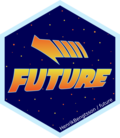
Create a multicore future whose value will be resolved asynchronously in a forked parallel process
Source:R/backend_api-11.MulticoreFutureBackend-class.R
multicore.Rd
WARNING: This function must never be called.
It may only be used with plan()
Usage
multicore(
...,
workers = availableCores(constraints = "multicore"),
gc = FALSE,
earlySignal = FALSE,
envir = parent.frame()
)
Arguments
- workers
The number of parallel processes to use. If a function, it is called without arguments when the future is created and its value is used to configure the workers.
- gc
If TRUE, the garbage collector run (in the process that evaluated the future) only after the value of the future is collected. Exactly when the values are collected may depend on various factors such as number of free workers and whether
earlySignal
is TRUE (more frequently) or FALSE (less frequently). Some types of futures ignore this argument.- earlySignal
Specified whether conditions should be signaled as soon as possible or not.
- envir
The environment from where global objects should be identified.
- ...
Additional named elements to
Future()
.
Value
A Future.
If workers == 1
, then all processing using done in the
current/main R session and we therefore fall back to using a
sequential future. To override this fallback, use workers = I(1)
.
This is also the case whenever multicore processing is not supported,
e.g. on Windows.
Details
A multicore future is a future that uses multicore evaluation, which means that its value is computed and resolved in parallel in another process.
This function is must not be called directly. Instead, the typical usages are:
Support for forked ("multicore") processing
Not all operating systems support process forking and thereby not multicore
futures. For instance, forking is not supported on Microsoft Windows.
Moreover, process forking may break some R environments such as RStudio.
Because of this, the future package disables process forking also in
such cases. See parallelly::supportsMulticore()
for details.
Trying to create multicore futures on non-supported systems or when
forking is disabled will result in multicore futures falling back to
becoming sequential futures. If used in RStudio, there will be an
informative warning:
> plan(multicore)
Warning message:
In supportsMulticoreAndRStudio(...) :
[ONE-TIME WARNING] Forked processing ('multicore') is not supported when
running R from RStudio because it is considered unstable. For more details,
how to control forked processing or not, and how to silence this warning in
future R sessions, see ?parallelly::supportsMulticore
See also
For processing in multiple background R sessions, see multisession futures.
Use parallelly::availableCores()
to see the total number of
cores that are available for the current R session.
Use availableCores("multicore") > 1L
to check
whether multicore futures are supported or not on the current
system.
Examples
## Use multicore futures
plan(multicore)
## A global variable
a <- 0
## Create future (explicitly)
f <- future({
b <- 3
c <- 2
a * b * c
})
## A multicore future is evaluated in a separate forked
## process. Changing the value of a global variable
## will not affect the result of the future.
a <- 7
print(a)
#> [1] 7
v <- value(f)
print(v)
#> [1] 0
stopifnot(v == 0)