), " and possibly more meta data such as the\n",
#> "call stack and a timestamp.")), "\n", "\n",
#> list(list("rng"), list("If TRUE, the ", list(
#> ".Random.seed"), " was updated from resolving the\n",
#> "future, otherwise not.")), "\n", "\n", list(
#> list("started, finished"), list(list("POSIXct"),
#> " timestamps\n", "when the evaluation of the future expression was started and finished.")),
#> "\n", "\n", list(list("version"), list("The version format of the results.")),
#> "\n", "\n", list(list(list()), list("(optional) Additional named results to be returned.")),
#> "\n"), "\n", list("\n", "An object of class FutureResult.\n"),
#> "\n", list("\n", "Results from resolving a future\n"),
#> "\n", list("\n", "This function is only part of the ",
#> list("backend"), " Future API.\n", "This function is ",
#> list("not"), " part of the frontend Future API.\n"),
#> "\n", list(list("Note to developers"), list("\n",
#> "\n", "The FutureResult structure is ", list(
#> "under development"), " and may change at anytime,\n",
#> "e.g. elements may be renamed or removed. Because of this, please avoid\n",
#> "accessing the elements directly in code. Feel free to reach out if you need\n",
#> "to do so in your code.\n")), "\n", "\n", list(
#> "internal"), "\n"), `MulticoreFuture-class.Rd` = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("MulticoreFuture-class"), "\n", list("MulticoreFuture-class"),
#> "\n", list("resolved.MulticoreFuture"), "\n", list(
#> "A multicore future is a future whose value will be resolved asynchronously in a parallel process"),
#> "\n", list("\n", list(list("resolved"), list("MulticoreFuture")),
#> "(x, run = TRUE, timeout = NULL, ...)\n"), "\n",
#> list("\n", list("MulticoreFuture()"), " returns an object of class ",
#> list("MulticoreFuture"), ".\n"), "\n", list("\n",
#> "A multicore future is a future whose value will be resolved asynchronously in a parallel process\n"),
#> "\n", list(list("Usage"), list("\n", "\n", "To use 'multicore' futures, use ",
#> list("plan(multicore, ...)"), ", cf. ", list(
#> "multicore"), ".\n")), "\n", "\n", list("internal"),
#> "\n"), `MultiprocessFuture-class.Rd` = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in", "\n", "% R/backend_api-03.MultiprocessFutureBackend-class.R",
#> "\n", list("MultiprocessFuture-class"), "\n", list(
#> "MultiprocessFuture-class"), "\n", list("MultiprocessFuture"),
#> "\n", list("A multiprocess future is a future whose value will be resolved asynchronously in a parallel process"),
#> "\n", list("\n", "MultiprocessFuture(expr = NULL, substitute = TRUE, envir = parent.frame(), ...)\n"),
#> "\n", list("\n", list(list("expr"), list("An ", list(),
#> " ", list("expression"), ".")), "\n", "\n", list(
#> list("substitute"), list("If TRUE, argument ",
#> list("expr"), " is\n", list(list("substitute"),
#> "()"), ":ed, otherwise not.")), "\n", "\n",
#> list(list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n", "identified.")),
#> "\n", "\n", list(list(list()), list("Additional named elements passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("MultiprocessFuture()"), " returns an object of class ",
#> list("MultiprocessFuture"), ".\n"), "\n", list(
#> "\n", "A multiprocess future is a future whose value will be resolved asynchronously in a parallel process\n"),
#> "\n", list("internal"), "\n"), `UniprocessFuture-class.Rd` = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/backend_api-UniprocessFuture-class.R",
#> "\n", list("UniprocessFuture-class"), "\n", list(
#> "UniprocessFuture-class"), "\n", list("UniprocessFuture"),
#> "\n", list("An uniprocess future is a future whose value will be resolved synchronously in the current process"),
#> "\n", list("\n", "UniprocessFuture(expr = NULL, substitute = TRUE, envir = parent.frame(), ...)\n"),
#> "\n", list("\n", list(list("expr"), list("An ", list(),
#> " ", list("expression"), ".")), "\n", "\n", list(
#> list("substitute"), list("If TRUE, argument ",
#> list("expr"), " is\n", list(list("substitute"),
#> "()"), ":ed, otherwise not.")), "\n", "\n",
#> list(list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n", "identified.")),
#> "\n", "\n", list(list(list()), list("Additional named elements passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("UniprocessFuture()"), " returns an object of class ",
#> list("UniprocessFuture"), ".\n"), "\n", list(
#> "\n", "An uniprocess future is a future whose value will be resolved synchronously in the current process\n"),
#> "\n", list("internal"), "\n"), backtrace.Rd = list(
#> "% Generated by roxygen2: do not edit by hand", "\n",
#> "% Please edit documentation in R/utils_api-backtrace.R",
#> "\n", list("backtrace"), "\n", list("backtrace"),
#> "\n", list("Back trace the expressions evaluated when an error was caught"),
#> "\n", list("\n", "backtrace(future, envir = parent.frame(), ...)\n"),
#> "\n", list("\n", list(list("future"), list("A future with a caught error.")),
#> "\n", "\n", list(list("envir"), list("the environment where to locate the future.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A list with the future's call stack that led up to the error.\n"),
#> "\n", list("\n", "Back trace the expressions evaluated when an error was caught\n"),
#> "\n", list("\n", "my_log <- function(x) log(x)\n",
#> "foo <- function(...) my_log(...)\n", "\n", "f <- future({ foo(\"a\") })\n",
#> "res <- tryCatch({\n", " v <- value(f)\n", "}, error = function(ex) {\n",
#> " t <- backtrace(f)\n", " print(t)\n", "})\n",
#> list("\n", "## R CMD check: make sure any open connections are closed afterward\n",
#> "plan(sequential)\n"), "\n", "\n"), "\n"),
#> cancel.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-cancel.R",
#> "\n", list("cancel"), "\n", list("cancel"), "\n",
#> list("Cancel a future"), "\n", list("\n", "cancel(x, interrupt = TRUE, ...)\n"),
#> "\n", list("\n", list(list("x"), list("A Future.")),
#> "\n", "\n", list(list("interrupt"), list("If TRUE, running futures are interrupted, if the\n",
#> "future backend supports it.")), "\n", "\n",
#> list(list(list()), list("All arguments used by the S3 methods.")),
#> "\n"), "\n", list("\n", list("cancel()"), " returns (invisibly) the canceled ",
#> list("Future"), "s after\n", "flagging them as \"canceled\" and possibly interrupting them as well.\n",
#> "\n", "Canceling a lazy or a finished future has no effect.\n"),
#> "\n", list("\n", "Cancels futures, with the option to interrupt running ones.\n"),
#> "\n", list("\n", list("if ((interactive() || .Platform[[\"OS.type\"]] != \"windows\")) (if (getRversion() >= \"3.4\") withAutoprint else force)({ # examplesIf"),
#> "\n", "## Set up two parallel workers\n", "plan(multisession, workers = 2)\n",
#> "\n", "## Launch two long running future\n",
#> "fs <- lapply(c(1, 2), function(duration) {\n",
#> " future({\n", " Sys.sleep(duration)\n",
#> " 42\n", " })\n", "})\n", "\n", "## Wait until at least one of the futures is resolved\n",
#> "while (!any(resolved(fs))) Sys.sleep(0.1)\n",
#> "\n", "## Cancel the future that is not yet resolved\n",
#> "r <- resolved(fs)\n", "cancel(fs[!r])\n",
#> "\n", "## Get the value of the resolved future\n",
#> "f <- fs[r]\n", "v <- value(f)\n", "message(\"Result: \", v)\n",
#> "\n", "## The value of the canceled future is an error\n",
#> "try(v <- value(fs[!r]))\n", "\n", "## Shut down parallel workers\n",
#> "plan(sequential)\n", list("}) # examplesIf"),
#> "\n"), "\n", list("\n", "A canceled future can be ",
#> list(list("reset()")), " to a lazy, vanilla future\n",
#> "such that it can be relaunched, possible on another future backend.\n"),
#> "\n"), cluster.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.ClusterFutureBackend-class.R",
#> "\n", list("cluster"), "\n", list("cluster"),
#> "\n", list("Create a cluster future whose value will be resolved asynchronously in a parallel process"),
#> "\n", list("\n", "cluster(\n", " ...,\n", " workers = availableWorkers(constraints = \"connections\"),\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " persistent = FALSE,\n", " envir = parent.frame()\n",
#> ")\n"), "\n", list("\n", list(list("workers"),
#> list("A ", list(list("cluster")), " object,\n",
#> "a character vector of host names, a positive numeric scalar,\n",
#> "or a function.\n", "If a character vector or a numeric scalar, a ",
#> list("cluster"), " object\n", "is created using ",
#> list(list("makeClusterPSOCK"), "(workers)"),
#> ".\n", "If a function, it is called without arguments ",
#> list("when the future\n", "is created"),
#> " and its value is used to configure the workers.\n",
#> "The function should return any of the above types.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("persistent"), list("If FALSE, the evaluation environment is cleared\n",
#> "from objects prior to the evaluation of the future.")),
#> "\n", "\n", list(list("envir"), list("The ",
#> list("environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(list()),
#> list("Additional named elements passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A ClusterFuture.\n"), "\n", list(
#> "\n", list("WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A cluster future is a future that uses cluster evaluation,\n",
#> "which means that its ", list("value is computed and resolved in\n",
#> "parallel in another process"), ".\n", "\n",
#> "This function is must ", list("not"), " be called directly. Instead, the\n",
#> "typical usages are:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures via a single background R process on the local machine\n",
#> "plan(cluster, workers = I(1))\n", "\n",
#> "# Evaluate futures via two background R processes on the local machine\n",
#> "plan(cluster, workers = 2)\n", "\n", "# Evaluate futures via a single R process on another machine on on the\n",
#> "# local area network (LAN)\n", "plan(cluster, workers = \"raspberry-pi\")\n",
#> "\n", "# Evaluate futures via a single R process running on a remote machine\n",
#> "plan(cluster, workers = \"pi.example.org\")\n",
#> "\n", "# Evaluate futures via four R processes, one running on the local machine,\n",
#> "# two running on LAN machine 'n1' and one on a remote machine\n",
#> "plan(cluster, workers = c(\"localhost\", \"n1\", \"n1\", \"pi.example.org\"))\n"),
#> list(list("html"), list(list("</div>"))), "\n"),
#> "\n", list("\n", list("\n", "\n", "## Use cluster futures\n",
#> "cl <- parallel::makeCluster(2, timeout = 60)\n",
#> "plan(cluster, workers = cl)\n", "\n", "## A global variable\n",
#> "a <- 0\n", "\n", "## Create future (explicitly)\n",
#> "f <- future({\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "\n", "## A cluster future is evaluated in a separate process.\n",
#> "## Regardless, changing the value of a global variable will\n",
#> "## not affect the result of the future.\n",
#> "a <- 7\n", "print(a)\n", "\n", "v <- value(f)\n",
#> "print(v)\n", "stopifnot(v == 0)\n", "\n",
#> "## CLEANUP\n", "parallel::stopCluster(cl)\n",
#> "\n"), "\n"), "\n", list("\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n"), "\n"), clusterExportSticky.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-sticky_globals.R",
#> "\n", list("clusterExportSticky"), "\n", list(
#> "clusterExportSticky"), "\n", list("Export globals to the sticky-globals environment of the cluster nodes"),
#> "\n", list("\n", "clusterExportSticky(cl, globals)\n"),
#> "\n", list("\n", list(list("cl"), list("(cluster) A cluster object as returned by\n",
#> list(list("parallel::makeCluster()")), ".")),
#> "\n", "\n", list(list("globals"), list("(list) A named list of sticky globals to be exported.")),
#> "\n"), "\n", list("\n", "(invisible; cluster) The cluster object.\n"),
#> "\n", list("\n", "Export globals to the sticky-globals environment of the cluster nodes\n"),
#> "\n", list("\n", "This requires that the ", list(
#> "future"), " package is installed on the cluster\n",
#> "nodes.\n"), "\n", list("internal"), "\n"),
#> find_references.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-marshalling.R",
#> "\n", list("find_references"), "\n", list("find_references"),
#> "\n", list("assert_no_references"), "\n", list(
#> "Get the first or all references of an ", list(),
#> " object"), "\n", list("\n", "find_references(x, first_only = FALSE)\n",
#> "\n", "assert_no_references(\n", " x,\n",
#> " action = c(\"error\", \"warning\", \"message\", \"string\"),\n",
#> " source = c(\"globals\", \"value\")\n", ")\n"),
#> "\n", list("\n", list(list("x"), list("The ",
#> list(), " object to be checked.")), "\n", "\n",
#> list(list("first_only"), list("If ", list("TRUE"),
#> ", only the first reference is returned,\n",
#> "otherwise all references.")), "\n", "\n",
#> list(list("action"), list("Type of action to take if a reference is found.")),
#> "\n", "\n", list(list("source"), list("Is the source of ",
#> list("x"), " the globals or the value of the future?")),
#> "\n"), "\n", list("\n", list("find_references()"),
#> " returns a list of zero or more references\n",
#> "identified.\n", "\n", "If a reference is detected, an informative error, warning, message,\n",
#> "or a character string is produced, otherwise ",
#> list("NULL"), " is returned\n", "invisibly.\n"),
#> "\n", list("\n", "Get the first or all references of an ",
#> list(), " object\n", "\n", "Assert that there are no references among the identified globals\n"),
#> "\n", list("internal"), "\n"), future.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-future.R, R/utils_api-futureCall.R,",
#> "\n", "% R/utils_api-minifuture.R", "\n", list(
#> "future"), "\n", list("future"), "\n", list(
#> "futureCall"), "\n", list("minifuture"), "\n",
#> list("Create a future"), "\n", list("\n", "future(\n",
#> " expr,\n", " envir = parent.frame(),\n",
#> " substitute = TRUE,\n", " lazy = FALSE,\n",
#> " seed = FALSE,\n", " globals = TRUE,\n",
#> " packages = NULL,\n", " stdout = TRUE,\n",
#> " conditions = \"condition\",\n", " label = NULL,\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " ...\n", ")\n", "\n", "futureCall(\n", " FUN,\n",
#> " args = list(),\n", " envir = parent.frame(),\n",
#> " lazy = FALSE,\n", " seed = FALSE,\n", " globals = TRUE,\n",
#> " packages = NULL,\n", " stdout = TRUE,\n",
#> " conditions = \"condition\",\n", " earlySignal = FALSE,\n",
#> " label = NULL,\n", " gc = FALSE,\n", " ...\n",
#> ")\n", "\n", "minifuture(\n", " expr,\n",
#> " substitute = TRUE,\n", " globals = NULL,\n",
#> " packages = NULL,\n", " stdout = NA,\n",
#> " conditions = NULL,\n", " seed = NULL,\n",
#> " ...,\n", " envir = parent.frame()\n", ")\n"),
#> "\n", list("\n", list(list("expr"), list("An ",
#> list(), " ", list("expression"), ".")), "\n",
#> "\n", list(list("envir"), list("The ", list(
#> "environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list("substitute"),
#> list("If TRUE, argument ", list("expr"),
#> " is\n", list(list("substitute"), "()"),
#> ":ed, otherwise not.")), "\n", "\n", list(
#> list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("seed"), list("(optional) If TRUE, the random seed, that is, the state of the\n",
#> "random number generator (RNG) will be set such that statistically sound\n",
#> "random numbers are produced (also during parallelization).\n",
#> "If FALSE (default), it is assumed that the future expression does neither\n",
#> "need nor use random numbers generation.\n",
#> "To use a fixed random seed, specify a L'Ecuyer-CMRG seed (seven integer)\n",
#> "or a regular RNG seed (a single integer). If the latter, then a\n",
#> "L'Ecuyer-CMRG seed will be automatically created based on the given seed.\n",
#> "Furthermore, if FALSE, then the future will be monitored to make sure it\n",
#> "does not use random numbers. If it does and depending on the value of\n",
#> "option ", list("future.rng.onMisuse"), ", the check is\n",
#> "ignored, an informative warning, or error will be produced.\n",
#> "If ", list("seed"), " is NULL, then the effect is as with ",
#> list("seed = FALSE"), "\n", "but without the RNG check being performed.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, or a named list\n",
#> "to control how globals are handled.\n",
#> "For details, see section 'Globals used by future expressions'\n",
#> "in the help for ", list(list("future()")),
#> ".")), "\n", "\n", list(list("packages"),
#> list("(optional) a character vector specifying packages\n",
#> "to be attached in the ", list(), " environment evaluating the future.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE (default), then the standard output is captured,\n",
#> "and re-outputted when ", list("value()"),
#> " is called.\n", "If FALSE, any output is silenced (by sinking it to the null device as\n",
#> "it is outputted).\n", "Using ", list("stdout = structure(TRUE, drop = TRUE)"),
#> " causes the captured\n", "standard output to be dropped from the future object as soon as it has\n",
#> "been relayed. This can help decrease the overall memory consumed by\n",
#> "captured output across futures.\n", "Using ",
#> list("stdout = NA"), " fully avoids intercepting the standard output;\n",
#> "behavior of such unhandled standard output depends on the future backend.")),
#> "\n", "\n", list(list("conditions"), list("A character string of conditions classes to be captured\n",
#> "and relayed. The default is to relay all conditions, including messages\n",
#> "and warnings. To drop all conditions, use ",
#> list("conditions = character(0)"), ".\n",
#> "Errors are always relayed.\n", "Attribute ",
#> list("exclude"), " can be used to ignore specific classes, e.g.\n",
#> list("conditions = structure(\"condition\", exclude = \"message\")"),
#> " will capture\n", "all ", list("condition"),
#> " classes except those that inherits from the ",
#> list("message"), " class.\n", "Using ", list(
#> "conditions = structure(..., drop = TRUE)"),
#> " causes any captured\n", "conditions to be dropped from the future object as soon as it has\n",
#> "been relayed, e.g. by ", list("value(f)"),
#> ". This can help decrease the overall\n",
#> "memory consumed by captured conditions across futures.\n",
#> "Using ", list("conditions = NULL"), " (not recommended) avoids intercepting conditions,\n",
#> "except from errors; behavior of such unhandled conditions depends on the\n",
#> "future backend and the environment from which R runs.")),
#> "\n", "\n", list(list("label"), list("A character string label attached to the future.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("FUN"), list("A ", list("function"),
#> " to be evaluated.")), "\n", "\n", list(
#> list("args"), list("A ", list("list"), " of arguments passed to function ",
#> list("FUN"), ".")), "\n", "\n", list(list(
#> list()), list("Additional arguments passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("future()"), " returns ", list(
#> "Future"), " that evaluates expression ", list(
#> "expr"), ".\n", "\n", list("futureCall()"),
#> " returns a ", list("Future"), " that calls function ",
#> list("FUN"), " with\n", "arguments ", list(
#> "args"), ".\n", "\n", list("minifuture(expr)"),
#> " creates a future with minimal overhead, by disabling\n",
#> "user-friendly behaviors, e.g. automatic identification of global\n",
#> "variables and packages needed, and relaying of output. Unless you have\n",
#> "good reasons for using this function, please use ",
#> list(list("future()")), " instead.\n", "This function exists mainly for the purpose of profiling and identifying\n",
#> "which automatic features of ", list(list("future()")),
#> " introduce extra overhead.\n"), "\n", list(
#> "\n", list(list("html"), list(list(list("logo.png"),
#> list("options: style='float: right;' alt='logo' width='120'")))),
#> "\n", "Creates a future that evaluates an ",
#> list(), " expression or\n", "a future that calls an ",
#> list(), " function with a set of arguments.\n",
#> "How, when, and where these futures are evaluated can be configured\n",
#> "using ", list(list("plan()")), " such that it is evaluated in parallel on,\n",
#> "for instance, the current machine, on a remote machine, or via a\n",
#> "job queue on a compute cluster.\n", "Importantly, any ",
#> list(), " code using futures remains the same regardless\n",
#> "on these settings and there is no need to modify the code when\n",
#> "switching from, say, sequential to parallel processing.\n"),
#> "\n", list("\n", "The state of a future is either unresolved or resolved.\n",
#> "The value of a future can be retrieved using ",
#> list("v <- ", list("value"), "(f)"), ".\n",
#> "Querying the value of a non-resolved future will ",
#> list("block"), " the call\n", "until the future is resolved.\n",
#> "It is possible to check whether a future is resolved or not\n",
#> "without blocking by using ", list(list("resolved"),
#> "(f)"), ".\n", "It is possible to ", list(
#> list("cancel()")), " a future that is being resolved.\n",
#> "Failed, canceled, and interrupted futures can be ",
#> list(list("reset()")), " to a\n", "lazy, vanilla future that can be relaunched.\n",
#> "\n", "The ", list("futureCall()"), " function works analogously to\n",
#> list(list("do.call"), "()"), ", which calls a function with a set of\n",
#> "arguments. The difference is that ", list(
#> "do.call()"), " returns the value of\n",
#> "the call whereas ", list("futureCall()"),
#> " returns a future.\n"), "\n", list(list("Eager or lazy evaluation"),
#> list("\n", "\n", "By default, a future is resolved using ",
#> list("eager"), " evaluation\n", "(", list(
#> "lazy = FALSE"), "). This means that the expression starts to\n",
#> "be evaluated as soon as the future is created.\n",
#> "\n", "As an alternative, the future can be resolved using ",
#> list("lazy"), "\n", "evaluation (", list(
#> "lazy = TRUE"), "). This means that the expression\n",
#> "will only be evaluated when the value of the future is requested.\n",
#> list("Note that this means that the expression may not be evaluated\n",
#> "at all - it is guaranteed to be evaluated if the value is requested"),
#> ".\n")), "\n", "\n", list(list("Globals used by future expressions"),
#> list("\n", "\n", "Global objects (short ",
#> list("globals"), ") are objects (e.g. variables and\n",
#> "functions) that are needed in order for the future expression to be\n",
#> "evaluated while not being local objects that are defined by the future\n",
#> "expression. For example, in\n", list("\n",
#> " a <- 42\n", " f <- future({ b <- 2; a * b })\n"),
#> "\n", "variable ", list("a"), " is a global of future assignment ",
#> list("f"), " whereas\n", list("b"), " is a local variable.\n",
#> "In order for the future to be resolved successfully (and correctly),\n",
#> "all globals need to be gathered when the future is created such that\n",
#> "they are available whenever and wherever the future is resolved.\n",
#> "\n", "The default behavior (", list("globals = TRUE"),
#> "),\n", "is that globals are automatically identified and gathered.\n",
#> "More precisely, globals are identified via code inspection of the\n",
#> "future expression ", list("expr"), " and their values are retrieved with\n",
#> "environment ", list("envir"), " as the starting point (basically via\n",
#> list("get(global, envir = envir, inherits = TRUE)"),
#> ").\n", list("In most cases, such automatic collection of globals is sufficient\n",
#> "and less tedious and error prone than if they are manually specified"),
#> ".\n", "\n", "However, for full control, it is also possible to explicitly specify\n",
#> "exactly which the globals are by providing their names as a character\n",
#> "vector.\n", "In the above example, we could use\n",
#> list("\n", " a <- 42\n", " f <- future({ b <- 2; a * b }, globals = \"a\")\n"),
#> "\n", "\n", "Yet another alternative is to explicitly specify also their values\n",
#> "using a named list as in\n", list("\n",
#> " a <- 42\n", " f <- future({ b <- 2; a * b }, globals = list(a = a))\n"),
#> "\n", "or\n", list("\n", " f <- future({ b <- 2; a * b }, globals = list(a = 42))\n"),
#> "\n", "\n", "Specifying globals explicitly avoids the overhead added from\n",
#> "automatically identifying the globals and gathering their values.\n",
#> "Furthermore, if we know that the future expression does not make use\n",
#> "of any global variables, we can disable the automatic search for\n",
#> "globals by using\n", list("\n", " f <- future({ a <- 42; b <- 2; a * b }, globals = FALSE)\n"),
#> "\n", "\n", "Future expressions often make use of functions from one or more packages.\n",
#> "As long as these functions are part of the set of globals, the future\n",
#> "package will make sure that those packages are attached when the future\n",
#> "is resolved. Because there is no need for such globals to be frozen\n",
#> "or exported, the future package will not export them, which reduces\n",
#> "the amount of transferred objects.\n", "For example, in\n",
#> list("\n", " x <- rnorm(1000)\n", " f <- future({ median(x) })\n"),
#> "\n", "variable ", list("x"), " and ", list(
#> "median()"), " are globals, but only ",
#> list("x"), "\n", "is exported whereas ",
#> list("median()"), ", which is part of the ",
#> list("stats"), "\n", "package, is not exported. Instead it is made sure that the ",
#> list("stats"), "\n", "package is on the search path when the future expression is evaluated.\n",
#> "Effectively, the above becomes\n", list(
#> "\n", " x <- rnorm(1000)\n", " f <- future({\n",
#> " library(stats)\n", " median(x)\n",
#> " })\n"), "\n", "To manually specify this, one can either do\n",
#> list("\n", " x <- rnorm(1000)\n", " f <- future({\n",
#> " median(x)\n", " }, globals = list(x = x, median = stats::median)\n"),
#> "\n", "or\n", list("\n", " x <- rnorm(1000)\n",
#> " f <- future({\n", " library(stats)\n",
#> " median(x)\n", " }, globals = list(x = x))\n"),
#> "\n", "Both are effectively the same.\n",
#> "\n", "Although rarely needed, a combination of automatic identification and manual\n",
#> "specification of globals is supported via attributes ",
#> list("add"), " (to add\n", "false negatives) and ",
#> list("ignore"), " (to ignore false positives) on value\n",
#> list("TRUE"), ". For example, with\n", list(
#> "globals = structure(TRUE, ignore = \"b\", add = \"a\")"),
#> " any globals\n", "automatically identified, except ",
#> list("b"), ", will be used, in addition to\n",
#> "global ", list("a"), ".\n")), "\n", "\n",
#> list("\n", "## Evaluate futures in parallel\n",
#> "plan(multisession)\n", "\n", "## Data\n",
#> "x <- rnorm(100)\n", "y <- 2 * x + 0.2 + rnorm(100)\n",
#> "w <- 1 + x ^ 2\n", "\n", "\n", "## EXAMPLE: Regular assignments (evaluated sequentially)\n",
#> "fitA <- lm(y ~ x, weights = w) ## with offset\n",
#> "fitB <- lm(y ~ x - 1, weights = w) ## without offset\n",
#> "fitC <- {\n", " w <- 1 + abs(x) ## Different weights\n",
#> " lm(y ~ x, weights = w)\n", "}\n", "print(fitA)\n",
#> "print(fitB)\n", "print(fitC)\n", "\n", "\n",
#> "## EXAMPLE: Future assignments (evaluated in parallel)\n",
#> "fitA %<-% lm(y ~ x, weights = w) ## with offset\n",
#> "fitB %<-% lm(y ~ x - 1, weights = w) ## without offset\n",
#> "fitC %<-% {\n", " w <- 1 + abs(x)\n", " lm(y ~ x, weights = w)\n",
#> "}\n", "print(fitA)\n", "print(fitB)\n", "print(fitC)\n",
#> "\n", "\n", "## EXAMPLE: Explicitly create futures (evaluated in parallel)\n",
#> "## and retrieve their values\n", "fA <- future( lm(y ~ x, weights = w) )\n",
#> "fB <- future( lm(y ~ x - 1, weights = w) )\n",
#> "fC <- future({\n", " w <- 1 + abs(x)\n",
#> " lm(y ~ x, weights = w)\n", "})\n", "fitA <- value(fA)\n",
#> "fitB <- value(fB)\n", "fitC <- value(fC)\n",
#> "print(fitA)\n", "print(fitB)\n", "print(fitC)\n",
#> "\n", list("\n", "## Make sure to \"close\" an multisession workers on Windows\n",
#> "plan(sequential)\n"), "\n", "## EXAMPLE: futureCall() and do.call()\n",
#> "x <- 1:100\n", "y0 <- do.call(sum, args = list(x))\n",
#> "print(y0)\n", "\n", "f1 <- futureCall(sum, args = list(x))\n",
#> "y1 <- value(f1)\n", "print(y1)\n"), "\n",
#> list("\n", "How, when and where futures are resolved is given by the\n",
#> list("future backend"), ", which can be set by the end user using the\n",
#> list(list("plan()")), " function.\n"), "\n",
#> list("\n", "The future logo was designed by Dan LaBar and tweaked by Henrik Bengtsson.\n"),
#> "\n"), futureAssign.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/delayed_api-futureAssign.R,",
#> "\n", "% R/infix_api-01-futureAssign_OP.R, R/infix_api-02-globals_OP.R,",
#> "\n", "% R/infix_api-03-seed_OP.R, R/infix_api-04-stdout_OP.R,",
#> "\n", "% R/infix_api-05-conditions_OP.R, R/infix_api-06-lazy_OP.R,",
#> "\n", "% R/infix_api-07-label_OP.R, R/infix_api-08-plan_OP.R,",
#> "\n", "% R/infix_api-09-tweak_OP.R", "\n",
#> list("futureAssign"), "\n", list("futureAssign"),
#> "\n", list("%<-%"), "\n", list("%->%"), "\n",
#> list("%globals%"), "\n", list("%packages%"),
#> "\n", list("%seed%"), "\n", list("%stdout%"),
#> "\n", list("%conditions%"), "\n", list("%lazy%"),
#> "\n", list("%label%"), "\n", list("%plan%"),
#> "\n", list("%tweak%"), "\n", list("Create a future assignment"),
#> "\n", list("\n", "futureAssign(\n", " x,\n",
#> " value,\n", " envir = parent.frame(),\n",
#> " substitute = TRUE,\n", " lazy = FALSE,\n",
#> " seed = FALSE,\n", " globals = TRUE,\n",
#> " packages = NULL,\n", " stdout = TRUE,\n",
#> " conditions = \"condition\",\n", " earlySignal = FALSE,\n",
#> " label = NULL,\n", " gc = FALSE,\n", " ...,\n",
#> " assign.env = envir\n", ")\n", "\n", "x %<-% value\n",
#> "\n", "fassignment %globals% globals\n", "fassignment %packages% packages\n",
#> "\n", "fassignment %seed% seed\n", "\n", "fassignment %stdout% capture\n",
#> "\n", "fassignment %conditions% capture\n",
#> "\n", "fassignment %lazy% lazy\n", "\n", "fassignment %label% label\n",
#> "\n", "fassignment %plan% strategy\n", "\n",
#> "fassignment %tweak% tweaks\n"), "\n", list(
#> "\n", list(list("x"), list("the name of a future variable, which will hold the value\n",
#> "of the future expression (as a promise).")),
#> "\n", "\n", list(list("value"), list("An ",
#> list(), " ", list("expression"), ".")), "\n",
#> "\n", list(list("envir"), list("The ", list(
#> "environment"), " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list("substitute"),
#> list("If TRUE, argument ", list("expr"),
#> " is\n", list(list("substitute"), "()"),
#> ":ed, otherwise not.")), "\n", "\n", list(
#> list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("seed"), list("(optional) If TRUE, the random seed, that is, the state of the\n",
#> "random number generator (RNG) will be set such that statistically sound\n",
#> "random numbers are produced (also during parallelization).\n",
#> "If FALSE (default), it is assumed that the future expression does neither\n",
#> "need nor use random numbers generation.\n",
#> "To use a fixed random seed, specify a L'Ecuyer-CMRG seed (seven integer)\n",
#> "or a regular RNG seed (a single integer). If the latter, then a\n",
#> "L'Ecuyer-CMRG seed will be automatically created based on the given seed.\n",
#> "Furthermore, if FALSE, then the future will be monitored to make sure it\n",
#> "does not use random numbers. If it does and depending on the value of\n",
#> "option ", list("future.rng.onMisuse"), ", the check is\n",
#> "ignored, an informative warning, or error will be produced.\n",
#> "If ", list("seed"), " is NULL, then the effect is as with ",
#> list("seed = FALSE"), "\n", "but without the RNG check being performed.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, or a named list\n",
#> "to control how globals are handled.\n",
#> "For details, see section 'Globals used by future expressions'\n",
#> "in the help for ", list(list("future()")),
#> ".")), "\n", "\n", list(list("packages"),
#> list("(optional) a character vector specifying packages\n",
#> "to be attached in the ", list(), " environment evaluating the future.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE (default), then the standard output is captured,\n",
#> "and re-outputted when ", list("value()"),
#> " is called.\n", "If FALSE, any output is silenced (by sinking it to the null device as\n",
#> "it is outputted).\n", "Using ", list("stdout = structure(TRUE, drop = TRUE)"),
#> " causes the captured\n", "standard output to be dropped from the future object as soon as it has\n",
#> "been relayed. This can help decrease the overall memory consumed by\n",
#> "captured output across futures.\n", "Using ",
#> list("stdout = NA"), " fully avoids intercepting the standard output;\n",
#> "behavior of such unhandled standard output depends on the future backend.")),
#> "\n", "\n", list(list("conditions"), list("A character string of conditions classes to be captured\n",
#> "and relayed. The default is to relay all conditions, including messages\n",
#> "and warnings. To drop all conditions, use ",
#> list("conditions = character(0)"), ".\n",
#> "Errors are always relayed.\n", "Attribute ",
#> list("exclude"), " can be used to ignore specific classes, e.g.\n",
#> list("conditions = structure(\"condition\", exclude = \"message\")"),
#> " will capture\n", "all ", list("condition"),
#> " classes except those that inherits from the ",
#> list("message"), " class.\n", "Using ", list(
#> "conditions = structure(..., drop = TRUE)"),
#> " causes any captured\n", "conditions to be dropped from the future object as soon as it has\n",
#> "been relayed, e.g. by ", list("value(f)"),
#> ". This can help decrease the overall\n",
#> "memory consumed by captured conditions across futures.\n",
#> "Using ", list("conditions = NULL"), " (not recommended) avoids intercepting conditions,\n",
#> "except from errors; behavior of such unhandled conditions depends on the\n",
#> "future backend and the environment from which R runs.")),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("label"), list("A character string label attached to the future.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("assign.env"), list("The ",
#> list("environment"), " to which the variable\n",
#> "should be assigned.")), "\n", "\n", list(
#> list("fassignment"), list("The future assignment, e.g.\n",
#> list("x %<-% { expr }"), ".")), "\n", "\n",
#> list(list("capture"), list("If TRUE, the standard output will be captured, otherwise not.")),
#> "\n", "\n", list(list("strategy"), list("The backend controlling how the future is\n",
#> "resolved. See ", list(list("plan()")), " for further details.")),
#> "\n", "\n", list(list("tweaks"), list("A named list (or vector) with arguments that\n",
#> "should be changed relative to the current backend.")),
#> "\n", "\n", list(list(list()), list("Additional arguments passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", list("futureAssign()"), " and ", list(
#> "x %<-% expr"), " returns the ", list("Future"),
#> " invisibly,\n", "e.g. ", list("f <- futureAssign(\"x\", expr)"),
#> " and ", list("f <- (x %<-% expr)"), ".\n"),
#> "\n", list("\n", list("x %<-% value"), " (also known as a \"future assignment\") and\n",
#> list("futureAssign(\"x\", value)"), " create a ",
#> list("Future"), " that evaluates the expression\n",
#> "(", list("value"), ") and binds it to variable ",
#> list("x"), " (as a\n", list("promise"), "). The expression is evaluated in parallel\n",
#> "in the background. Later on, when ", list(
#> "x"), " is first queried, the value of future\n",
#> "is automatically retrieved as it were a regular variable and ",
#> list("x"), " is\n", "materialized as a regular value.\n"),
#> "\n", list("\n", "For a future created via a future assignment, ",
#> list("x %<-% value"), " or\n", list("futureAssign(\"x\", value)"),
#> ", the value is bound to a promise, which when\n",
#> "queried will internally call ", list(list(
#> "value()")), " on the future and which will then\n",
#> "be resolved into a regular variable bound to that value. For example, with\n",
#> "future assignment ", list("x %<-% value"),
#> ", the first time variable ", list("x"), " is queried\n",
#> "the call blocks if, and only if, the future is not yet resolved. As soon\n",
#> "as it is resolved, and any succeeding queries, querying ",
#> list("x"), " will\n", "immediately give the value.\n",
#> "\n", "The future assignment construct ", list(
#> "x %<-% value"), " is not a formal assignment\n",
#> "per se, but a binary infix operator on objects ",
#> list("x"), " and expression ", list("value"),
#> ".\n", "However, by using non-standard evaluation, this constructs can emulate an\n",
#> "assignment operator similar to ", list("x <- value"),
#> ". Due to ", list(), "'s precedence rules\n",
#> "of operators, future expressions often need to be explicitly bracketed,\n",
#> "e.g. ", list("x %<-% { a + b }"), ".\n"),
#> "\n", list(list("Adjust future arguments of a future assignment"),
#> list("\n", "\n", "\n", list(list("future()")),
#> " and ", list(list("futureAssign()")), " take several arguments that can be used\n",
#> "to explicitly specify what global variables and packages the future should\n",
#> "use. They can also be used to override default behaviors of the future,\n",
#> "e.g. whether output should be relayed or not. When using a future\n",
#> "assignment, these arguments can be specified via corresponding\n",
#> "assignment expression. For example, ",
#> list("x %<-% { rnorm(10) } %seed% TRUE"),
#> "\n", "corresponds to ", list("futureAssign(\"x\", { rnorm(10) }, seed = TRUE)"),
#> ". Here are\n", "a several examples.\n",
#> "\n", "To explicitly specify variables and functions that a future assignment\n",
#> "should use, use ", list("%globals%"), ". To explicitly specify which packages need\n",
#> "to be attached for the evaluate to success, use ",
#> list("%packages%"), ". For\n", "example,\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x <- rnorm(1000)\n", "> y %<-% { median(x) } %globals% list(x = x) %packages% \"stats\"\n",
#> "> y\n", "[1] -0.03956372\n"), list(list(
#> "html"), list(list("</div>"))), "\n", "\n",
#> "The ", list("median()"), " function is part of the 'stats' package.\n",
#> "\n", "To declare that you will generate random numbers, use ",
#> list("%seed%"), ", e.g.\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { rnorm(3) } %seed% TRUE\n",
#> "> x\n", "[1] -0.2590562 -1.2262495 0.8858702\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n", "\n", "To disable relaying of standard output (e.g. ",
#> list("print()"), ", ", list("cat()"), ", and\n",
#> list("str()"), "), while keeping relaying of conditions (e.g. ",
#> list("message()"), " and\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { cat(\"Hello\\n\"); message(\"Hi there\"); 42 } %stdout% FALSE\n",
#> "> y <- 13\n", "> z <- x + y\n", "Hi there\n",
#> "> z\n", "[1] 55\n"), list(list("html"),
#> list(list("</div>"))), "\n", "\n", "To disable relaying of conditions, use ",
#> list("%conditions%"), ", e.g.\n", "\n", list(
#> list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { cat(\"Hello\\n\"); message(\"Hi there\"); 42 } %conditions% character(0)\n",
#> "> y <- 13\n", "> z <- x + y\n", "Hello\n",
#> "> z\n", "[1] 55\n"), list(list("html"),
#> list(list("</div>"))), "\n", "\n", list(
#> list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { print(1:10); message(\"Hello\"); 42 } %stdout% FALSE\n",
#> "> y <- 13\n", "> z <- x + y\n", "Hello\n",
#> "> z\n", "[1] 55\n"), list(list("html"),
#> list(list("</div>"))), "\n", "\n", "To create a future without launching in such that it will only be\n",
#> "processed if the value is really needed, use ",
#> list("%lazy%"), ", e.g.\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { Sys.sleep(5); 42 } %lazy% TRUE\n",
#> "> y <- sum(1:10)\n", "> system.time(z <- x + y)\n",
#> " user system elapsed \n", " 0.004 0.000 5.008\n",
#> "> z\n", "[1] 97\n"), list(list("html"),
#> list(list("</div>"))), "\n")), "\n", "\n",
#> list(list("Error handling"), list("\n", "\n",
#> "\n", "Because future assignments are promises, errors produced by the the\n",
#> "future expression will not be signaled until the value of the future is\n",
#> "requested. For example, if you create a future assignment that produce\n",
#> "an error, you will not be affected by the error until you \"touch\" the\n",
#> "future-assignment variable. For example,\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { stop(\"boom\") }\n", "> y <- sum(1:10)\n",
#> "> z <- x + y\n", "Error in eval(quote({ : boom\n"),
#> list(list("html"), list(list("</div>"))), "\n")),
#> "\n", "\n", list(list("Use alternative future backend for future assignment"),
#> list("\n", "\n", "\n", "Futures are evaluated on the future backend that the user has specified\n",
#> "by ", list(list("plan()")), ". With regular futures, we can temporarily use another future\n",
#> "backend by wrapping our code in ", list(
#> "with(plan(...), { ... }]"), ", or temporarily\n",
#> "inside a function using ", list("with(plan(...), local = TRUE)"),
#> ". To achieve the\n", "same for a specific future assignment, use ",
#> list("%plan%"), ", e.g.\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode\">"))),
#> list("> plan(multisession)\n", "> x %<-% { 42 }\n",
#> "> y %<-% { 13 } %plan% sequential\n",
#> "> z <- x + y\n", "> z\n", "[1] 55\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n", "\n", "Here ", list("x"), " is resolved in the background via the ",
#> list("multisession"), " backend,\n", "whereas ",
#> list("y"), " is resolved sequentially in the main R session.\n")),
#> "\n", "\n", list(list("Getting the future object of a future assignment"),
#> list("\n", "\n", "\n", "The underlying ", list(
#> "Future"), " of a future variable ", list(
#> "x"), " can be retrieved without\n", "blocking using ",
#> list("f <- ", list("futureOf"), "(x)"), ", e.g.\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("> x %<-% { stop(\"boom\") }\n", "> f_x <- futureOf(x)\n",
#> "> resolved(f_x)\n", "[1] TRUE\n", "> x\n",
#> "Error in eval(quote({ : boom\n", "> value(f_x)\n",
#> "Error in eval(quote({ : boom\n"), list(
#> list("html"), list(list("</div>"))), "\n",
#> "\n", "Technically, both the future and the variable (promise) are assigned at\n",
#> "the same time to environment ", list("assign.env"),
#> " where the name of the future is\n", list(
#> ".future_<name>"), ".\n")), "\n", "\n"),
#> futureOf.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/delayed_api-futureOf.R",
#> "\n", list("futureOf"), "\n", list("futureOf"),
#> "\n", list("Get the future of a future variable"),
#> "\n", list("\n", "futureOf(\n", " var = NULL,\n",
#> " envir = parent.frame(),\n", " mustExist = TRUE,\n",
#> " default = NA,\n", " drop = FALSE\n", ")\n"),
#> "\n", list("\n", list(list("var"), list("the variable. If NULL, all futures in the\n",
#> "environment are returned.")), "\n", "\n",
#> list(list("envir"), list("the environment where to search from.")),
#> "\n", "\n", list(list("mustExist"), list("If TRUE and the variable does not exists, then\n",
#> "an informative error is thrown, otherwise NA is returned.")),
#> "\n", "\n", list(list("default"), list("the default value if future was not found.")),
#> "\n", "\n", list(list("drop"), list("if TRUE and ",
#> list("var"), " is NULL, then returned list\n",
#> "only contains futures, otherwise also ",
#> list("default"), " values.")), "\n"), "\n",
#> list("\n", "A ", list("Future"), " (or ", list(
#> "default"), ").\n", "If ", list("var"), " is NULL, then a named list of Future:s are returned.\n"),
#> "\n", list("\n", "Get the future of a future variable that has been created directly\n",
#> "or indirectly via ", list(list("future()")),
#> ".\n"), "\n", list("\n", "a %<-% { 1 }\n",
#> "\n", "f <- futureOf(a)\n", "print(f)\n", "\n",
#> "b %<-% { 2 }\n", "\n", "f <- futureOf(b)\n",
#> "print(f)\n", "\n", "## All futures\n", "fs <- futureOf()\n",
#> "print(fs)\n", "\n", "\n", "## Futures part of environment\n",
#> "env <- new.env()\n", "env$c %<-% { 3 }\n",
#> "\n", "f <- futureOf(env$c)\n", "print(f)\n",
#> "\n", "f2 <- futureOf(c, envir = env)\n", "print(f2)\n",
#> "\n", "f3 <- futureOf(\"c\", envir = env)\n",
#> "print(f3)\n", "\n", "fs <- futureOf(envir = env)\n",
#> "print(fs)\n"), "\n"), futureSessionInfo.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-futureSessionInfo.R",
#> "\n", list("futureSessionInfo"), "\n", list("futureSessionInfo"),
#> "\n", list("Get future-specific session information and validate current backend"),
#> "\n", list("\n", "futureSessionInfo(test = TRUE, anonymize = TRUE)\n"),
#> "\n", list("\n", list(list("test"), list("If TRUE, one or more futures are created to query workers\n",
#> "and validate their information.")), "\n",
#> "\n", list(list("anonymize"), list("If TRUE, user names and host names are anonymized.")),
#> "\n"), "\n", list("\n", "Nothing.\n"), "\n",
#> list("\n", "Get future-specific session information and validate current backend\n"),
#> "\n", list("\n", "plan(multisession, workers = 2)\n",
#> "futureSessionInfo()\n", "plan(sequential)\n"),
#> "\n"), futures.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-futures.R",
#> "\n", list("futures"), "\n", list("futures"),
#> "\n", list("Get all futures in a container"),
#> "\n", list("\n", "futures(x, ...)\n"), "\n",
#> list("\n", list(list("x"), list("An environment, a list, or a list environment.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "An object of same type as ",
#> list("x"), " and with the same names\n", "and/or dimensions, if set.\n"),
#> "\n", list("\n", "Gets all futures in an environment, a list, or a list environment\n",
#> "and returns an object of the same class (and dimensions).\n",
#> "Non-future elements are returned as is.\n"),
#> "\n", list("\n", "This function is useful for retrieve futures that were created via\n",
#> "future assignments (", list("%<-%"), ") and therefore stored as promises.\n",
#> "This function turns such promises into standard ",
#> list("Future"), "\n", "objects.\n"), "\n"),
#> getExpression.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("getExpression"), "\n", list("getExpression"),
#> "\n", list("getExpression.Future"), "\n", list(
#> "Inject code for the next type of future to use for nested futures"),
#> "\n", list("\n", "getExpression(future, ...)\n"),
#> "\n", list("\n", list(list("future"), list("Current future.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A future expression with code injected to set what\n",
#> "type of future to use for nested futures, iff any.\n"),
#> "\n", list("\n", "Inject code for the next type of future to use for nested futures\n"),
#> "\n", list("\n", "If there is no future backend specified after this one, the default\n",
#> "is to use ", list("sequential"), " futures. This conservative approach protects\n",
#> "against spawning off recursive futures by mistake, especially\n",
#> list("multicore"), " and ", list("multisession"),
#> " ones.\n", "The default will also set ", list(
#> "options(mc.cores = 1L)"), " (*) so that\n",
#> "no parallel ", list(), " processes are spawned off by functions such as\n",
#> list("parallel::mclapply()"), " and friends.\n",
#> "\n", "Currently it is not possible to specify what type of nested\n",
#> "futures to be used, meaning the above default will always be\n",
#> "used.\n", "See ", list(list("https://github.com/futureverse/future/issues/37"),
#> list("Issue #37")), "\n", "for plans on adding support for custom nested future types.\n",
#> "\n", "(*) Ideally we would set ", list("mc.cores = 0"),
#> " but that will unfortunately\n", "cause ",
#> list("mclapply()"), " and friends to generate an error saying\n",
#> "\"'mc.cores' must be >= 1\". Ideally those functions should\n",
#> "fall back to using the non-multicore alternative in this\n",
#> "case, e.g. ", list("mclapply(...)"), " => ",
#> list("lapply(...)"), ".\n", "See ", list("https://github.com/HenrikBengtsson/Wishlist-for-R/issues/7"),
#> "\n", "for a discussion on this.\n"), "\n",
#> list("internal"), "\n"), getGlobalsAndPackages.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-globals.R",
#> "\n", list("getGlobalsAndPackages"), "\n", list(
#> "getGlobalsAndPackages"), "\n", list("Retrieves global variables of an expression and their associated packages"),
#> "\n", list("\n", "getGlobalsAndPackages(\n",
#> " expr,\n", " envir = parent.frame(),\n",
#> " tweak = tweakExpression,\n", " globals = TRUE,\n",
#> " locals = getOption(\"future.globals.globalsOf.locals\", TRUE),\n",
#> " resolve = getOption(\"future.globals.resolve\"),\n",
#> " persistent = FALSE,\n", " maxSize = getOption(\"future.globals.maxSize\", 500 * 1024^2),\n",
#> " onReference = getOption(\"future.globals.onReference\", \"ignore\"),\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "expr"), list("An ", list(), " expression whose globals should be found.")),
#> "\n", "\n", list(list("envir"), list("The environment from which globals should be searched.")),
#> "\n", "\n", list(list("tweak"), list("(optional) A function that takes an expression and returned a modified one.")),
#> "\n", "\n", list(list("globals"), list("(optional) a logical, a character vector, a named list, or a ",
#> list("Globals"), " object. If TRUE, globals are identified by code inspection based on ",
#> list("expr"), " and ", list("tweak"), " searching from environment ",
#> list("envir"), ". If FALSE, no globals are used. If a character vector, then globals are identified by lookup based their names ",
#> list("globals"), " searching from environment ",
#> list("envir"), ". If a named list or a Globals object, the globals are used as is.")),
#> "\n", "\n", list(list("locals"), list("Should globals part of any \"local\" environment of\n",
#> "a function be included or not?")), "\n",
#> "\n", list(list("resolve"), list("If TRUE, any future that is a global variables (or part of one) is resolved and replaced by a \"constant\" future.")),
#> "\n", "\n", list(list("persistent"), list("If TRUE, non-existing globals (= identified in expression but not found in memory) are always silently ignored and assumed to be existing in the evaluation environment. If FALSE, non-existing globals are by default ignored, but may also trigger an informative error if option ",
#> list("future.globals.onMissing"), " in ",
#> list("\"error\""), " (should only be used for troubleshooting).")),
#> "\n", "\n", list(list("maxSize"), list("The maximum allowed total size (in bytes) of globals---for\n",
#> "the purpose of preventing too large exports / transfers happening by\n",
#> "mistake. If the total size of the global objects are greater than this\n",
#> "limit, an informative error message is produced. If\n",
#> list("maxSize = +Inf"), ", then this assertion is skipped. (Default: 500 MiB).")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A named list with elements ",
#> list("expr"), " (the tweaked expression), ",
#> list("globals"), " (a named list of class ",
#> list("FutureGlobals"), ") and ", list("packages"),
#> " (a character string).\n"), "\n", list("\n",
#> "Retrieves global variables of an expression and their associated packages\n"),
#> "\n", list("\n", "Internally, ", list(list("globalsOf"),
#> "()"), " is used to identify globals and associated packages from the expression.\n"),
#> "\n", list("internal"), "\n"), makeClusterFuture.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-makeClusterFuture.R",
#> "\n", list("makeClusterFuture"), "\n", list("makeClusterFuture"),
#> "\n", list("FUTURE"), "\n", list("Create a Future Cluster of Stateless Workers for Parallel Processing"),
#> "\n", list("\n", "makeClusterFuture(specs = nbrOfWorkers(), ...)\n"),
#> "\n", list("\n", list(list("specs"), list("Ignored.\n",
#> "If specified, the value should equal ", list(
#> "nbrOfWorkers()"), " (default).\n", "A missing value corresponds to specifying ",
#> list("nbrOfWorkers()"), ".\n", "This argument exists only to support\n",
#> list("parallel::makeCluster(NA, type = future::FUTURE)"),
#> ".")), "\n", "\n", list(list(list()), list(
#> "Named arguments passed to ", list(list("future()")),
#> ".")), "\n"), "\n", list("\n", "Returns a ",
#> list("parallel"), " ", list("cluster"), " object of class ",
#> list("FutureCluster"), ".\n"), "\n", list("\n",
#> list("WARNING: Please note that this sets up a stateless set of cluster nodes,\n",
#> "which means that ", list("clusterEvalQ(cl, { a <- 3.14 })"),
#> " will have no effect.\n", "Consider this a first beta version and use it with great care,\n",
#> "particularly because of the stateless nature of the cluster.\n",
#> "For now, I recommend to manually validate that you can get identical\n",
#> "results using this cluster type with what you get from using the\n",
#> "classical ", list("parallel::makeCluster()"),
#> " cluster type."), "\n"), "\n", list(list(
#> "Future Clusters are Stateless"), list("\n",
#> "\n", "Traditionally, a cluster nodes has a one-to-one mapping to a cluster\n",
#> "worker process. For example, ", list("cl <- makeCluster(2, type = \"PSOCK\")"),
#> "\n", "launches two parallel worker processes in the background, where\n",
#> "cluster node ", list("cl[[1]]"), " maps to worker #1 and node ",
#> list("cl[[2]]"), " to\n", "worker #2, and that never changes through the lifespan of these\n",
#> "workers. This one-to-one mapping allows for deterministic\n",
#> "configuration of workers. For examples, some code may assign globals\n",
#> "with values specific to each worker, e.g.\n",
#> list("clusterEvalQ(cl[1], { a <- 3.14 })"),
#> " and\n", list("clusterEvalQ(cl[2], { a <- 2.71 })"),
#> ".\n", "\n", "In contrast, there is no one-to-one mapping between cluster nodes\n",
#> "and the parallel workers when using a future cluster. This is because\n",
#> "we cannot make assumptions on where are parallel task will be\n",
#> "processed. Where a parallel task is processes is up to the future\n",
#> "backend to decide - some backends do this deterministically, whereas\n",
#> "others other resolves task at the first available worker. Also, the\n",
#> "worker processes might be ", list("transient"),
#> " for some future backends, i.e.\n", "the only exist for the life-span of the parallel task and then\n",
#> "terminates.\n", "\n", "Because of this, one must not rely in node-specific behaviors,\n",
#> "because that concept does not make sense with a future cluster.\n",
#> "To protect against this, any attempt to address a subset of future\n",
#> "cluster nodes, results in an error, e.g. ",
#> list("clusterEvalQ(cl[1], ...)"), ",\n", list(
#> "clusterEvalQ(cl[1:2], ...)"), ", and ",
#> list("clusterEvalQ(cl[2:1], ...)"), " in\n",
#> "the above example will all give an error.\n",
#> "\n", "Exceptions to the latter limitation are ",
#> list("clusterSetRNGStream()"), "\n", "and ",
#> list("clusterExport()"), ", which can be safely used with future clusters.\n",
#> "See below for more details.\n", "If ", list(
#> "clusterEvalQ()"), " is called, the call is ignored, and a warning\n",
#> "is produced.\n")), "\n", "\n", list(list("clusterSetRNGStream"),
#> list("\n", "\n", list(list("parallel::clusterSetRNGStream()")),
#> " distributes \"L'Ecuyer-CMRG\" RNG\n", "streams to the cluster nodes, which record them such that the next\n",
#> "round of futures will use them. When used, the RNG state after the\n",
#> "futures are resolved are recorded accordingly, such that the next\n",
#> "round again of future will use those, and so on. This strategy\n",
#> "makes sure ", list("clusterSetRNGStream()"),
#> " has the expected effect although\n", "futures are stateless.\n")),
#> "\n", "\n", list(list("clusterExport"), list(
#> "\n", "\n", list(list("parallel::clusterExport()")),
#> " assign values to the cluster nodes.\n", "Specifically, these values are recorded and are used as globals\n",
#> "for all futures created there on.\n")), "\n",
#> "\n", list("\n", list("if ((getRversion() >= \"4.4.0\")) (if (getRversion() >= \"3.4\") withAutoprint else force)({ # examplesIf"),
#> "\n", "plan(multisession)\n", "cl <- makeClusterFuture()\n",
#> "\n", "parallel::clusterSetRNGStream(cl)\n",
#> "\n", "y <- parallel::parLapply(cl, 11:13, function(x) {\n",
#> " message(\"Process ID: \", Sys.getpid())\n",
#> " mean(rnorm(n = x))\n", "})\n", "str(y)\n",
#> "\n", "plan(sequential)\n", list("}) # examplesIf"),
#> "\n"), "\n", list("internal"), "\n"), mandelbrot.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/demo_api-mandelbrot.R",
#> "\n", list("mandelbrot"), "\n", list("mandelbrot"),
#> "\n", list("as.raster.Mandelbrot"), "\n", list(
#> "plot.Mandelbrot"), "\n", list("mandelbrot_tiles"),
#> "\n", list("mandelbrot.matrix"), "\n", list("mandelbrot.numeric"),
#> "\n", list("Mandelbrot convergence counts"),
#> "\n", list("\n", "mandelbrot(...)\n", "\n", list(
#> list("mandelbrot"), list("matrix")), "(Z, maxIter = 200L, tau = 2, ...)\n",
#> "\n", list(list("mandelbrot"), list("numeric")),
#> "(\n", " xmid = -0.75,\n", " ymid = 0,\n",
#> " side = 3,\n", " resolution = 400L,\n",
#> " maxIter = 200L,\n", " tau = 2,\n", " ...\n",
#> ")\n"), "\n", list("\n", list(list("Z"), list(
#> "A complex matrix for which convergence\n",
#> "counts should be calculated.")), "\n", "\n",
#> list(list("maxIter"), list("Maximum number of iterations per bin.")),
#> "\n", "\n", list(list("tau"), list("A threshold; the radius when calling\n",
#> "divergence (Mod(z) > tau).")), "\n", "\n",
#> list(list("xmid, ymid, side, resolution"),
#> list("Alternative specification of\n", "the complex plane ",
#> list("Z"), ", where\n", list("mean(Re(Z)) == xmid"),
#> ",\n", list("mean(Im(Z)) == ymid"), ",\n",
#> list("diff(range(Re(Z))) == side"), ",\n",
#> list("diff(range(Im(Z))) == side"), ", and\n",
#> list("dim(Z) == c(resolution, resolution)"),
#> ".")), "\n"), "\n", list("\n", "Returns an integer matrix (of class Mandelbrot) with\n",
#> "non-negative counts.\n"), "\n", list("\n",
#> "Mandelbrot convergence counts\n"), "\n", list(
#> "\n", "counts <- mandelbrot(xmid = -0.75, ymid = 0, side = 3)\n",
#> "str(counts)\n", list("\n", "plot(counts)\n"),
#> "\n", "\n", list("\n", "demo(\"mandelbrot\", package = \"future\", ask = FALSE)\n"),
#> "\n", "\n"), "\n", list("\n", "The internal Mandelbrot algorithm was inspired by and\n",
#> "adopted from similar GPL code of Martin Maechler available\n",
#> "from ftp://stat.ethz.ch/U/maechler/R/ on 2005-02-18 (sic!).\n"),
#> "\n", list("internal"), "\n"), multicore.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("multicore"), "\n", list("multicore"),
#> "\n", list("Create a multicore future whose value will be resolved asynchronously in a forked parallel process"),
#> "\n", list("\n", "multicore(\n", " ...,\n",
#> " workers = availableCores(constraints = \"multicore\"),\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " envir = parent.frame()\n", ")\n"), "\n",
#> list("\n", list(list("workers"), list("The number of parallel processes to use.\n",
#> "If a function, it is called without arguments ",
#> list("when the future\n", "is created"), " and its value is used to configure the workers.")),
#> "\n", "\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(
#> list()), list("Additional named elements to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A ", list("Future"), ".\n", "If ",
#> list("workers == 1"), ", then all processing using done in the\n",
#> "current/main ", list(), " session and we therefore fall back to using a\n",
#> "sequential future. To override this fallback, use ",
#> list("workers = I(1)"), ".\n", "This is also the case whenever multicore processing is not supported,\n",
#> "e.g. on Windows.\n"), "\n", list("\n", list(
#> "WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A multicore future is a future that uses multicore evaluation,\n",
#> "which means that its ", list("value is computed and resolved in\n",
#> "parallel in another process"), ".\n", "\n",
#> "This function is must ", list("not"), " be called directly. Instead, the\n",
#> "typical usages are:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures in parallel on the local machine via as many forked\n",
#> "# processes as available to the current R process\n",
#> "plan(multicore)\n", "\n", "# Evaluate futures in parallel on the local machine via two forked processes\n",
#> "plan(multicore, workers = 2)\n"), list(list(
#> "html"), list(list("</div>"))), "\n"), "\n",
#> list(list("Support for forked (\"multicore\") processing"),
#> list("\n", "\n", "Not all operating systems support process forking and thereby not multicore\n",
#> "futures. For instance, forking is not supported on Microsoft Windows.\n",
#> "Moreover, process forking may break some R environments such as RStudio.\n",
#> "Because of this, the future package disables process forking also in\n",
#> "such cases. See ", list(list("parallelly::supportsMulticore()")),
#> " for details.\n", "Trying to create multicore futures on non-supported systems or when\n",
#> "forking is disabled will result in multicore futures falling back to\n",
#> "becoming ", list("sequential"), " futures. If used in RStudio, there will be an\n",
#> "informative warning:\n", "\n", list(list(
#> "html"), list(list("<div class=\"sourceCode r\">"))),
#> list("> plan(multicore)\n", "Warning message:\n",
#> "In supportsMulticoreAndRStudio(...) :\n",
#> " [ONE-TIME WARNING] Forked processing ('multicore') is not supported when\n",
#> "running R from RStudio because it is considered unstable. For more details,\n",
#> "how to control forked processing or not, and how to silence this warning in\n",
#> "future R sessions, see ?parallelly::supportsMulticore\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n")), "\n", "\n", list("\n", "## Use multicore futures\n",
#> "plan(multicore)\n", "\n", "## A global variable\n",
#> "a <- 0\n", "\n", "## Create future (explicitly)\n",
#> "f <- future({\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "\n", "## A multicore future is evaluated in a separate forked\n",
#> "## process. Changing the value of a global variable\n",
#> "## will not affect the result of the future.\n",
#> "a <- 7\n", "print(a)\n", "\n", "v <- value(f)\n",
#> "print(v)\n", "stopifnot(v == 0)\n"), "\n",
#> list("\n", "For processing in multiple background ",
#> list(), " sessions, see\n", list("multisession"),
#> " futures.\n", "\n", "For alternative future backends, see the 'A Future for R: Available Future\n",
#> "Backends' vignette and ", list("https://www.futureverse.org/backends.html"),
#> ".\n", "\n", "Use ", list(list("parallelly::availableCores()")),
#> " to see the total number of\n", "cores that are available for the current ",
#> list(), " session.\n", "Use ", list(list("availableCores"),
#> "(\"multicore\") > 1L"), " to check\n", "whether multicore futures are supported or not on the current\n",
#> "system.\n"), "\n"), multisession.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in", "\n",
#> "% R/backend_api-13.MultisessionFutureBackend-class.R",
#> "\n", list("multisession"), "\n", list("multisession"),
#> "\n", list("Create a multisession future whose value will be resolved asynchronously in a parallel ",
#> list(), " session"), "\n", list("\n", "multisession(\n",
#> " ...,\n", " workers = availableCores(),\n",
#> " lazy = FALSE,\n", " rscript_libs = .libPaths(),\n",
#> " gc = FALSE,\n", " earlySignal = FALSE,\n",
#> " envir = parent.frame()\n", ")\n"), "\n",
#> list("\n", list(list("workers"), list("The number of parallel processes to use.\n",
#> "If a function, it is called without arguments ",
#> list("when the future\n", "is created"), " and its value is used to configure the workers.")),
#> "\n", "\n", list(list("lazy"), list("If FALSE (default), the future is resolved\n",
#> "eagerly (starting immediately), otherwise not.")),
#> "\n", "\n", list(list("rscript_libs"), list(
#> "A character vector of ", list(), " package library folders that\n",
#> "the workers should use. The default is ",
#> list(".libPaths()"), " so that multisession\n",
#> "workers inherits the same library path as the main ",
#> list(), " session.\n", "To avoid this, use ",
#> list("plan(multisession, ..., rscript_libs = NULL)"),
#> ".\n", list("Important: Note that the library path is set on the workers when they are\n",
#> "created, i.e. when ", list("plan(multisession)"),
#> " is called. Any changes to\n", list(".libPaths()"),
#> " in the main R session after the workers have been created\n",
#> "will have no effect."), "\n", "This is passed down as-is to ",
#> list(list("parallelly::makeClusterPSOCK()")),
#> ".")), "\n", "\n", list(list("gc"), list(
#> "If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ",
#> list("earlySignal"), " is TRUE (more\n",
#> "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(
#> list()), list("Additional arguments passed to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A MultisessionFuture.\n", "If ",
#> list("workers == 1"), ", then all processing is done in the\n",
#> "current/main ", list(), " session and we therefore fall back to using a\n",
#> "lazy future. To override this fallback, use ",
#> list("workers = I(1)"), ".\n"), "\n", list(
#> "\n", list("WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A multisession future is a future that uses multisession evaluation,\n",
#> "which means that its ", list("value is computed and resolved in\n",
#> "parallel in another ", list(), " session"),
#> ".\n", "\n", "This function is must ", list(
#> "not"), " be called directly. Instead, the\n",
#> "typical usages are:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures in parallel on the local machine via as many background\n",
#> "# processes as available to the current R process\n",
#> "plan(multisession)\n", "\n", "# Evaluate futures in parallel on the local machine via two background\n",
#> "# processes\n", "plan(multisession, workers = 2)\n"),
#> list(list("html"), list(list("</div>"))), "\n",
#> "\n", "The background ", list(), " sessions (the \"workers\") are created using\n",
#> list(list("makeClusterPSOCK()")), ".\n", "\n",
#> "For the total number of\n", list(), " sessions available including the current/main ",
#> list(), " process, see\n", list(list("parallelly::availableCores()")),
#> ".\n", "\n", "A multisession future is a special type of cluster future.\n"),
#> "\n", list("\n", list("\n", "\n", "## Use multisession futures\n",
#> "plan(multisession)\n", "\n", "## A global variable\n",
#> "a <- 0\n", "\n", "## Create future (explicitly)\n",
#> "f <- future({\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "\n", "## A multisession future is evaluated in a separate R session.\n",
#> "## Changing the value of a global variable will not affect\n",
#> "## the result of the future.\n", "a <- 7\n",
#> "print(a)\n", "\n", "v <- value(f)\n", "print(v)\n",
#> "stopifnot(v == 0)\n", "\n", "## Explicitly close multisession workers by switching plan\n",
#> "plan(sequential)\n"), "\n"), "\n", list("\n",
#> "For processing in multiple forked ", list(),
#> " sessions, see\n", list("multicore"), " futures.\n",
#> "\n", "Use ", list(list("parallelly::availableCores()")),
#> " to see the total number of\n", "cores that are available for the current ",
#> list(), " session.\n"), "\n"), nbrOfWorkers.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-nbrOfWorkers.R",
#> "\n", list("nbrOfWorkers"), "\n", list("nbrOfWorkers"),
#> "\n", list("nbrOfFreeWorkers"), "\n", list("Get the number of workers available"),
#> "\n", list("\n", "nbrOfWorkers(evaluator = NULL)\n",
#> "\n", "nbrOfFreeWorkers(evaluator = NULL, background = FALSE, ...)\n"),
#> "\n", list("\n", list(list("evaluator"), list(
#> "A future evaluator function.\n", "If NULL (default), the current evaluator as returned\n",
#> "by ", list(list("plan()")), " is used.")),
#> "\n", "\n", list(list("background"), list("If TRUE, only workers that can process a future in the\n",
#> "background are considered. If FALSE, also workers running in the main ",
#> list(), "\n", "process are considered, e.g. when using the 'sequential' backend.")),
#> "\n", "\n", list(list(list()), list("Not used; reserved for future use.")),
#> "\n"), "\n", list("\n", list("nbrOfWorkers()"),
#> " returns a positive number in ", list(list(
#> "{1, 2, 3, ...}")), ", which\n", "for some future backends may also be ",
#> list("+Inf"), ".\n", "\n", list("nbrOfFreeWorkers()"),
#> " returns a non-negative number in\n", list(
#> list("{0, 1, 2, 3, ...}")), " which is less than or equal to ",
#> list("nbrOfWorkers()"), ".\n"), "\n", list(
#> "\n", "Get the number of workers available\n"),
#> "\n", list("\n", "plan(multisession)\n", "nbrOfWorkers() ## == availableCores()\n",
#> "\n", "plan(sequential)\n", "nbrOfWorkers() ## == 1\n"),
#> "\n"), nullcon.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-basic.R",
#> "\n", list("nullcon"), "\n", list("nullcon"),
#> "\n", list("Creates a connection to the system null device"),
#> "\n", list("\n", "nullcon()\n"), "\n", list("\n",
#> "Returns a open, binary ", list(list("base::connection()")),
#> ".\n"), "\n", list("\n", "Creates a connection to the system null device\n"),
#> "\n", list("internal"), "\n"), plan.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-plan-with.R, R/utils_api-plan.R,",
#> "\n", "% R/utils_api-tweak.R", "\n", list("with.FutureStrategyList"),
#> "\n", list("with.FutureStrategyList"), "\n",
#> list("plan"), "\n", list("tweak"), "\n", list(
#> "Evaluate an expression using a temporarily set future plan"),
#> "\n", list("\n", list(list("with"), list("FutureStrategyList")),
#> "(data, expr, ..., local = FALSE, envir = parent.frame(), .cleanup = NA)\n",
#> "\n", "plan(\n", " strategy = NULL,\n", " ...,\n",
#> " substitute = TRUE,\n", " .skip = FALSE,\n",
#> " .call = TRUE,\n", " .cleanup = NA,\n",
#> " .init = TRUE\n", ")\n", "\n", "tweak(strategy, ..., penvir = parent.frame())\n"),
#> "\n", list("\n", list(list("data"), list("The future plan to use temporarily.")),
#> "\n", "\n", list(list("expr"), list("The R expression to be evaluated.")),
#> "\n", "\n", list(list("local"), list("If TRUE, then the future plan specified by ",
#> list("data"), "\n", "is applied temporarily in the calling frame. Argument ",
#> list("expr"), " must\n", "not be specified if ",
#> list("local = TRUE"), ".")), "\n", "\n",
#> list(list("envir"), list("The environment where the future plan should be set and the\n",
#> "expression evaluated.")), "\n", "\n", list(
#> list(".cleanup"), list("(internal) Used to stop implicitly started clusters.")),
#> "\n", "\n", list(list("strategy"), list("An existing future function or the name of one.")),
#> "\n", "\n", list(list("substitute"), list("If ",
#> list("TRUE"), ", the ", list("strategy"),
#> " expression is\n", list("substitute()"),
#> ":d, otherwise not.")), "\n", "\n", list(
#> list(".skip"), list("(internal) If ", list(
#> "TRUE"), ", then attempts to set a future backend\n",
#> "that is the same as what is currently in use, will be skipped.")),
#> "\n", "\n", list(list(".call"), list("(internal) Used for recording the call to this function.")),
#> "\n", "\n", list(list(".init"), list("(internal) Used to initiate workers.")),
#> "\n", "\n", list(list("penvir"), list("The environment used when searching for a future\n",
#> "function by its name.")), "\n", "\n", list(
#> list(list()), list("Named arguments to replace the defaults of existing\n",
#> "arguments.")), "\n"), "\n", list("\n",
#> "The value of the expression evaluated (invisibly).\n",
#> "\n", list("plan()"), " returns a the previous plan invisibly if a new future backend\n",
#> "is chosen, otherwise it returns the current one visibly.\n",
#> "\n", "a future function.\n"), "\n", list("\n",
#> "This function allows ", list("the user"),
#> " to plan the future, more specifically,\n",
#> "it specifies how ", list(list("future()")),
#> ":s are resolved,\n", "e.g. sequentially or in parallel.\n"),
#> "\n", list("\n", "The default backend is ", list(
#> list("sequential")), ", but another one can be set\n",
#> "using ", list("plan()"), ", e.g. ", list("plan(multisession)"),
#> " will launch parallel workers\n", "running in the background, which then will be used to resolve future.\n",
#> "To shut down background workers launched this way, call ",
#> list("plan(sequential)"), ".\n"), "\n", list(
#> list("Built-in evaluation strategies"), list(
#> "\n", "\n", "The ", list("future"), " package provides the following built-in backends:\n",
#> "\n", list("\n", list(list(list(list("sequential")),
#> ":"), list("\n", "Resolves futures sequentially in the current ",
#> list(), " process, e.g.\n", list("plan(sequential)"),
#> ".\n")), "\n", list(list(list(list("multisession")),
#> ":"), list("\n", "Resolves futures asynchronously (in parallel) in separate\n",
#> list(), " sessions running in the background on the same machine, e.g.\n",
#> list("plan(multisession)"), " and ", list(
#> "plan(multisession, workers = 2)"), ".\n")),
#> "\n", list(list(list(list("multicore")),
#> ":"), list("\n", "Resolves futures asynchronously (in parallel) in separate\n",
#> list("forked"), " ", list(), " processes running in the background on\n",
#> "the same machine, e.g.\n", list("plan(multicore)"),
#> " and ", list("plan(multicore, workers = 2)"),
#> ".\n", "This backend is not supported on Windows.\n")),
#> "\n", list(list(list(list("cluster")),
#> ":"), list("\n", "Resolves futures asynchronously (in parallel) in separate\n",
#> list(), " sessions running typically on one or more machines, e.g.\n",
#> list("plan(cluster)"), ", ", list("plan(cluster, workers = 2)"),
#> ", and\n", list("plan(cluster, workers = c(\"n1\", \"n1\", \"n2\", \"server.remote.org\"))"),
#> ".\n")), "\n"), "\n")), "\n", "\n", list(
#> list("Other evaluation strategies available"),
#> list("\n", "\n", "\n", "In addition to the built-in ones, additional parallel backends are\n",
#> "implemented in future-backend packages ",
#> list("future.callr"), " and\n", list("future.mirai"),
#> " that leverage R package ", list("callr"),
#> " and\n", list("mirai"), ":\n", "\n", list(
#> "\n", list(list(list("callr"), ":"), list(
#> "\n", "Similar to ", list("multisession"),
#> ", this resolved futures in parallel in\n",
#> "background ", list(), " sessions on the local machine via the ",
#> list("callr"), "\n", "package, e.g. ",
#> list("plan(future.callr::callr)"), " and\n",
#> list("plan(future.callr::callr, workers = 2)"),
#> ". The difference is that\n", "each future is processed in a fresh parallel R worker, which is\n",
#> "automatically shut down as soon as the future is resolved.\n",
#> "This can help decrease the overall memory. Moreover, contrary\n",
#> "to ", list("multisession"), ", ", list(
#> "callr"), " does not rely on socket connections,\n",
#> "which means it is not limited by the number of connections that\n",
#> list(), " can have open at any time.\n")),
#> "\n", "\n", list(list(list("mirai_multisession"),
#> ":"), list("\n", "Similar to ", list(
#> "multisession"), ", this resolved futures in parallel in\n",
#> "background ", list(), " sessions on the local machine via the ",
#> list("mirai"), "\n", "package, e.g. ",
#> list("plan(future.mirai::mirai_multisession)"),
#> " and\n", list("plan(future.mirai::mirai_multisession, workers = 2)"),
#> ".\n")), "\n", "\n", list(list(list("mirai_cluster"),
#> ":"), list("\n", "Similar to ", list(
#> "cluster"), ", this resolved futures in parallel via\n",
#> "pre-configured ", list(), " ", list(
#> "mirai"), " daemon processes, e.g.\n",
#> list("plan(future.mirai::mirai_cluster)"),
#> ".\n")), "\n"), "\n", "\n", "Another example is the ",
#> list("future.batchtools"), " package, which leverages\n",
#> list("batchtools"), " package, to resolve futures via high-performance compute\n",
#> "(HPC) job schedulers, e.g. LSF, Slurm, TORQUE/PBS, Grid Engine, and\n",
#> "OpenLava;\n", "\n", list("\n", list(list(
#> list("batchtools_slurm"), ":"), list("\n",
#> "The backend resolved futures via the Slurm scheduler, e.g.\n",
#> list("plan(future.batchtools::batchtools_slurm)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_torque"),
#> ":"), list("\n", "The backend resolved futures via the TORQUE/PBS scheduler, e.g.\n",
#> list("plan(future.batchtools::batchtools_torque)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_sge"),
#> ":"), list("\n", "The backend resolved futures via the Grid Engine (SGE, AGE) scheduler,\n",
#> "e.g. ", list("plan(future.batchtools::batchtools_sge)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_lsf"),
#> ":"), list("\n", "The backend resolved futures via the Load Sharing Facility (LSF)\n",
#> "scheduler, e.g. ", list("plan(future.batchtools::batchtools_lsf)"),
#> ".\n")), "\n", "\n", list(list(list("batchtools_openlava"),
#> ":"), list("\n", "The backend resolved futures via the OpenLava scheduler, e.g.\n",
#> list("plan(future.batchtools::batchtools_openlava)"),
#> ".\n")), "\n"), "\n")), "\n", "\n", list(
#> list("For package developers"), list("\n",
#> "\n", "\n", "Please refrain from modifying the future backend inside your packages /\n",
#> "functions, i.e. do not call ", list("plan()"),
#> " in your code. Instead, leave\n", "the control on what backend to use to the end user. This idea is part of\n",
#> "the core philosophy of the future framework---as a developer you can never\n",
#> "know what future backends the user have access to. Moreover, by not making\n",
#> "any assumptions about what backends are available, your code will also work\n",
#> "automatically with any new backends developed after you wrote your code.\n",
#> "\n", "If you think it is necessary to modify the future backend within a\n",
#> "function, then make sure to undo the changes when exiting the function.\n",
#> "This can be achieved by using ", list("with(plan(...), local = TRUE)"),
#> ", e.g.\n", "\n", list("\n", " my_fcn <- function(x) {\n",
#> " with(plan(multisession), local = TRUE)\n",
#> " y <- analyze(x)\n", " summarize(y)\n",
#> " }\n"), "\n", "\n", "This is important because the end-user might have already set the future\n",
#> "strategy elsewhere for other purposes and will most likely not known that\n",
#> "calling your function will break their setup.\n",
#> list("Remember, your package and its functions might be used in a greater\n",
#> "context where multiple packages and functions are involved and those might\n",
#> "also rely on the future framework, so it is important to avoid stepping on\n",
#> "others' toes."), "\n")), "\n", "\n", list(
#> list("Using plan() in scripts and vignettes"),
#> list("\n", "\n", "\n", "When writing scripts or vignettes that use futures, try to place any\n",
#> "call to ", list("plan()"), " as far up (i.e. as early on) in the code as possible.\n",
#> "This will help users to quickly identify where the future plan is set up\n",
#> "and allow them to modify it to their computational resources.\n",
#> "Even better is to leave it to the user to set the ",
#> list("plan()"), " prior to\n", list("source()"),
#> ":ing the script or running the vignette.\n",
#> "If a ", list(list(".future.R")), " exists in the current directory and / or in\n",
#> "the user's home directory, it is sourced when the ",
#> list("future"), " package is\n", list("loaded"),
#> ". Because of this, the ", list(".future.R"),
#> " file provides a\n", "convenient place for users to set the ",
#> list("plan()"), ".\n", "This behavior can be controlled via an ",
#> list(), " option---see\n", list("future options"),
#> " for more details.\n")), "\n", "\n", list(
#> "\n", "# Evaluate a future using the 'multisession' plan\n",
#> "with(plan(multisession, workers = 2), {\n",
#> " f <- future(Sys.getpid())\n", " w_pid <- value(f)\n",
#> "})\n", "print(c(main = Sys.getpid(), worker = w_pid))\n",
#> "\n", "\n", "\n", "# Evaluate a future locally using the 'multisession' plan\n",
#> "local({\n", " with(plan(multisession, workers = 2), local = TRUE)\n",
#> "\n", " f <- future(Sys.getpid())\n", " w_pid <- value(f)\n",
#> " print(c(main = Sys.getpid(), worker = w_pid))\n",
#> "})\n", "\n", "\n", "a <- b <- c <- NA_real_\n",
#> "\n", "# An sequential future\n", "plan(sequential)\n",
#> "f <- future({\n", " a <- 7\n", " b <- 3\n",
#> " c <- 2\n", " a * b * c\n", "})\n", "y <- value(f)\n",
#> "print(y)\n", "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n", "\n", "# A sequential future with lazy evaluation\n",
#> "plan(sequential)\n", "f <- future({\n", " a <- 7\n",
#> " b <- 3\n", " c <- 2\n", " a * b * c\n",
#> "}, lazy = TRUE)\n", "y <- value(f)\n", "print(y)\n",
#> "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n", "\n", "# A multicore future (specified as a string)\n",
#> "plan(\"multicore\")\n", "f <- future({\n",
#> " a <- 7\n", " b <- 3\n", " c <- 2\n", " a * b * c\n",
#> "})\n", "y <- value(f)\n", "print(y)\n", "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n", "## Multisession futures gives an error on R CMD check on\n",
#> "## Windows (but not Linux or macOS) for unknown reasons.\n",
#> "## The same code works in package tests.\n",
#> list("\n", "\n", "# A multisession future (specified via a string variable)\n",
#> "plan(\"future::multisession\")\n", "f <- future({\n",
#> " a <- 7\n", " b <- 3\n", " c <- 2\n",
#> " a * b * c\n", "})\n", "y <- value(f)\n",
#> "print(y)\n", "str(list(a = a, b = b, c = c)) ## All NAs\n",
#> "\n"), "\n", "\n", "\n", "## Explicitly specifying number of workers\n",
#> "## (default is parallelly::availableCores())\n",
#> "plan(multicore, workers = 2)\n", "message(\"Number of parallel workers: \", nbrOfWorkers())\n",
#> "\n", "\n", "## Explicitly close multisession workers by switching plan\n",
#> "plan(sequential)\n"), "\n", list("\n", "Use ",
#> list(list("plan()")), " to set a future to become the\n",
#> "new default strategy.\n"), "\n"), private_length.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-basic.R",
#> "\n", list(".length"), "\n", list(".length"),
#> "\n", list("Gets the length of an object without dispatching"),
#> "\n", list("\n", ".length(x)\n"), "\n", list(
#> "\n", list(list("x"), list("Any ", list(),
#> " object.")), "\n"), "\n", list("\n", "A non-negative integer.\n"),
#> "\n", list("\n", "Gets the length of an object without dispatching\n"),
#> "\n", list("\n", "This function returns ", list(
#> "length(unclass(x))"), ", but tries to avoid\n",
#> "calling ", list("unclass(x)"), " unless necessary.\n"),
#> "\n", list("\n", list(list(".subset"), "()"),
#> " and ", list(list(".subset2"), "()"), ".\n"),
#> "\n", list("internal"), "\n"), `re-exports.Rd` = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/000.re-exports.R",
#> "\n", list("re-exports"), "\n", list("re-exports"),
#> "\n", list("as.cluster"), "\n", list("availableCores"),
#> "\n", list("availableWorkers"), "\n", list("makeClusterPSOCK"),
#> "\n", list("supportsMulticore"), "\n", list("Functions Moved to 'parallelly'"),
#> "\n", list("\n", "The following function used to be part of ",
#> list("future"), " but has since\n", "been migrated to ",
#> list("parallelly"), ". The migration started with\n",
#> list("future"), " 1.20.0 (November 2020). They were moved because they\n",
#> "are also useful outside of the ", list("future"),
#> " framework.\n"), "\n", list("\n", list("If you are using any of these from the ",
#> list("future"), " package, please\n", "switch to use the ones from the ",
#> list("parallelly"), " package. Thank you!"),
#> "\n", list("\n", list(), " ", list(list("parallelly::as.cluster()")),
#> "\n", list(), " ", list(list("parallelly::autoStopCluster()")),
#> " (no longer re-exported)\n", list(), " ",
#> list(list("parallelly::availableCores()")),
#> "\n", list(), " ", list(list("parallelly::availableWorkers()")),
#> "\n", list(), " ", list(list("parallelly::makeClusterMPI()")),
#> " (no longer re-exported)\n", list(),
#> " ", list(list("parallelly::makeClusterPSOCK()")),
#> "\n", list(), " ", list(list("parallelly::makeNodePSOCK()")),
#> " (no longer re-exported)\n", list(),
#> " ", list(list("parallelly::supportsMulticore()")),
#> "\n"), "\n", "\n", "For backward-compatible reasons, ",
#> list("some"), " of these functions remain\n",
#> "available as exact copies also from this package (as re-exports), e.g.\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("cl <- parallelly::makeClusterPSOCK(2)\n"),
#> list(list("html"), list(list("</div>"))), "\n",
#> "\n", "can still be accessed as:\n", "\n",
#> list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("cl <- future::makeClusterPSOCK(2)\n"),
#> list(list("html"), list(list("</div>"))), "\n",
#> "\n", list("Note that it is the goal to remove all of the above from this package."),
#> "\n"), "\n", list("internal"), "\n"), readImmediateConditions.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-immediateCondition.R",
#> "\n", list("readImmediateConditions"), "\n",
#> list("readImmediateConditions"), "\n", list("saveImmediateCondition"),
#> "\n", list("Writes and Reads 'immediateCondition' RDS Files"),
#> "\n", list("\n", "readImmediateConditions(\n",
#> " path = immediateConditionsPath(rootPath = rootPath),\n",
#> " rootPath = tempdir(),\n", " pattern = \"[.]rds$\",\n",
#> " include = getOption(\"future.relay.immediate\", \"immediateCondition\"),\n",
#> " signal = FALSE,\n", " remove = TRUE\n",
#> ")\n", "\n", "saveImmediateCondition(\n", " cond,\n",
#> " path = immediateConditionsPath(rootPath = rootPath),\n",
#> " rootPath = tempdir()\n", ")\n"), "\n", list(
#> "\n", list(list("path"), list("(character string) The folder where the RDS files are.")),
#> "\n", "\n", list(list("pattern"), list("(character string) A regular expression selecting\n",
#> "the RDS files to be read.")), "\n", "\n",
#> list(list("include"), list("(character vector) The class or classes of the objects\n",
#> "to be kept.")), "\n", "\n", list(list("signal"),
#> list("(logical) If TRUE, the condition read are signaled.")),
#> "\n", "\n", list(list("remove"), list("(logical) If TRUE, the RDS files used are removed on exit.")),
#> "\n", "\n", list(list("cond"), list("A condition of class ",
#> list("immediateCondition"), ".")), "\n"),
#> "\n", list("\n", list("readImmediateConditions()"),
#> " returns an unnamed ", list("base::list"),
#> " of\n", "named lists with elements ", list(
#> "condition"), " and ", list("signaled"),
#> ", where\n", "the ", list("condition"), " elements hold ",
#> list("immediateCondition"), " objects.\n",
#> "\n", list("saveImmediateCondition()"), " returns, invisibly, the pathname of\n",
#> "the RDS written.\n"), "\n", list("\n", "Writes and Reads 'immediateCondition' RDS Files\n"),
#> "\n", list("internal"), "\n"), requestCore.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("requestCore"), "\n", list("requestCore"),
#> "\n", list("Request a core for multicore processing"),
#> "\n", list("\n", "requestCore(\n", " await,\n",
#> " workers = availableCores(constraints = \"multicore\"),\n",
#> " timeout,\n", " delta,\n", " alpha\n",
#> ")\n"), "\n", list("\n", list(list("await"),
#> list("A function used to try to \"collect\"\n",
#> "finished multicore subprocesses.")), "\n",
#> "\n", list(list("workers"), list("Total number of workers available.")),
#> "\n", "\n", list(list("timeout"), list("Maximum waiting time (in seconds) allowed\n",
#> "before a timeout error is generated.")),
#> "\n", "\n", list(list("delta"), list("Then base interval (in seconds) to wait\n",
#> "between each try.")), "\n", "\n", list(list(
#> "alpha"), list("A multiplicative factor used to increase\n",
#> "the wait interval after each try.")), "\n"),
#> "\n", list("\n", "Invisible TRUE. If no cores are available after\n",
#> "extensive waiting, then a timeout error is thrown.\n"),
#> "\n", list("\n", "If no cores are available, the current process\n",
#> "blocks until a core is available.\n"), "\n",
#> list("internal"), "\n"), reset.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-reset.R",
#> "\n", list("reset"), "\n", list("reset"), "\n",
#> list("Reset a finished, failed, canceled, or interrupted future to a lazy future"),
#> "\n", list("\n", "reset(x, ...)\n"), "\n", list(
#> "\n", list(list("x"), list("A Future.")), "\n",
#> "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", list("reset()"), " returns a lazy, vanilla ",
#> list("Future"), " that can be relaunched.\n",
#> "Resetting a running future results in a ",
#> list("FutureError"), ".\n"), "\n", list("\n",
#> "A future that has successfully completed, ",
#> list("canceled"), ", interrupted,\n", "or has failed due to an error, can be relaunched after resetting it.\n"),
#> "\n", list("\n", "A lazy, vanilla ", list("Future"),
#> " can be reused in another R session. For\n",
#> "instance, if we do:\n", "\n", list(list("html"),
#> list(list("<div class=\"sourceCode\">"))),
#> list("library(future)\n", "a <- 2\n", "f <- future(42 * a, lazy = TRUE)\n",
#> "saveRDS(f, \"myfuture.rds\")\n"), list(list(
#> "html"), list(list("</div>"))), "\n", "\n",
#> "Then we can read and evaluate the future in another R session using:\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode\">"))),
#> list("library(future)\n", "f <- readRDS(\"myfuture.rds\")\n",
#> "v <- value(f)\n", "print(v)\n", "#> [1] 84\n"),
#> list(list("html"), list(list("</div>"))), "\n"),
#> "\n", list("\n", "## Like mean(), but fails 90% of the time\n",
#> "shaky_mean <- function(x) {\n", " if (as.double(Sys.time()) %% 1 < 0.90) stop(\"boom\")\n",
#> " mean(x)\n", "}\n", "\n", "x <- rnorm(100)\n",
#> "\n", "## Calculate the mean of 'x' with a risk of failing randomly\n",
#> "f <- future({ shaky_mean(x) })\n", "\n", "## Relaunch until success\n",
#> "repeat({\n", " v <- tryCatch(value(f), error = identity)\n",
#> " if (!inherits(v, \"error\")) break\n", " message(\"Resetting failed future, and retry in 0.1 seconds\")\n",
#> " f <- reset(f)\n", " Sys.sleep(0.1)\n",
#> "})\n", "cat(\"mean:\", v, \"\\n\")\n"), "\n"),
#> resetWorkers.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-plan.R",
#> "\n", list("resetWorkers"), "\n", list("resetWorkers"),
#> "\n", list("Free up active background workers"),
#> "\n", list("\n", "resetWorkers(x, ...)\n"), "\n",
#> list("\n", list(list("x"), list("A FutureStrategy.")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "Free up active background workers\n"),
#> "\n", list("\n", "This function will resolve any active futures that is currently\n",
#> "being evaluated on background workers.\n"),
#> "\n", list("\n", "resetWorkers(plan())\n", "\n"),
#> "\n", list("internal"), "\n"), resolve.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-resolve.R",
#> "\n", list("resolve"), "\n", list("resolve"),
#> "\n", list("Resolve one or more futures synchronously"),
#> "\n", list("\n", "resolve(\n", " x,\n", " idxs = NULL,\n",
#> " recursive = 0,\n", " result = FALSE,\n",
#> " stdout = FALSE,\n", " signal = FALSE,\n",
#> " force = FALSE,\n", " sleep = getOption(\"future.wait.interval\", 0.01),\n",
#> " ...\n", ")\n"), "\n", list("\n", list(list(
#> "x"), list("A ", list("Future"), " to be resolved, or a list, an environment, or a\n",
#> "list environment of futures to be resolved.")),
#> "\n", "\n", list(list("idxs"), list("(optional) integer or logical index specifying the subset of\n",
#> "elements to check.")), "\n", "\n", list(
#> list("recursive"), list("A non-negative number specifying how deep of a recursion\n",
#> "should be done. If TRUE, an infinite recursion is used. If FALSE or zero,\n",
#> "no recursion is performed.")), "\n", "\n",
#> list(list("result"), list("(internal) If TRUE, the results are ",
#> list("retrieved"), ", otherwise not.\n",
#> "Note that this only collects the results from the parallel worker, which\n",
#> "can help lower the overall latency if there are multiple concurrent futures.\n",
#> "This does ", list("not"), " return the collected results.")),
#> "\n", "\n", list(list("stdout"), list("(internal) If TRUE, captured standard output is relayed, otherwise not.")),
#> "\n", "\n", list(list("signal"), list("(internal) If TRUE, captured ",
#> list("conditions"), " are relayed,\n", "otherwise not.")),
#> "\n", "\n", list(list("force"), list("(internal) If TRUE, captured standard output and captured\n",
#> list("conditions"), " already relayed is relayed again, otherwise not.")),
#> "\n", "\n", list(list("sleep"), list("Number of seconds to wait before checking if futures have been\n",
#> "resolved since last time.")), "\n", "\n",
#> list(list(list()), list("Not used.")), "\n"),
#> "\n", list("\n", "Returns ", list("x"), " (regardless of subsetting or not).\n",
#> "If ", list("signal"), " is TRUE and one of the futures produces an error, then\n",
#> "that error is produced.\n"), "\n", list("\n",
#> "This function provides an efficient mechanism for waiting for multiple\n",
#> "futures in a container (e.g. list or environment) to be resolved while in\n",
#> "the meanwhile retrieving values of already resolved futures.\n"),
#> "\n", list("\n", "This function is resolves synchronously, i.e. it blocks until ",
#> list("x"), " and\n", "any containing futures are resolved.\n"),
#> "\n", list("\n", "To resolve a future ", list(
#> "variable"), ", first retrieve its\n", list(
#> "Future"), " object using ", list(list("futureOf()")),
#> ", e.g.\n", list("resolve(futureOf(x))"), ".\n"),
#> "\n"), resolved.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-resolved.R",
#> "\n", list("resolved"), "\n", list("resolved"),
#> "\n", list("Check whether a future is resolved or not"),
#> "\n", list("\n", "resolved(x, ...)\n"), "\n",
#> list("\n", list(list("x"), list("A ", list("Future"),
#> ", a list, or an environment (which also\n",
#> "includes ", list("list environment"), ").")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "A logical of the same length and dimensions as ",
#> list("x"), ".\n", "Each element is TRUE unless the corresponding element is a\n",
#> "non-resolved future in case it is FALSE.\n"),
#> "\n", list("\n", "Check whether a future is resolved or not\n"),
#> "\n", list("\n", "This method needs to be implemented by the class that implement\n",
#> "the Future API. The implementation should return either TRUE or FALSE\n",
#> "and must never throw an error (except for ",
#> list("FutureError"), ":s which indicate\n",
#> "significant, often unrecoverable infrastructure problems).\n",
#> "It should also be possible to use the method for polling the\n",
#> "future until it is resolved (without having to wait infinitely long),\n",
#> "e.g. ", list("while (!resolved(future)) Sys.sleep(5)"),
#> ".\n"), "\n"), result.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("result.Future"), "\n", list("result.Future"),
#> "\n", list("result"), "\n", list("Get the results of a resolved future"),
#> "\n", list("\n", list(list("result"), list("Future")),
#> "(future, ...)\n"), "\n", list("\n", list(list(
#> "future"), list("A ", list("Future"), ".")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "The ", list("FutureResult"),
#> " object.\n"), "\n", list("\n", "Get the results of a resolved future\n"),
#> "\n", list("\n", "This function is only part of the ",
#> list("backend"), " Future API.\n", "This function is ",
#> list("not"), " part of the frontend Future API.\n"),
#> "\n", list("internal"), "\n"), run.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-Future-class.R",
#> "\n", list("run.Future"), "\n", list("run.Future"),
#> "\n", list("run"), "\n", list("Run a future"),
#> "\n", list("\n", list(list("run"), list("Future")),
#> "(future, ...)\n"), "\n", list("\n", list(list(
#> "future"), list("A ", list("Future"), ".")),
#> "\n", "\n", list(list(list()), list("Not used.")),
#> "\n"), "\n", list("\n", "The ", list("Future"),
#> " object.\n"), "\n", list("\n", "Run a future\n"),
#> "\n", list("\n", "This function can only be called once per future.\n",
#> "Further calls will result in an informative error.\n",
#> "If a future is not run when its value is queried,\n",
#> "then it is run at that point.\n"), "\n", list(
#> "internal"), "\n"), save_rds.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-immediateCondition.R",
#> "\n", list("save_rds"), "\n", list("save_rds"),
#> "\n", list("Robustly Saves an Object to RDS File Atomically"),
#> "\n", list("\n", "save_rds(object, pathname, ...)\n"),
#> "\n", list("\n", list(list("object"), list("The ",
#> list(), " object to be save.")), "\n", "\n",
#> list(list("pathname"), list("RDS file to written.")),
#> "\n", "\n", list(list(list()), list("(optional) Additional arguments passed to ",
#> list(list("base::saveRDS()")), ".")), "\n"),
#> "\n", list("\n", "The pathname of the RDS written.\n"),
#> "\n", list("\n", "Robustly Saves an Object to RDS File Atomically\n"),
#> "\n", list("\n", "Uses ", list("base::saveRDS"),
#> " internally but writes the object atomically by first\n",
#> "writing to a temporary file which is then renamed.\n"),
#> "\n", list("internal"), "\n"), sequential.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.SequentialFutureBackend-class.R",
#> "\n", list("sequential"), "\n", list("sequential"),
#> "\n", list("uniprocess"), "\n", list("Create a sequential future whose value will be in the current ",
#> list(), " session"), "\n", list("\n", "sequential(..., gc = FALSE, earlySignal = FALSE, envir = parent.frame())\n"),
#> "\n", list("\n", list(list("gc"), list("If TRUE, the garbage collector run (in the process that\n",
#> "evaluated the future) only after the value of the future is collected.\n",
#> "Exactly when the values are collected may depend on various factors such\n",
#> "as number of free workers and whether ", list(
#> "earlySignal"), " is TRUE (more\n", "frequently) or FALSE (less frequently).\n",
#> list("Some types of futures ignore this argument."))),
#> "\n", "\n", list(list("earlySignal"), list(
#> "Specified whether conditions should be signaled as soon\n",
#> "as possible or not.")), "\n", "\n", list(
#> list("envir"), list("The ", list("environment"),
#> " from where global objects should be\n",
#> "identified.")), "\n", "\n", list(list(
#> list()), list("Additional named elements to ",
#> list(list("Future()")), ".")), "\n"), "\n",
#> list("\n", "A ", list("Future"), ".\n"), "\n",
#> list("\n", list("WARNING: This function must never be called.\n",
#> "It may only be used with ", list(list("plan()"))),
#> "\n"), "\n", list("\n", "A sequential future is a future that is evaluated sequentially in the\n",
#> "current ", list(), " session similarly to how ",
#> list(), " expressions are evaluated in ", list(),
#> ".\n", "The only difference to ", list(), " itself is that globals are validated\n",
#> "by default just as for all other types of futures in this package.\n",
#> "\n", "This function is must ", list("not"),
#> " be called directly. Instead, the\n", "typical usages are:\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("# Evaluate futures sequentially in the current R process\n",
#> "plan(sequential)\n"), list(list("html"),
#> list(list("</div>"))), "\n"), "\n", list(
#> "\n", "## Use sequential futures\n", "plan(sequential)\n",
#> "\n", "## A global variable\n", "a <- 0\n",
#> "\n", "## Create a sequential future\n", "f <- future({\n",
#> " b <- 3\n", " c <- 2\n", " a * b * c\n",
#> "})\n", "\n", "## Since 'a' is a global variable in future 'f' which\n",
#> "## is eagerly resolved (default), this global has already\n",
#> "## been resolved / incorporated, and any changes to 'a'\n",
#> "## at this point will _not_ affect the value of 'f'.\n",
#> "a <- 7\n", "print(a)\n", "\n", "v <- value(f)\n",
#> "print(v)\n", "stopifnot(v == 0)\n"), "\n"),
#> sessionDetails.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils_api-sessionDetails.R",
#> "\n", list("sessionDetails"), "\n", list("sessionDetails"),
#> "\n", list("Outputs details on the current ",
#> list(), " session"), "\n", list("\n", "sessionDetails(env = FALSE)\n"),
#> "\n", list("\n", list(list("env"), list("If TRUE, ",
#> list("Sys.getenv()"), " information is returned.")),
#> "\n"), "\n", list("\n", "Invisibly a list of all details.\n"),
#> "\n", list("\n", "Outputs details on the current ",
#> list(), " session\n"), "\n", list("internal"),
#> "\n"), signalConditions.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/protected_api-signalConditions.R",
#> "\n", list("signalConditions"), "\n", list("signalConditions"),
#> "\n", list("Signals Captured Conditions"), "\n",
#> list("\n", "signalConditions(\n", " future,\n",
#> " include = \"condition\",\n", " exclude = NULL,\n",
#> " resignal = TRUE,\n", " ...\n", ")\n"),
#> "\n", list("\n", list(list("future"), list("A resolved ",
#> list("Future"), ".")), "\n", "\n", list(list(
#> "include"), list("A character string of ",
#> list("condition"), "\n", "classes to signal.")),
#> "\n", "\n", list(list("exclude"), list("A character string of ",
#> list("condition"), "\n", "classes ", list(
#> "not"), " to signal.")), "\n", "\n", list(
#> list("resignal"), list("If TRUE, then already signaled conditions are signaled\n",
#> "again, otherwise not.")), "\n", "\n",
#> list(list(list()), list("Not used.")), "\n"),
#> "\n", list("\n", "Returns the ", list("Future"),
#> " where conditioned that were signaled\n",
#> "have been flagged to have been signaled.\n"),
#> "\n", list("\n", "Captured conditions that meet the ",
#> list("include"), " and ", list("exclude"),
#> "\n", "requirements are signaled ", list("in the order as they were captured"),
#> ".\n"), "\n", list("\n", "Conditions are signaled by\n",
#> list(list("signalCondition"), "()"), ".\n"),
#> "\n", list("internal"), "\n"), sticky_globals.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-sticky_globals.R",
#> "\n", list("sticky_globals"), "\n", list("sticky_globals"),
#> "\n", list("Place a sticky-globals environment immediately after the global environment"),
#> "\n", list("\n", "sticky_globals(erase = FALSE, name = \"future:sticky_globals\", pos = 2L)\n"),
#> "\n", list("\n", list(list("erase"), list("(logical) If TRUE, the environment is erased, otherwise not.")),
#> "\n", "\n", list(list("name"), list("(character) The name of the environment on the ",
#> list("base::search"), "\n", "path.")), "\n",
#> "\n", list(list("pos"), list("(integer) The position on the search path where the\n",
#> "environment should be positioned. If ",
#> list("pos == 0L"), ", then the environment\n",
#> "is detached, if it exists.")), "\n"), "\n",
#> list("\n", "(invisible; environment) The environment.\n"),
#> "\n", list("\n", "Place a sticky-globals environment immediately after the global environment\n"),
#> "\n", list("internal"), "\n"), usedCores.Rd = list(
#> "% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/backend_api-11.MulticoreFutureBackend-class.R",
#> "\n", list("usedCores"), "\n", list("usedCores"),
#> "\n", list("Get number of cores currently used"),
#> "\n", list("\n", "usedCores()\n"), "\n", list(
#> "\n", "A non-negative integer.\n"), "\n", list(
#> "\n", "Get number of children (and don't count the current process)\n",
#> "used by the current ", list(), " session. The number of children\n",
#> "is the total number of subprocesses launched by this\n",
#> "process that are still running and whose values have yet\n",
#> "not been collected.\n"), "\n", list("internal"),
#> "\n"), value.Rd = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/core_api-value.R",
#> "\n", list("value"), "\n", list("value"), "\n",
#> list("value.Future"), "\n", list("value.list"),
#> "\n", list("value.listenv"), "\n", list("value.environment"),
#> "\n", list("The value of a future or the values of all elements in a container"),
#> "\n", list("\n", "value(...)\n", "\n", list(list(
#> "value"), list("Future")), "(future, stdout = TRUE, signal = TRUE, drop = FALSE, ...)\n",
#> "\n", list(list("value"), list("list")), "(\n",
#> " x,\n", " idxs = NULL,\n", " recursive = 0,\n",
#> " reduce = NULL,\n", " stdout = TRUE,\n",
#> " signal = TRUE,\n", " cancel = TRUE,\n",
#> " interrupt = cancel,\n", " inorder = TRUE,\n",
#> " drop = FALSE,\n", " force = TRUE,\n", " sleep = getOption(\"future.wait.interval\", 0.01),\n",
#> " ...\n", ")\n", "\n", list(list("value"),
#> list("listenv")), "(\n", " x,\n", " idxs = NULL,\n",
#> " recursive = 0,\n", " reduce = NULL,\n",
#> " stdout = TRUE,\n", " signal = TRUE,\n",
#> " cancel = TRUE,\n", " interrupt = cancel,\n",
#> " inorder = TRUE,\n", " drop = FALSE,\n",
#> " force = TRUE,\n", " sleep = getOption(\"future.wait.interval\", 0.01),\n",
#> " ...\n", ")\n", "\n", list(list("value"),
#> list("environment")), "(x, ...)\n"), "\n",
#> list("\n", list(list("future, x"), list("A ",
#> list("Future"), ", an environment, a list, or a list environment.")),
#> "\n", "\n", list(list("stdout"), list("If TRUE, standard output captured while resolving futures\n",
#> "is relayed, otherwise not.")), "\n", "\n",
#> list(list("signal"), list("If TRUE, ", list(
#> "conditions"), " captured while resolving\n",
#> "futures are relayed, otherwise not.")),
#> "\n", "\n", list(list("drop"), list("If TRUE, resolved futures are minimized in size and invalidated\n",
#> "as soon the as their values have been collected and any output and\n",
#> "conditions have been relayed.\n", "Combining ",
#> list("drop = TRUE"), " with ", list("inorder = FALSE"),
#> " reduces the memory use\n", "sooner, especially avoiding the risk of holding on to future values until\n",
#> "the very end.")), "\n", "\n", list(list(
#> "idxs"), list("(optional) integer or logical index specifying the subset of\n",
#> "elements to check.")), "\n", "\n", list(
#> list("recursive"), list("A non-negative number specifying how deep of a recursion\n",
#> "should be done. If TRUE, an infinite recursion is used. If FALSE or zero,\n",
#> "no recursion is performed.")), "\n", "\n",
#> list(list("reduce"), list("An optional function for reducing all the values.\n",
#> "Optional attribute ", list("init"), " can be used to set initial value for the\n",
#> "reduction. If not specified, the first value will be used as the\n",
#> "initial value.\n", "Reduction of values is done as soon as possible, but always in the\n",
#> "same order as ", list("x"), ", unless ",
#> list("inorder"), " is FALSE.")), "\n", "\n",
#> list(list("cancel, interrupt"), list("If TRUE and ",
#> list("signal"), " is TRUE, non-resolved futures\n",
#> "are canceled as soon as an error is detected in one of the futures,\n",
#> "before signaling the error. Argument ",
#> list("interrupt"), " is passed to ", list(
#> "cancel()"), "\n", "controlling whether non-resolved futures should also be interrupted.")),
#> "\n", "\n", list(list("inorder"), list("If TRUE, then standard output and conditions are relayed,\n",
#> "and value reduction, is done in the order the futures occur in ",
#> list("x"), ", but\n", "always as soon as possible. This is achieved by buffering the details\n",
#> "until they can be released. By setting ",
#> list("inorder = FALSE"), ", no buffering\n",
#> "takes place and everything is relayed and reduced as soon as a new future\n",
#> "is resolved. Regardlessly, the values are always returned in the same\n",
#> "order as ", list("x"), ".")), "\n", "\n",
#> list(list("force"), list("(internal) If TRUE, captured standard output and captured\n",
#> list("conditions"), " already relayed is relayed again, otherwise not.")),
#> "\n", "\n", list(list("sleep"), list("Number of seconds to wait before checking if futures have been\n",
#> "resolved since last time.")), "\n", "\n",
#> list(list(list()), list("All arguments used by the S3 methods.")),
#> "\n"), "\n", list("\n", list("value()"), " of a Future object returns the value of the future, which can\n",
#> "be any type of ", list(), " object.\n", "\n",
#> list("value()"), " of a list, an environment, or a list environment returns an\n",
#> "object with the same number of elements and of the same class.\n",
#> "Names and dimension attributes are preserved, if available.\n",
#> "All future elements are replaced by their corresponding ",
#> list("value()"), " values.\n", "For all other elements, the existing object is kept as-is.\n",
#> "\n", "If ", list("signal"), " is TRUE and one of the futures produces an error, then\n",
#> "that error is relayed. Any remaining, non-resolved futures in ",
#> list("x"), " are\n", "canceled, prior to signaling such an error.\n"),
#> "\n", list("\n", "Gets the value of a future or the values of all elements (including futures)\n",
#> "in a container such as a list, an environment, or a list environment.\n",
#> "If one or more futures is unresolved, then this function blocks until all\n",
#> "queried futures are resolved.\n"), "\n", list(
#> "\n", "## ------------------------------------------------------\n",
#> "## A single future\n", "## ------------------------------------------------------\n",
#> "x <- sample(100, size = 50)\n", "f <- future(mean(x))\n",
#> "v <- value(f)\n", "message(\"The average of 50 random numbers in [1,100] is: \", v)\n",
#> "\n", "\n", "\n", "## ------------------------------------------------------\n",
#> "## Ten futures\n", "## ------------------------------------------------------\n",
#> "xs <- replicate(10, { list(sample(100, size = 50)) })\n",
#> "fs <- lapply(xs, function(x) { future(mean(x)) })\n",
#> "\n", "## The 10 values as a list (because 'fs' is a list)\n",
#> "vs <- value(fs)\n", "message(\"The ten averages are:\")\n",
#> "str(vs)\n", "\n", "## The 10 values as a vector (by manually unlisting)\n",
#> "vs <- value(fs)\n", "vs <- unlist(vs)\n",
#> "message(\"The ten averages are: \", paste(vs, collapse = \", \"))\n",
#> "\n", "## The values as a vector (by reducing)\n",
#> "vs <- value(fs, reduce = `c`)\n", "message(\"The ten averages are: \", paste(vs, collapse = \", \"))\n",
#> "\n", "## Calculate the sum of the averages (by reducing)\n",
#> "total <- value(fs, reduce = `sum`)\n", "message(\"The sum of the ten averages is: \", total)\n"),
#> "\n"), `zzz-future.options.Rd` = list("% Generated by roxygen2: do not edit by hand",
#> "\n", "% Please edit documentation in R/utils-options.R",
#> "\n", list("zzz-future.options"), "\n", list(
#> "zzz-future.options"), "\n", list("future.options"),
#> "\n", list("future.startup.script"), "\n", list(
#> "future.debug"), "\n", list("future.demo.mandelbrot.region"),
#> "\n", list("future.demo.mandelbrot.nrow"), "\n",
#> list("future.fork.multithreading.enable"), "\n",
#> list("future.globals.maxSize"), "\n", list("future.globals.method"),
#> "\n", list("future.globals.onMissing"), "\n",
#> list("future.globals.resolve"), "\n", list("future.globals.onReference"),
#> "\n", list("future.plan"), "\n", list("future.onFutureCondition.keepFuture"),
#> "\n", list("future.resolve.recursive"), "\n",
#> list("future.connections.onMisuse"), "\n", list(
#> "future.defaultDevice.onMisuse"), "\n", list(
#> "future.devices.onMisuse"), "\n", list("future.globalenv.onMisuse"),
#> "\n", list("future.rng.onMisuse"), "\n", list(
#> "future.wait.alpha"), "\n", list("future.wait.interval"),
#> "\n", list("future.wait.timeout"), "\n", list(
#> "future.output.windows.reencode"), "\n", list(
#> "future.journal"), "\n", list("future.globals.objectSize.method"),
#> "\n", list("future.ClusterFuture.clusterEvalQ"),
#> "\n", list("R_FUTURE_STARTUP_SCRIPT"), "\n",
#> list("R_FUTURE_DEBUG"), "\n", list("R_FUTURE_DEMO_MANDELBROT_REGION"),
#> "\n", list("R_FUTURE_DEMO_MANDELBROT_NROW"),
#> "\n", list("R_FUTURE_FORK_MULTITHREADING_ENABLE"),
#> "\n", list("R_FUTURE_GLOBALS_MAXSIZE"), "\n",
#> list("R_FUTURE_GLOBALS_METHOD"), "\n", list("R_FUTURE_GLOBALS_ONMISSING"),
#> "\n", list("R_FUTURE_GLOBALS_RESOLVE"), "\n",
#> list("R_FUTURE_GLOBALS_ONREFERENCE"), "\n", list(
#> "R_FUTURE_PLAN"), "\n", list("R_FUTURE_ONFUTURECONDITION_KEEPFUTURE"),
#> "\n", list("R_FUTURE_RESOLVE_RECURSIVE"), "\n",
#> list("R_FUTURE_CONNECTIONS_ONMISUSE"), "\n",
#> list("R_FUTURE_DEVICES_ONMISUSE"), "\n", list(
#> "R_FUTURE_DEFAULTDEVICE_ONMISUSE"), "\n", list(
#> "R_FUTURE_GLOBALENV_ONMISUSE"), "\n", list(
#> "R_FUTURE_RNG_ONMISUSE"), "\n", list("R_FUTURE_WAIT_ALPHA"),
#> "\n", list("R_FUTURE_WAIT_INTERVAL"), "\n", list(
#> "R_FUTURE_WAIT_TIMEOUT"), "\n", list("R_FUTURE_RESOLVED_TIMEOUT"),
#> "\n", list("R_FUTURE_OUTPUT_WINDOWS_REENCODE"),
#> "\n", list("R_FUTURE_JOURNAL"), "\n", list("R_FUTURE_GLOBALS_OBJECTSIZE_METHOD"),
#> "\n", list("R_FUTURE_CLUSTERFUTURE_CLUSTEREVALQ"),
#> "\n", list("future.cmdargs"), "\n", list(".future.R"),
#> "\n", list("Options used for futures"), "\n",
#> list("\n", "Below are the ", list(), " options and environment variables that are used by the\n",
#> list("future"), " package and packages enhancing it.",
#> list(), "\n", list(), "\n", list("WARNING: Note that the names and the default values of these options may\n",
#> "change in future versions of the package. Please use with care until\n",
#> "further notice."), "\n"), "\n", list(list(
#> "Packages must not change future options"),
#> list("\n", "\n", "\n", "Just like for other R options, as a package developer you must ",
#> list("not"), " change\n", "any of the below ",
#> list("future.*"), " options. Only the end-user should set these.\n",
#> "If you find yourself having to tweak one of the options, make sure to\n",
#> "undo your changes immediately afterward. For example, if you want to\n",
#> "bump up the ", list("future.globals.maxSize"),
#> " limit when creating a future,\n", "use something like the following inside your function:\n",
#> "\n", list(list("html"), list(list("<div class=\"sourceCode r\">"))),
#> list("oopts <- options(future.globals.maxSize = 1.0 * 1e9) ## 1.0 GB\n",
#> "on.exit(options(oopts))\n", "f <- future({ expr }) ## Launch a future with large objects\n"),
#> list(list("html"), list(list("</div>"))),
#> "\n")), "\n", "\n", list(list("Options for controlling futures"),
#> list("\n", "\n", list("\n", list(list(list(
#> "future.plan"), ":"), list("(character string or future function) Default future backend used unless otherwise specified via ",
#> list(list("plan()")), ". This will also be the future plan set when calling ",
#> list("plan(\"default\")"), ". If not specified, this option may be set when the ",
#> list("future"), " package is ", list("loaded"),
#> " if command-line option ", list("--parallel=ncores"),
#> " (short ", list("-p ncores"), ") is specified; if ",
#> list("ncores > 1"), ", then option ", list(
#> "future.plan"), " is set to ", list("multisession"),
#> " otherwise ", list("sequential"), " (in addition to option ",
#> list("mc.cores"), " being set to ", list(
#> "ncores"), ", if ", list("ncores >= 1"),
#> "). (Default: ", list("sequential"), ")")),
#> "\n", "\n", list(list(list("future.globals.maxSize"),
#> ":"), list("(numeric) Maximum allowed total size (in bytes) of global variables identified. This is used to protect against exporting too large objects to parallel workers by mistake. Transferring large objects over a network, or over the internet, can be slow and therefore introduce a large bottleneck that increases the overall processing time. It can also result in large egress or ingress costs, which may exist on some systems. If set of ",
#> list("+Inf"), ", then the check for large globals is skipped. (Default: ",
#> list("500 * 1024 ^ 2"), " = 500 MiB)")),
#> "\n", "\n", list(list(list("future.globals.onReference"),
#> ": (", list("beta feature - may change"),
#> ")"), list("(character string) Controls whether the identified globals should be scanned for so called ",
#> list("references"), " (e.g. external pointers and connections) or not. It is unlikely that another ",
#> list(), " process (\"worker\") can use a global that uses a internal reference of the master ",
#> list(), " process---we call such objects ",
#> list("non-exportable globals"), ".\n",
#> "If this option is ", list("\"error\""),
#> ", an informative error message is produced if a non-exportable global is detected.\n",
#> "If ", list("\"warning\""), ", a warning is produced, but the processing will continue; it is likely that the future will be resolved with a run-time error unless processed in the master ",
#> list(), " process (e.g. ", list("plan(sequential)"),
#> " and ", list("plan(multicore)"), ").\n",
#> "If ", list("\"ignore\""), ", no scan is performed.\n",
#> "(Default: ", list("\"ignore\""), " but may change)\n")),
#> "\n", "\n", list(list(list("future.resolve.recursive"),
#> ":"), list("(integer) An integer specifying the maximum recursive depth to which futures should be resolved. If negative, nothing is resolved. If ",
#> list("0"), ", only the future itself is resolved. If ",
#> list("1"), ", the future and any of its elements that are futures are resolved, and so on. If ",
#> list("+Inf"), ", infinite search depth is used. (Default: ",
#> list("0"), ")")), "\n", "\n", list(list(
#> list("future.onFutureCondition.keepFuture"),
#> ":"), list("(logical) If ", list("TRUE"),
#> ", a ", list("FutureCondition"), " keeps a copy of the ",
#> list("Future"), " object that triggered the condition. If ",
#> list("FALSE"), ", it is dropped. (Default: ",
#> list("TRUE"), ")")), "\n", "\n", list(list(
#> list("future.wait.timeout"), ":"), list(
#> "(numeric) Maximum waiting time (in seconds) for a future to resolve or for a free worker to become available before a timeout error is generated. (Default: ",
#> list("30 * 24 * 60 * 60"), " (= 30 days))")),
#> "\n", "\n", list(list(list("future.wait.interval"),
#> ":"), list("(numeric) Initial interval (in\n",
#> "seconds) between polls. This controls the polling frequency for finding\n",
#> "an available worker when all workers are currently busy. It also controls\n",
#> "the polling frequency of ", list("resolve()"),
#> ". (Default: ", list("0.01"), " = 1 ms)")),
#> "\n", "\n", list(list(list("future.wait.alpha"),
#> ":"), list("(numeric) Positive scale factor used to increase the interval after each poll. (Default: ",
#> list("1.01"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Options for built-in sanity checks"),
#> list("\n", "\n", "\n", "Ideally, the evaluation of a future should have no side effects. To\n",
#> "protect against unexpected side effects, the future framework comes\n",
#> "with a set of built-in tools for checking against this.\n",
#> "Below R options control these built-in checks and what should happen\n",
#> "if they fail. You may modify them for troubleshooting purposes, but\n",
#> "please refrain from disabling these checks when there is an underlying\n",
#> "problem that should be fixed.\n", "\n",
#> list("Beta features: Please consider these checks to be \"under construction\"."),
#> "\n", "\n", list("\n", list(list(list("future.connections.onMisuse"),
#> ":"), list("(character string)\n", "A future must close any connections it opens and must not close\n",
#> "connections it did not open itself.\n",
#> "If such misuse is detected and this option is set to ",
#> list("\"error\""), ",\n", "then an informative error is produced. If it is set to ",
#> list("\"warning\""), ",\n", "a warning is produced. If",
#> list("\"ignore\""), ", no check is performed.\n",
#> "(Default: ", list("\"warning\""), ")\n")),
#> "\n", "\n", list(list(list("future.defaultDevice.onMisuse"),
#> ":"), list("(character string)\n", "A future must open graphics devices explicitly, if it creates new\n",
#> "plots. It should not rely on the default graphics device that\n",
#> "is given by R option ", list("\"default\""),
#> ", because that rarely does what\n",
#> "is intended.\n", "If such misuse is detected and this option is set to ",
#> list("\"error\""), ",\n", "then an informative error is produced. If it is set to ",
#> list("\"warning\""), ",\n", "a warning is produced. If",
#> list("\"ignore\""), ", no check is performed.\n",
#> "(Default: ", list("\"warning\""), ")\n")),
#> "\n", "\n", list(list(list("future.devices.onMisuse"),
#> ":"), list("(character string)\n", "A future must close any graphics devices it opens and must not close\n",
#> "devices it did not open itself.\n",
#> "If such misuse is detected and this option is set to ",
#> list("\"error\""), ",\n", "then an informative error is produced. If it is set to ",
#> list("\"warning\""), ",\n", "a warning is produced. If",
#> list("\"ignore\""), ", no check is performed.\n",
#> "(Default: ", list("\"warning\""), ")\n")),
#> "\n", "\n", list(list(list("future.globalenv.onMisuse"),
#> ":"), list("(character string)\n", "Assigning variables to the global environment for the purpose of using\n",
#> "the variable at a later time makes no sense with futures, because the\n",
#> "next the future may be evaluated in different R process.\n",
#> "To protect against mistakes, the future framework attempts to detect\n",
#> "when variables are added to the global environment.\n",
#> "If this is detected, and this option is set to ",
#> list("\"error\""), ", then an\n", "informative error is produced. If ",
#> list("\"warning\""), ", then a warning is\n",
#> "produced. If ", list("\"ignore\""),
#> ", no check is performed.\n", "(Default: ",
#> list("\"ignore\""), ")\n")), "\n", "\n",
#> list(list(list("future.rng.onMisuse"),
#> ":"), list("(character string)\n", "If random numbers are used in futures, then parallel RNG should be\n",
#> list("declared"), " in order to get statistical sound RNGs. You can declare\n",
#> "this by specifying future argument ",
#> list("seed = TRUE"), ". The defaults in the\n",
#> "future framework assume that ", list(
#> "no"), " random number generation (RNG) is\n",
#> "taken place in the future expression because L'Ecuyer-CMRG RNGs come\n",
#> "with an unnecessary overhead if not needed.\n",
#> "To protect against mistakes of not declaring use of the RNG, the\n",
#> "future framework detects when random numbers were used despite not\n",
#> "declaring such use.\n", "If this is detected, and this options is set ",
#> list("\"error\""), ", then an\n", "informative error is produced. If ",
#> list("\"warning\""), ", then a warning is\n",
#> "produced. If ", list("\"ignore\""),
#> ", no check is performed.\n", "(Default: ",
#> list("\"warning\""), ")\n")), "\n"),
#> "\n")), "\n", "\n", list(list("Options for debugging futures"),
#> list("\n", "\n", list("\n", list(list(list(
#> "future.debug"), ":"), list("(logical) If ",
#> list("TRUE"), ", extensive debug messages are generated. (Default: ",
#> list("FALSE"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Options for controlling package startup"),
#> list("\n", "\n", list("\n", list(list(list(
#> "future.startup.script"), ":"), list("(character vector or a logical) Specifies zero of more future startup scripts to be sourced when the ",
#> list("future"), " package is ", list("attached"),
#> ". It is only the first existing script that is sourced. If none of the specified files exist, nothing is sourced---there will be neither a warning nor an error.\n",
#> "If this option is not specified, environment variable ",
#> list("R_FUTURE_STARTUP_SCRIPT"), " is considered, where multiple scripts may be separated by either a colon (",
#> list(":"), ") or a semicolon (", list(";"),
#> "). If neither is set, or either is set to ",
#> list("TRUE"), ", the default is to look for a ",
#> list(".future.R"), " script in the current directory and then in the user's home directory. To disable future startup scripts, set the option or the environment variable to ",
#> list("FALSE"), ". ", list("Importantly"),
#> ", this option is ", list("always"), " set to ",
#> list("FALSE"), " if the ", list("future"),
#> " package is loaded as part of a future expression being evaluated, e.g. in a background process. In order words, they are sourced in the main ",
#> list(), " process but not in future processes. (Default: ",
#> list("TRUE"), " in main ", list(), " process and ",
#> list("FALSE"), " in future processes / during future evaluation)")),
#> "\n", "\n", list(list(list("future.cmdargs"),
#> ":"), list("(character vector) Overrides ",
#> list(list("commandArgs"), "()"), " when the ",
#> list("future"), " package is ", list("loaded"),
#> ".")), "\n"), "\n")), "\n", "\n", list(
#> list("Options for configuring low-level system behaviors"),
#> list("\n", "\n", "\n", list("\n", list(list(
#> list("future.fork.multithreading.enable"),
#> " (", list("beta feature - may change"),
#> "):"), list("(logical) Enable or disable ",
#> list("multi-threading"), " while using ",
#> list("forked"), " parallel processing. If ",
#> list("FALSE"), ", different multi-thread library settings are overridden such that they run in single-thread mode. Specifically, multi-threading will be disabled for OpenMP (which requires the ",
#> list("RhpcBLASctl"), " package) and for ",
#> list("RcppParallel"), ". If ", list("TRUE"),
#> ", or not set (the default), multi-threading is allowed. Parallelization via multi-threaded processing (done in native code by some packages and external libraries) while at the same time using forked (aka \"multicore\") parallel processing is known to unstable. Note that this is not only true when using ",
#> list("plan(multicore)"), " but also when using, for instance, ",
#> list(list("mclapply"), "()"), " of the ",
#> list("parallel"), " package. (Default: not set)")),
#> "\n", "\n", list(list(list("future.output.windows.reencode"),
#> ":"), list("(logical) Enable or disable re-encoding of UTF-8 symbols that were incorrectly encoded while captured. In R (< 4.2.0) and on older versions of MS Windows, R cannot capture UTF-8 symbols as-is when they are captured from the standard output. For examples, a UTF-8 check mark symbol (",
#> list("\"\\u2713\""), ") would be relayed as ",
#> list("\"<U+2713>\""), " (a string with eight ASCII characters). Setting this option to ",
#> list("TRUE"), " will cause ", list("value()"),
#> " to attempt to recover the intended UTF-8 symbols from ",
#> list("<U+nnnn>"), " string components, if, and only if, the string was captured by a future resolved on MS Windows. (Default: ",
#> list("TRUE"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Options for demos"), list("\n",
#> "\n", list("\n", list(list(list("future.demo.mandelbrot.region"),
#> ":"), list("(integer) Either a named list of ",
#> list(list("mandelbrot()")), " arguments or an integer in {1, 2, 3} specifying a predefined Mandelbrot region. (Default: ",
#> list("1L"), ")")), "\n", "\n", list(list(
#> list("future.demo.mandelbrot.nrow"), ":"),
#> list("(integer) Number of rows and columns of tiles. (Default: ",
#> list("3L"), ")")), "\n"), "\n")), "\n",
#> "\n", list(list("Deprecated or for internal prototyping"),
#> list("\n", "\n", "\n", "The following options exists only for troubleshooting purposes and must not\n",
#> "be used in production. If used, there is a risk that the results are\n",
#> "non-reproducible if processed elsewhere. To lower the risk of them being\n",
#> "used by mistake, they are marked as deprecated and will produce warnings\n",
#> "if set.\n", "\n", list("\n", list(list(list(
#> "future.globals.onMissing"), ":"), list(
#> "(character string) Action to take when non-existing global variables (\"globals\" or \"unknowns\") are identified when the future is created. If ",
#> list("\"error\""), ", an error is generated immediately. If ",
#> list("\"ignore\""), ", no action is taken and an attempt to evaluate the future expression will be made. The latter is useful when there is a risk for false-positive globals being identified, e.g. when future expression contains non-standard evaluation (NSE). (Default: ",
#> list("\"ignore\""), ")")), "\n", "\n",
#> list(list(list("future.globals.method"),
#> ":"), list("(character string) Method used to identify globals. For details, see ",
#> list(list("globalsOf"), "()"), ". (Default: ",
#> list("\"ordered\""), ")")), "\n", "\n",
#> list(list(list("future.globals.resolve"),
#> ":"), list("(logical) If ", list("TRUE"),
#> ", globals that are ", list(list("Future")),
#> " objects (typically created as ", list(
#> "explicit"), " futures) will be resolved and have their values (using ",
#> list("value()"), ") collected. Because searching for unresolved futures among globals (including their content) can be expensive, the default is not to do it and instead leave it to the run-time checks that assert proper ownership when resolving futures and collecting their values. (Default: ",
#> list("FALSE"), ")")), "\n"), "\n")),
#> "\n", "\n", list(list("Environment variables that set R options"),
#> list("\n", "\n", "All of the above ", list(),
#> " ", list("future.*"), " options can be set by corresponding\n",
#> "environment variable ", list("R_FUTURE_*"),
#> " ", list("when the ", list("future"), " package is\n",
#> "loaded"), ". This means that those environment variables must be set before\n",
#> "the ", list("future"), " package is loaded in order to have an effect.\n",
#> "For example, if ", list("R_FUTURE_RNG_ONMISUSE=\"ignore\""),
#> ", then option\n", list("future.rng.onMisuse"),
#> " is set to ", list("\"ignore\""), " (character string).\n",
#> "Similarly, if ", list("R_FUTURE_GLOBALS_MAXSIZE=\"50000000\""),
#> ", then option\n", list("future.globals.maxSize"),
#> " is set to ", list("50000000"), " (numeric).\n")),
#> "\n", "\n", list(list("Options moved to the 'parallelly' package"),
#> list("\n", "\n", "Several functions have been moved to the ",
#> list("parallelly"), " package:\n", list("\n",
#> list(), " ", list(list("parallelly::availableCores()")),
#> "\n", list(), " ", list(list("parallelly::availableWorkers()")),
#> "\n", list(), " ", list(list("parallelly::makeClusterMPI()")),
#> "\n", list(), " ", list(list("parallelly::makeClusterPSOCK()")),
#> "\n", list(), " ", list(list("parallelly::makeNodePSOCK()")),
#> "\n", list(), " ", list(list("parallelly::supportsMulticore()")),
#> "\n"), "\n", "\n", "The options and environment variables controlling those have been adjusted\n",
#> "accordingly to have different prefixes.\n",
#> "For example, option ", list("future.fork.enable"),
#> " has been renamed to\n", list("parallelly.fork.enable"),
#> " and the corresponding environment variable\n",
#> list("R_FUTURE_FORK_ENABLE"), " has been renamed to\n",
#> list("R_PARALLELLY_FORK_ENABLE"), ".\n",
#> "For backward compatibility reasons, the ",
#> list("parallelly"), " package will\n", "support both versions for a long foreseeable time.\n",
#> "See the ", list("parallelly::parallelly.options"),
#> " page for the settings.\n")), "\n", "\n",
#> list("\n", "# Allow at most 5 MB globals per futures\n",
#> "options(future.globals.maxSize = 5e6)\n",
#> "\n", "# Be strict; catch all RNG mistakes\n",
#> "options(future.rng.onMisuse = \"error\")\n",
#> "\n", "\n"), "\n", list("\n", "To set ", list(),
#> " options or environment variables when ",
#> list(), " starts (even before the ", list("future"),
#> " package is loaded), see the ", list("Startup"),
#> " help page. The ", list(list("https://cran.r-project.org/package=startup"),
#> list(list("startup"))), " package provides a friendly mechanism for configurating ",
#> list(), "'s startup process.\n"), "\n")), source = list(
#> `Future-class.Rd` = "R/backend_api-Future-class.R",
#> FutureBackend.Rd = c("R/backend_api-01-FutureBackend-class.R",
#> "R/backend_api-03.MultiprocessFutureBackend-class.R",
#> "R/backend_api-11.ClusterFutureBackend-class.R",
#> "R/backend_api-11.MulticoreFutureBackend-class.R",
#> "R/backend_api-11.SequentialFutureBackend-class.R",
#> "R/backend_api-13.MultisessionFutureBackend-class.R"
#> ), FutureCondition.Rd = c("R/protected_api-FutureCondition-class.R",
#> "R/protected_api-journal.R"), FutureGlobals.Rd = "R/protected_api-FutureGlobals-class.R",
#> FutureResult.Rd = "R/protected_api-FutureResult-class.R",
#> `MulticoreFuture-class.Rd` = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> `MultiprocessFuture-class.Rd` = "R/backend_api-03.MultiprocessFutureBackend-class.R",
#> `UniprocessFuture-class.Rd` = "R/backend_api-UniprocessFuture-class.R",
#> backtrace.Rd = "R/utils_api-backtrace.R", cancel.Rd = "R/core_api-cancel.R",
#> cluster.Rd = "R/backend_api-11.ClusterFutureBackend-class.R",
#> clusterExportSticky.Rd = "R/utils-sticky_globals.R",
#> find_references.Rd = "R/utils-marshalling.R", future.Rd = c("R/core_api-future.R",
#> "R/utils_api-futureCall.R", "R/utils_api-minifuture.R"
#> ), futureAssign.Rd = c("R/delayed_api-futureAssign.R",
#> "R/infix_api-01-futureAssign_OP.R", "R/infix_api-02-globals_OP.R",
#> "R/infix_api-03-seed_OP.R", "R/infix_api-04-stdout_OP.R",
#> "R/infix_api-05-conditions_OP.R", "R/infix_api-06-lazy_OP.R",
#> "R/infix_api-07-label_OP.R", "R/infix_api-08-plan_OP.R",
#> "R/infix_api-09-tweak_OP.R"), futureOf.Rd = "R/delayed_api-futureOf.R",
#> futureSessionInfo.Rd = "R/utils_api-futureSessionInfo.R",
#> futures.Rd = "R/protected_api-futures.R", getExpression.Rd = "R/backend_api-Future-class.R",
#> getGlobalsAndPackages.Rd = "R/protected_api-globals.R",
#> makeClusterFuture.Rd = "R/utils_api-makeClusterFuture.R",
#> mandelbrot.Rd = "R/demo_api-mandelbrot.R", multicore.Rd = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> multisession.Rd = "R/backend_api-13.MultisessionFutureBackend-class.R",
#> nbrOfWorkers.Rd = "R/utils_api-nbrOfWorkers.R", nullcon.Rd = "R/utils-basic.R",
#> plan.Rd = c("R/utils_api-plan-with.R", "R/utils_api-plan.R",
#> "R/utils_api-tweak.R"), private_length.Rd = "R/utils-basic.R",
#> `re-exports.Rd` = "R/000.re-exports.R", readImmediateConditions.Rd = "R/utils-immediateCondition.R",
#> requestCore.Rd = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> reset.Rd = "R/core_api-reset.R", resetWorkers.Rd = "R/utils_api-plan.R",
#> resolve.Rd = "R/protected_api-resolve.R", resolved.Rd = "R/core_api-resolved.R",
#> result.Rd = "R/backend_api-Future-class.R", run.Rd = "R/backend_api-Future-class.R",
#> save_rds.Rd = "R/utils-immediateCondition.R", sequential.Rd = "R/backend_api-11.SequentialFutureBackend-class.R",
#> sessionDetails.Rd = "R/utils_api-sessionDetails.R",
#> signalConditions.Rd = "R/protected_api-signalConditions.R",
#> sticky_globals.Rd = "R/utils-sticky_globals.R", usedCores.Rd = "R/backend_api-11.MulticoreFutureBackend-class.R",
#> value.Rd = "R/core_api-value.R", `zzz-future.options.Rd` = "R/utils-options.R"),
#> keywords = list("internal", "internal", "internal", "internal",
#> "internal", "internal", "internal", "internal", character(0),
#> character(0), character(0), "internal", "internal",
#> character(0), character(0), character(0), character(0),
#> character(0), "internal", "internal", "internal",
#> "internal", character(0), character(0), character(0),
#> "internal", character(0), "internal", "internal",
#> "internal", "internal", character(0), "internal",
#> character(0), character(0), "internal", "internal",
#> "internal", character(0), "internal", "internal",
#> "internal", "internal", character(0), character(0)),
#> concepts = list(character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0), character(0), character(0),
#> character(0), character(0)), internal = c(TRUE, TRUE,
#> TRUE, TRUE, TRUE, TRUE, TRUE, TRUE, FALSE, FALSE, FALSE,
#> TRUE, TRUE, FALSE, FALSE, FALSE, FALSE, FALSE, TRUE,
#> TRUE, TRUE, TRUE, FALSE, FALSE, FALSE, TRUE, FALSE, TRUE,
#> TRUE, TRUE, TRUE, FALSE, TRUE, FALSE, FALSE, TRUE, TRUE,
#> TRUE, FALSE, TRUE, TRUE, TRUE, TRUE, FALSE, FALSE), lifecycle = list(
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL,
#> NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL, NULL)),
#> tutorials = list(name = character(0), file_out = character(0),
#> title = character(0), pagetitle = character(0), url = character(0)),
#> vignettes = list(name = c("future-1-overview", "future-2-output",
#> "future-2b-backend", "future-3-topologies", "future-4-issues",
#> "future-4-non-exportable-objects", "future-5-startup", "future-6-future-api-backend-specification",
#> "future-7-for-package-developers", "future-8-how-future-is-validated"
#> ), type = c("rmd", "rmd", "rmd", "rmd", "rmd", "rmd", "rmd",
#> "rmd", "rmd", "rmd"), file_in = c("vignettes/future-1-overview.Rmd",
#> "vignettes/future-2-output.Rmd", "vignettes/future-2b-backend.Rmd",
#> "vignettes/future-3-topologies.Rmd", "vignettes/future-4-issues.Rmd",
#> "vignettes/future-4-non-exportable-objects.Rmd", "vignettes/future-5-startup.Rmd",
#> "vignettes/future-6-future-api-backend-specification.Rmd",
#> "vignettes/future-7-for-package-developers.Rmd", "vignettes/future-8-how-future-is-validated.Rmd"
#> ), file_out = c("articles/future-1-overview.html", "articles/future-2-output.html",
#> "articles/future-2b-backend.html", "articles/future-3-topologies.html",
#> "articles/future-4-issues.html", "articles/future-4-non-exportable-objects.html",
#> "articles/future-5-startup.html", "articles/future-6-future-api-backend-specification.html",
#> "articles/future-7-for-package-developers.html", "articles/future-8-how-future-is-validated.html"
#> ), title = c("A Future for R: A Comprehensive Overview",
#> "A Future for R: Text and Message Output", "A Future for R: Available Future Backends",
#> "A Future for R: Future Topologies", "A Future for R: Common Issues with Solutions",
#> "A Future for R: Non-Exportable Objects", "A Future for R: Controlling Default Future Strategy",
#> "A Future for R: Future API Backend Specification", "A Future for R: Best Practices for Package Developers",
#> "A Future for R: How the Future Framework is Validated"),
#> description = c(NA_character_, NA_character_, NA_character_,
#> NA_character_, NA_character_, NA_character_, NA_character_,
#> NA_character_, NA_character_, NA_character_), depth = c(1L,
#> 1L, 1L, 1L, 1L, 1L, 1L, 1L, 1L, 1L)))), examples = base::quote(TRUE),
#> run_dont_run = base::quote(FALSE), seed = base::quote(1014L),
#> lazy = base::quote(FALSE), override = base::quote(list()),
#> install = base::quote(FALSE), preview = base::quote(FALSE),
#> new_process = base::quote(FALSE), devel = base::quote(FALSE),
#> cli_colors = base::quote(16777216L), hyperlinks = base::quote(TRUE))
#>
#> [[13]]
#> pkgdown::build_site(...)
#>
#> [[14]]
#> build_site_local(pkg = pkg, examples = examples, run_dont_run = run_dont_run,
#> seed = seed, lazy = lazy, override = override, preview = preview,
#> devel = devel)
#>
#> [[15]]
#> build_reference(pkg, lazy = lazy, examples = examples, run_dont_run = run_dont_run,
#> seed = seed, override = override, preview = FALSE, devel = devel)
#>
#> [[16]]
#> unwrap_purrr_error(purrr::map(topics, build_reference_topic,
#> pkg = pkg, lazy = lazy, examples_env = examples_env, run_dont_run = run_dont_run))
#>
#> [[17]]
#> withCallingHandlers(code, purrr_error_indexed = function(err) {
#> cnd_signal(err$parent)
#> })
#>
#> [[18]]
#> purrr::map(topics, build_reference_topic, pkg = pkg, lazy = lazy,
#> examples_env = examples_env, run_dont_run = run_dont_run)
#>
#> [[19]]
#> map_("list", .x, .f, ..., .progress = .progress)
#>
#> [[20]]
#> with_indexed_errors(i = i, names = names, error_call = .purrr_error_call,
#> call_with_cleanup(map_impl, environment(), .type, .progress,
#> n, names, i))
#>
#> [[21]]
#> withCallingHandlers(expr, error = function(cnd) {
#> if (i == 0L) {
#> }
#> else {
#> message <- c(i = "In index: {i}.")
#> if (!is.null(names) && !is.na(names[[i]]) && names[[i]] !=
#> "") {
#> name <- names[[i]]
#> message <- c(message, i = "With name: {name}.")
#> }
#> else {
#> name <- NULL
#> }
#> cli::cli_abort(message, location = i, name = name, parent = cnd,
#> call = error_call, class = "purrr_error_indexed")
#> }
#> })
#>
#> [[22]]
#> call_with_cleanup(map_impl, environment(), .type, .progress,
#> n, names, i)
#>
#> [[23]]
#> .f(.x[[i]], ...)
#>
#> [[24]]
#> withCallingHandlers(data_reference_topic(topic, pkg, examples_env = examples_env,
#> run_dont_run = run_dont_run), error = function(err) {
#> cli::cli_abort("Failed to parse Rd in {.file {topic$file_in}}",
#> parent = err, call = quote(build_reference()))
#> })
#>
#> [[25]]
#> data_reference_topic(topic, pkg, examples_env = examples_env,
#> run_dont_run = run_dont_run)
#>
#> [[26]]
#> run_examples(tags$tag_examples[[1]], env = if (is.null(examples_env)) NULL else new.env(parent = examples_env),
#> topic = tools::file_path_sans_ext(topic$file_in), run_dont_run = run_dont_run)
#>
#> [[27]]
#> highlight_examples(code, topic, env = env)
#>
#> [[28]]
#> downlit::evaluate_and_highlight(code, fig_save = fig_save_topic,
#> env = eval_env, output_handler = handler)
#>
#> [[29]]
#> evaluate::evaluate(code, child_env(env), new_device = TRUE, output_handler = output_handler)
#>
#> [[30]]
#> withRestarts(with_handlers({
#> for (expr in tle$exprs) {
#> ev <- withVisible(eval(expr, envir))
#> watcher$capture_plot_and_output()
#> watcher$print_value(ev$value, ev$visible, envir)
#> }
#> TRUE
#> }, handlers), eval_continue = function() TRUE, eval_stop = function() FALSE)
#>
#> [[31]]
#> withRestartList(expr, restarts)
#>
#> [[32]]
#> withOneRestart(withRestartList(expr, restarts[-nr]), restarts[[nr]])
#>
#> [[33]]
#> doWithOneRestart(return(expr), restart)
#>
#> [[34]]
#> withRestartList(expr, restarts[-nr])
#>
#> [[35]]
#> withOneRestart(expr, restarts[[1L]])
#>
#> [[36]]
#> doWithOneRestart(return(expr), restart)
#>
#> [[37]]
#> with_handlers({
#> for (expr in tle$exprs) {
#> ev <- withVisible(eval(expr, envir))
#> watcher$capture_plot_and_output()
#> watcher$print_value(ev$value, ev$visible, envir)
#> }
#> TRUE
#> }, handlers)
#>
#> [[38]]
#> eval(call)
#>
#> [[39]]
#> eval(call)
#>
#> [[40]]
#> withCallingHandlers(code, message = function (cnd)
#> {
#> watcher$capture_plot_and_output()
#> if (on_message$capture) {
#> watcher$push(cnd)
#> }
#> if (on_message$silence) {
#> invokeRestart("muffleMessage")
#> }
#> }, warning = function (cnd)
#> {
#> if (getOption("warn") >= 2 || getOption("warn") < 0) {
#> return()
#> }
#> watcher$capture_plot_and_output()
#> if (on_warning$capture) {
#> cnd <- sanitize_call(cnd)
#> watcher$push(cnd)
#> }
#> if (on_warning$silence) {
#> invokeRestart("muffleWarning")
#> }
#> }, error = function (cnd)
#> {
#> watcher$capture_plot_and_output()
#> cnd <- sanitize_call(cnd)
#> watcher$push(cnd)
#> switch(on_error, continue = invokeRestart("eval_continue"),
#> stop = invokeRestart("eval_stop"), error = NULL)
#> })
#>
#> [[41]]
#> withVisible(eval(expr, envir))
#>
#> [[42]]
#> eval(expr, envir)
#>
#> [[43]]
#> eval(expr, envir)
#>
#> [[44]]
#> future({
#> foo("a")
#> })
#>
#> [[45]]
#> Future(expr, substitute = FALSE, envir = envir, lazy = TRUE,
#> seed = seed, globals = globals, packages = packages, stdout = stdout,
#> conditions = conditions, earlySignal = earlySignal, label = label,
#> gc = gc, onReference = onReference, ...)
#>
#> [[46]]
#> eval(expr, envir = globalenv())
#>
#> [[47]]
#> eval(expr, envir = globalenv())
#>
#> [[48]]
#> local({
#> {
#> foo("a")
#> }
#> })
#>
#> [[49]]
#> eval.parent(substitute(eval(quote(expr), envir)))
#>
#> [[50]]
#> eval(expr, p)
#>
#> [[51]]
#> eval(expr, p)
#>
#> [[52]]
#> eval(quote({
#> {
#> foo("a")
#> }
#> }), new.env())
#>
#> [[53]]
#> eval(quote({
#> {
#> foo("a")
#> }
#> }), new.env())
#>
#> [[54]]
#> foo("a")
#>
#> [[55]]
#> my_log(...)
#>
#> [[56]]
#> log(x)
#>